Java Lecture 5 CS 1311X Our Story So Far The Story of O Inheritance,
82 Slides263.50 KB
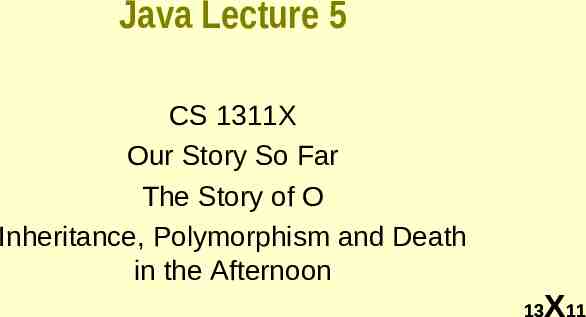
Java Lecture 5 CS 1311X Our Story So Far The Story of O Inheritance, Polymorphism and Death in the Afternoon X11 13
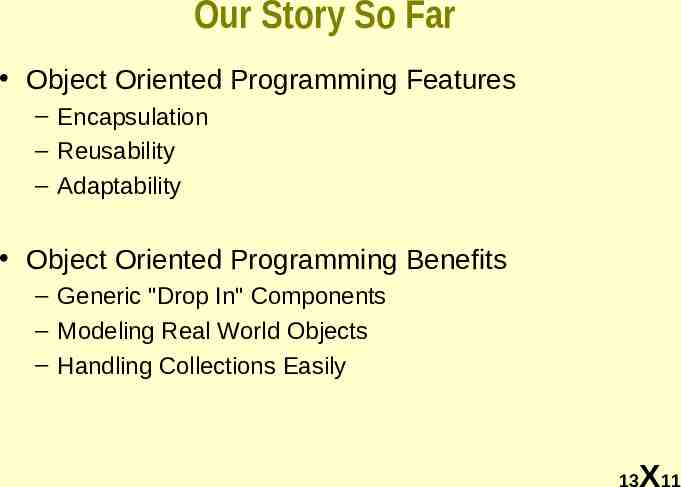
Our Story So Far Object Oriented Programming Features – Encapsulation – Reusability – Adaptability Object Oriented Programming Benefits – Generic "Drop In" Components – Modeling Real World Objects – Handling Collections Easily X11 13
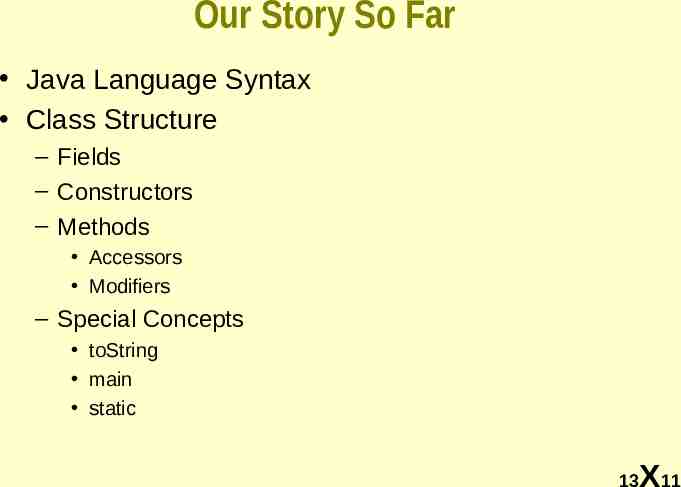
Our Story So Far Java Language Syntax Class Structure – Fields – Constructors – Methods Accessors Modifiers – Special Concepts toString main static X11 13
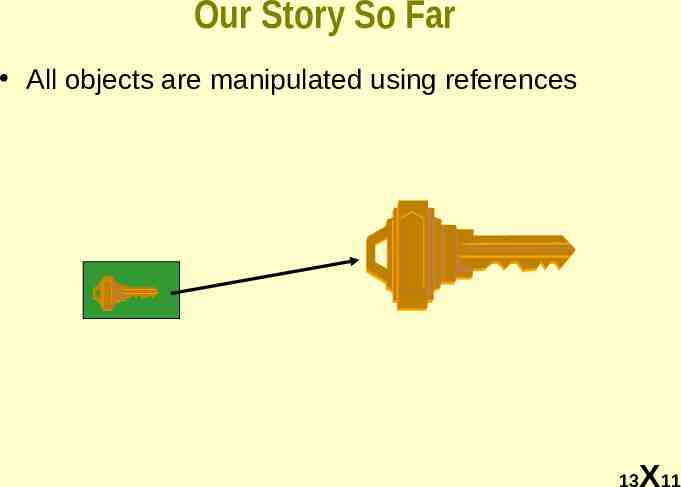
Our Story So Far All objects are manipulated using references X11 13
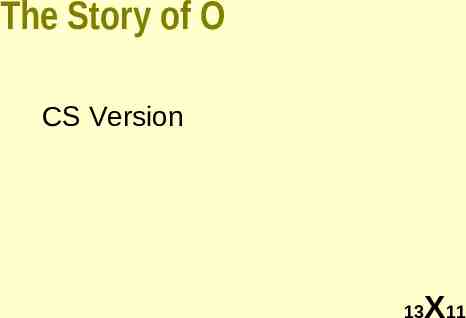
The Story of O CS Version X11 13
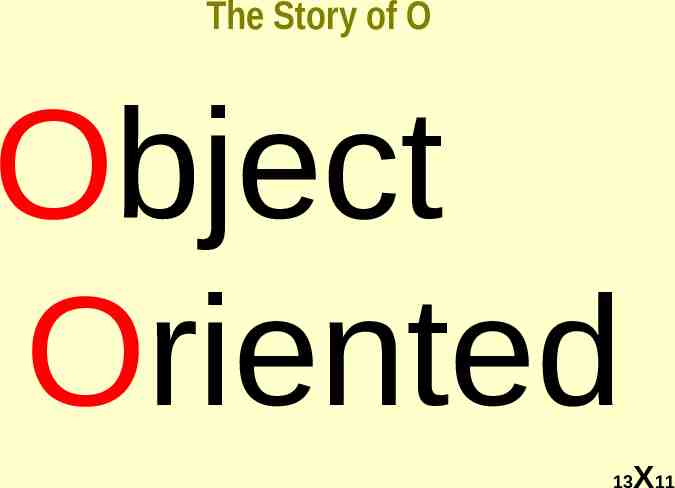
The Story of O Object Oriented X11 13
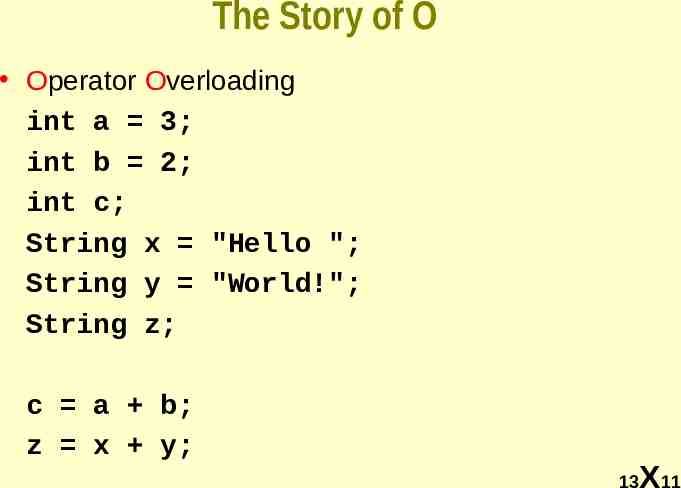
The Story of O Operator Overloading int a 3; int b 2; int c; String x "Hello "; String y "World!"; String z; c a b; z x y; X11 13
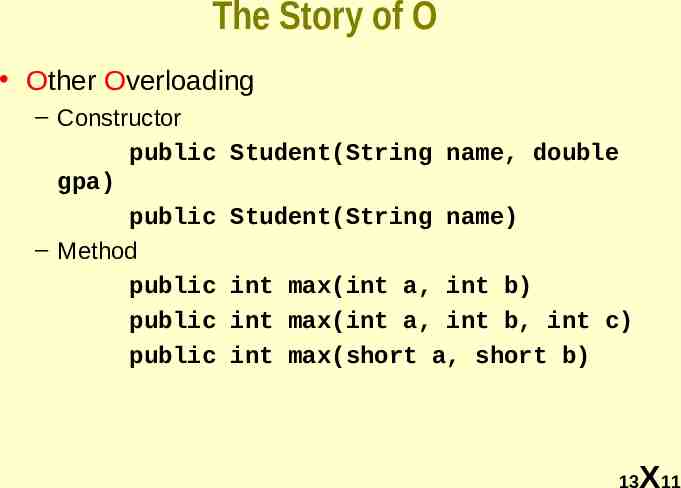
The Story of O Other Overloading – Constructor public gpa) public – Method public public public Student(String name, double Student(String name) int max(int a, int b) int max(int a, int b, int c) int max(short a, short b) X11 13
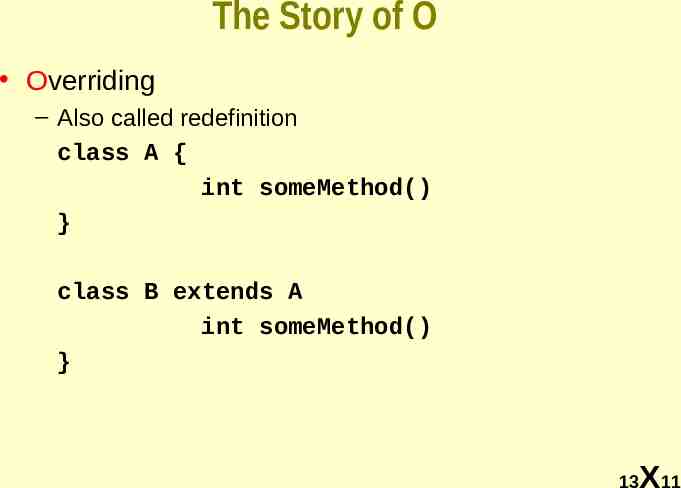
The Story of O Overriding – Also called redefinition class A { int someMethod() } class B extends A int someMethod() } X11 13
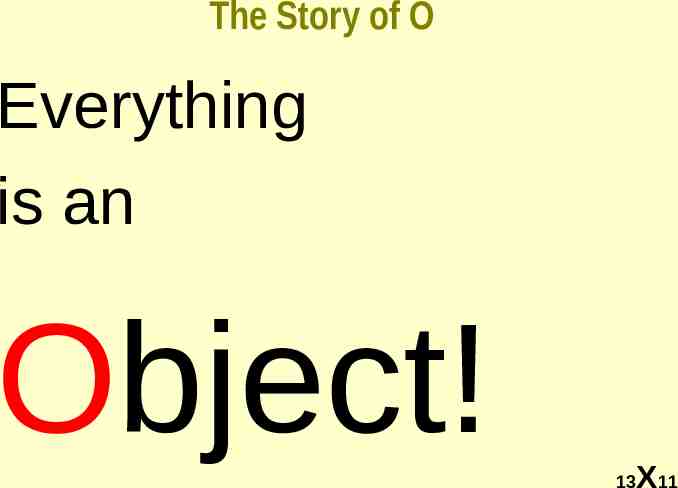
The Story of O Everything is an Object! X11 13
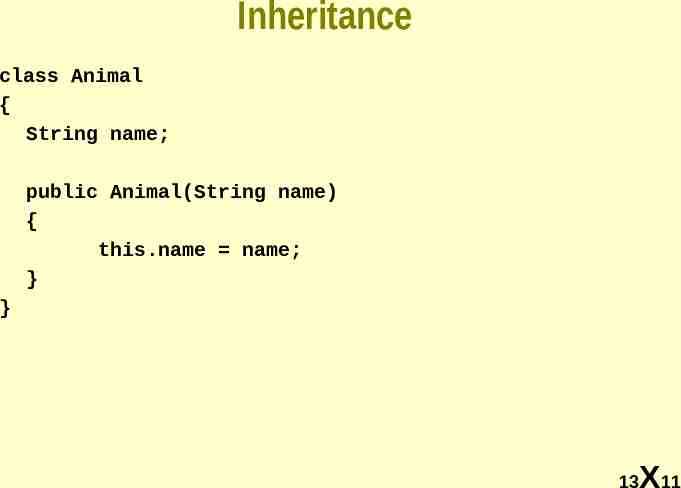
Inheritance class Animal { String name; public Animal(String name) { this.name name; } } X11 13
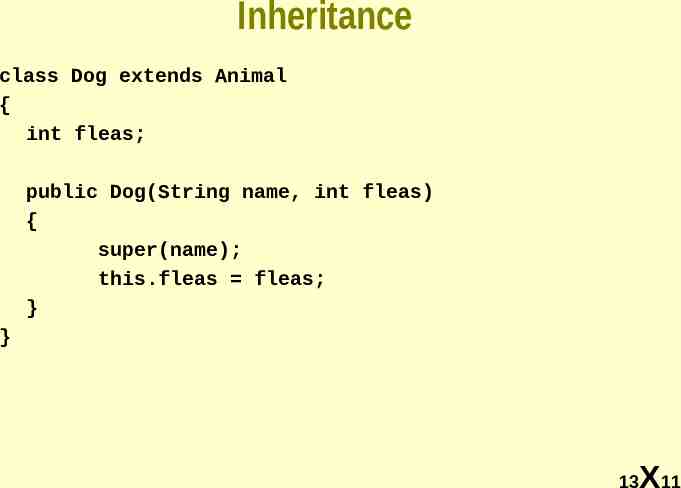
Inheritance class Dog extends Animal { int fleas; public Dog(String name, int fleas) { super(name); this.fleas fleas; } } X11 13
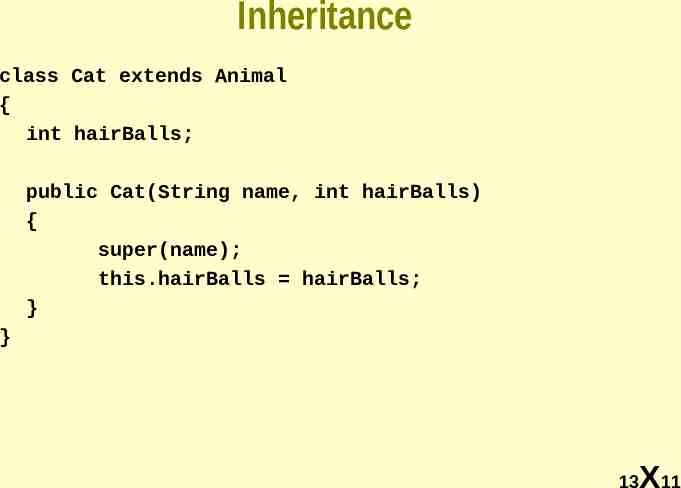
Inheritance class Cat extends Animal { int hairBalls; public Cat(String name, int hairBalls) { super(name); this.hairBalls hairBalls; } } X11 13
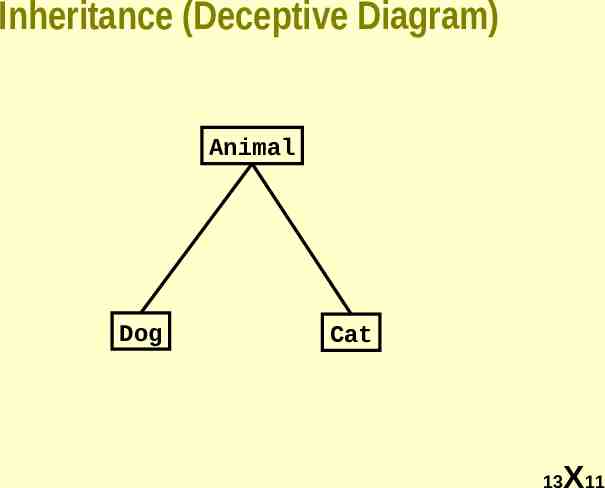
Inheritance (Deceptive Diagram) Animal Dog Cat X11 13
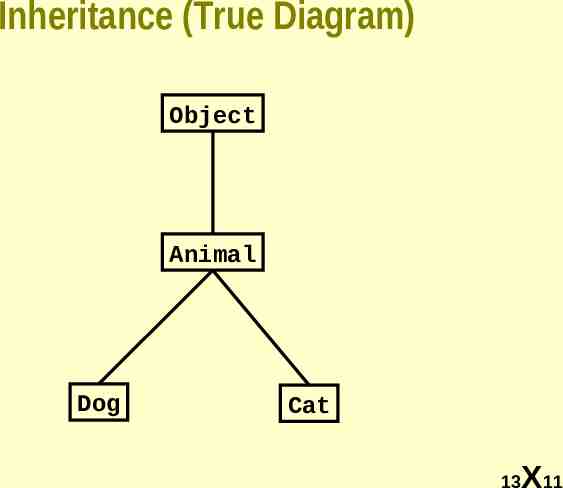
Inheritance (True Diagram) Object Animal Dog Cat X11 13
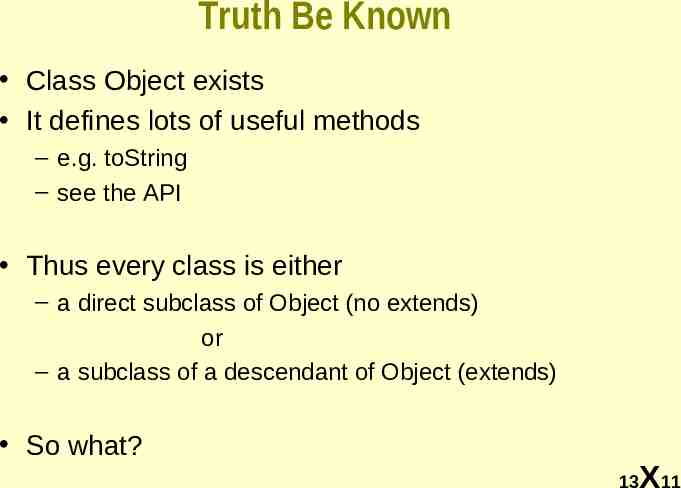
Truth Be Known Class Object exists It defines lots of useful methods – e.g. toString – see the API Thus every class is either – a direct subclass of Object (no extends) or – a subclass of a descendant of Object (extends) So what? X11 13
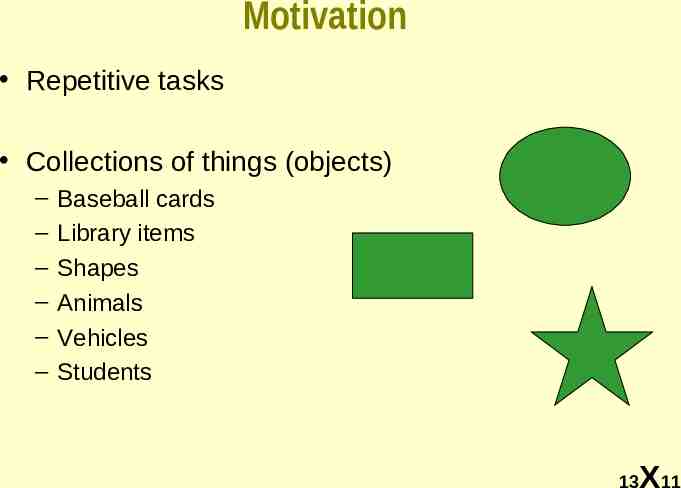
Motivation Repetitive tasks Collections of things (objects) – – – – – – Baseball cards Library items Shapes Animals Vehicles Students X11 13
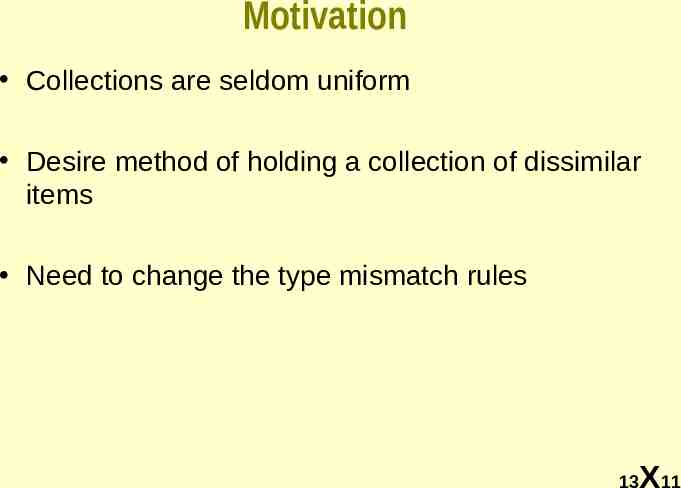
Motivation Collections are seldom uniform Desire method of holding a collection of dissimilar items Need to change the type mismatch rules X11 13
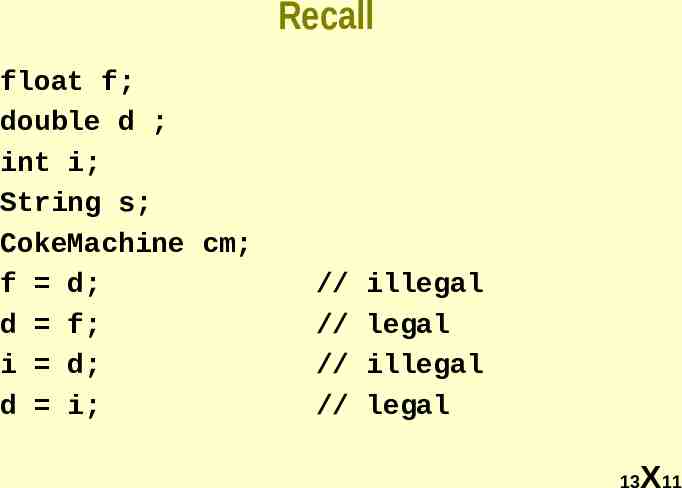
Recall float f; double d ; int i; String s; CokeMachine cm; f d; d f; i d; d i; // // // // illegal legal illegal legal X11 13
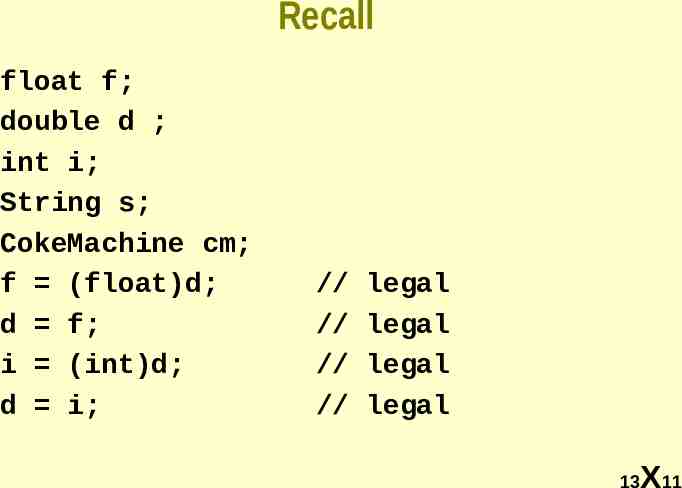
Recall float f; double d ; int i; String s; CokeMachine cm; f (float)d; d f; i (int)d; d i; // // // // legal legal legal legal X11 13
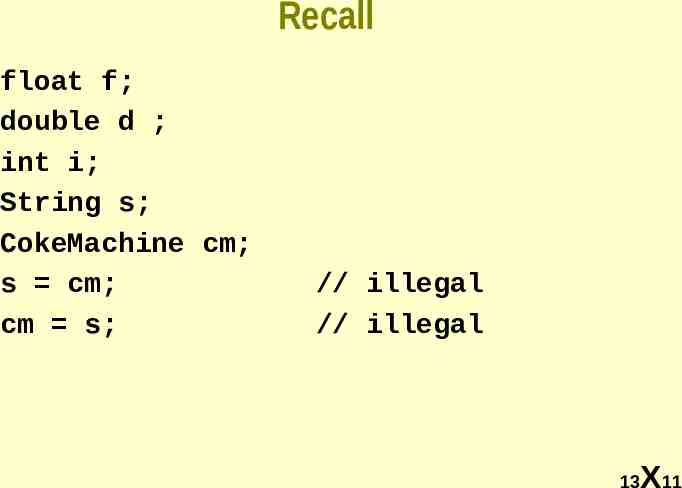
Recall float f; double d ; int i; String s; CokeMachine cm; s cm; cm s; // illegal // illegal X11 13
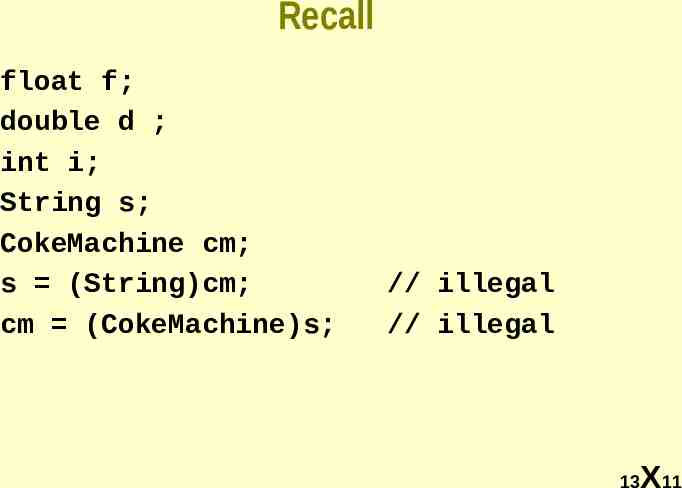
Recall float f; double d ; int i; String s; CokeMachine cm; s (String)cm; cm (CokeMachine)s; // illegal // illegal X11 13
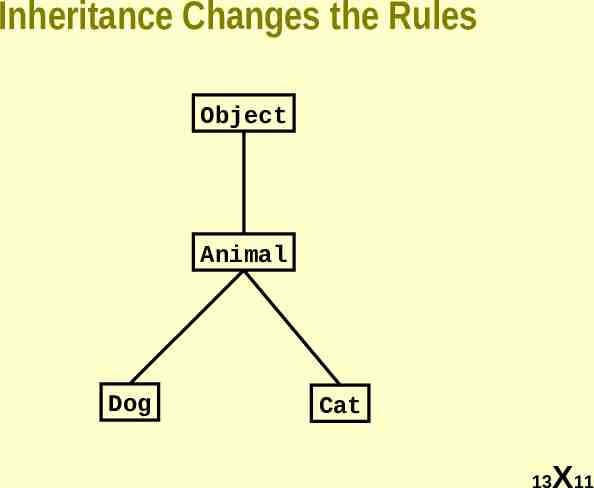
Inheritance Changes the Rules Object Animal Dog Cat X11 13
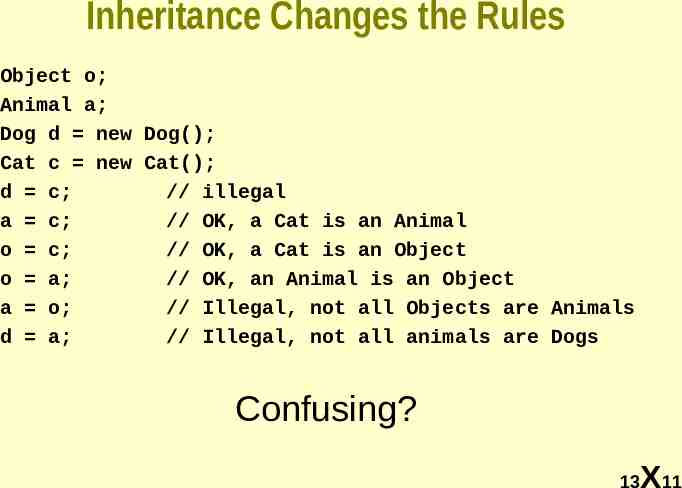
Inheritance Changes the Rules Object o; Animal a; Dog d new Dog(); Cat c new Cat(); d c; // illegal a c; // OK, a Cat is an Animal o c; // OK, a Cat is an Object o a; // OK, an Animal is an Object a o; // Illegal, not all Objects are Animals d a; // Illegal, not all animals are Dogs Confusing? X11 13
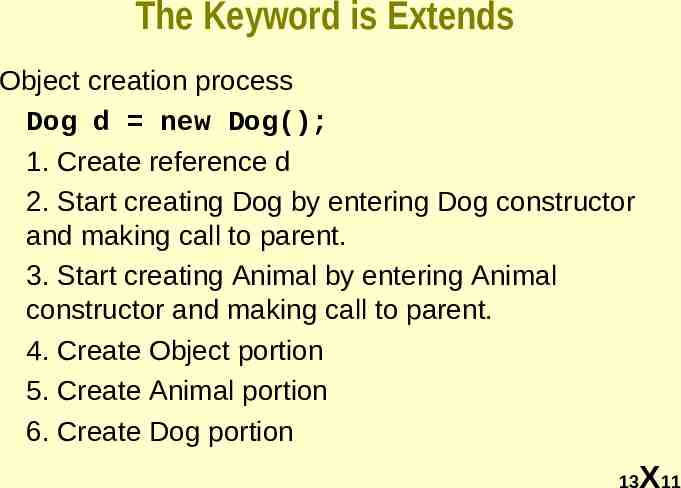
The Keyword is Extends Object creation process Dog d new Dog(); 1. Create reference d 2. Start creating Dog by entering Dog constructor and making call to parent. 3. Start creating Animal by entering Animal constructor and making call to parent. 4. Create Object portion 5. Create Animal portion 6. Create Dog portion X11 13
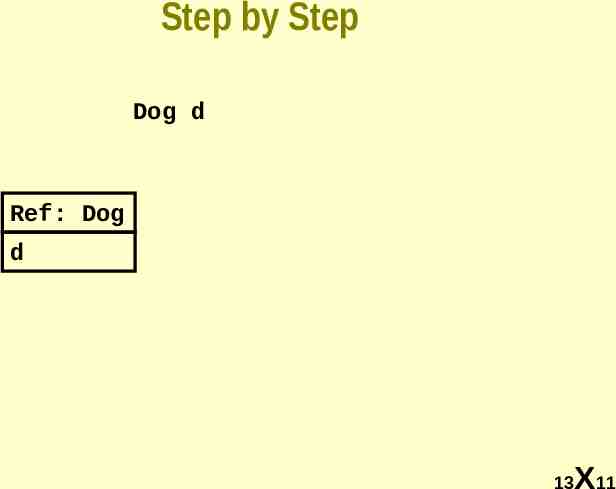
Step by Step Dog d Ref: Dog d X11 13
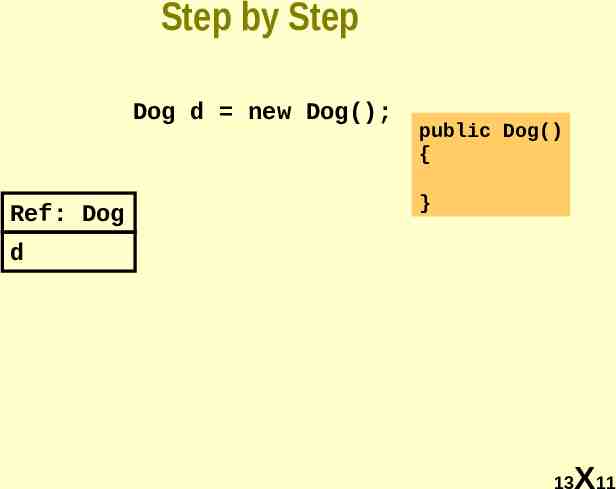
Step by Step Dog d new Dog(); Ref: Dog public Dog() { } d X11 13
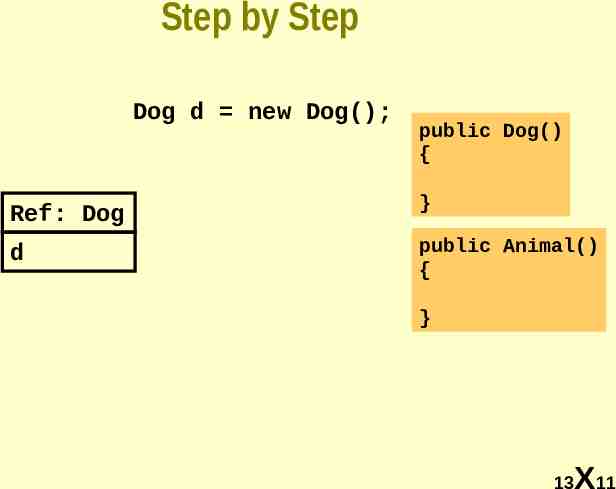
Step by Step Dog d new Dog(); public Dog() { Ref: Dog } d public Animal() { } X11 13
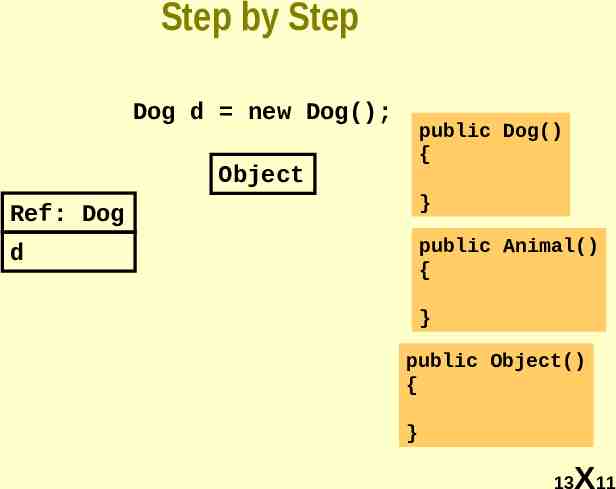
Step by Step Dog d new Dog(); public Dog() { Object Ref: Dog } d public Animal() { } public Object() { } X11 13
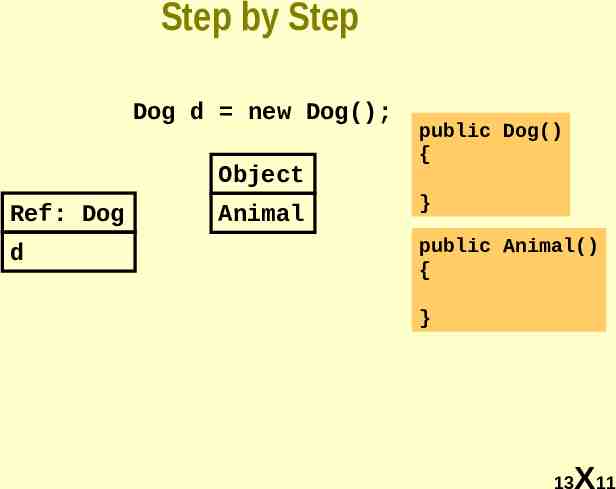
Step by Step Dog d new Dog(); Object Ref: Dog d Animal public Dog() { } public Animal() { } X11 13
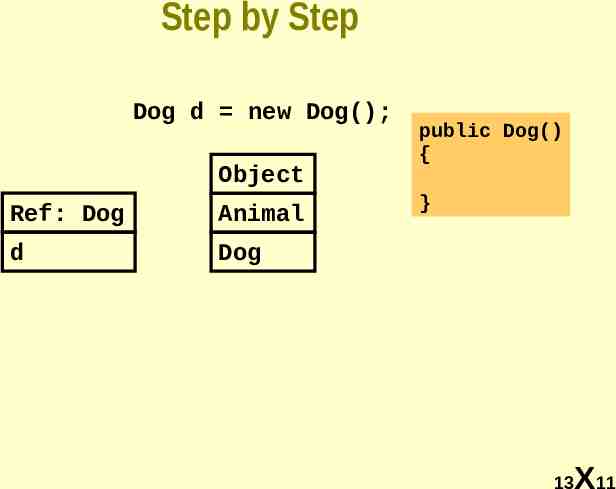
Step by Step Dog d new Dog(); Object Ref: Dog Animal d Dog public Dog() { } X11 13
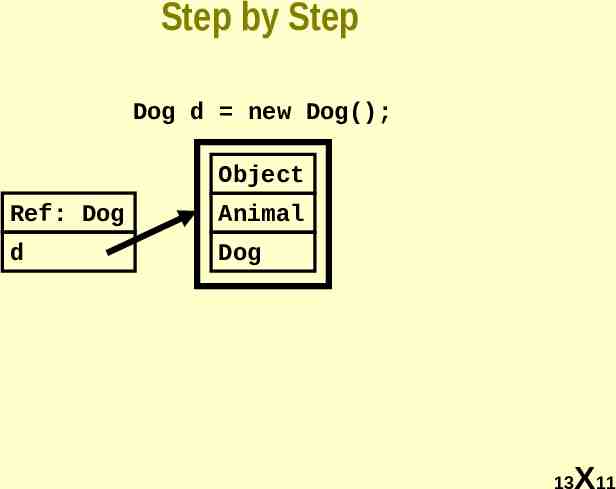
Step by Step Dog d new Dog(); Object Ref: Dog Animal d Dog X11 13
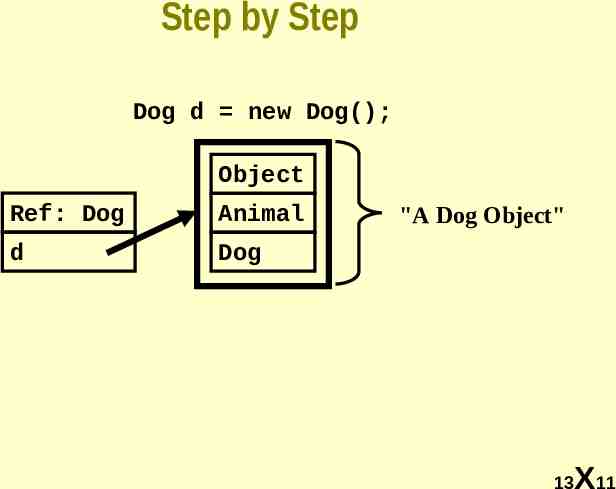
Step by Step Dog d new Dog(); Object Ref: Dog Animal d Dog "A Dog Object" X11 13
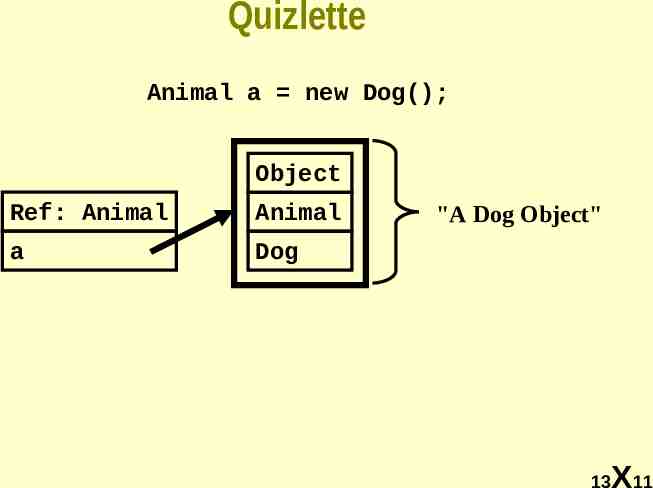
Quizlette Animal a new Dog(); Object Ref: Animal Animal a Dog "A Dog Object" X11 13
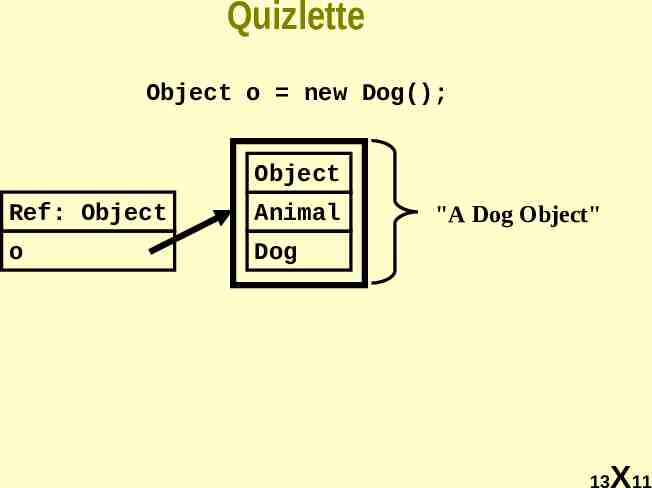
Quizlette Object o new Dog(); Object Ref: Object Animal o Dog "A Dog Object" X11 13
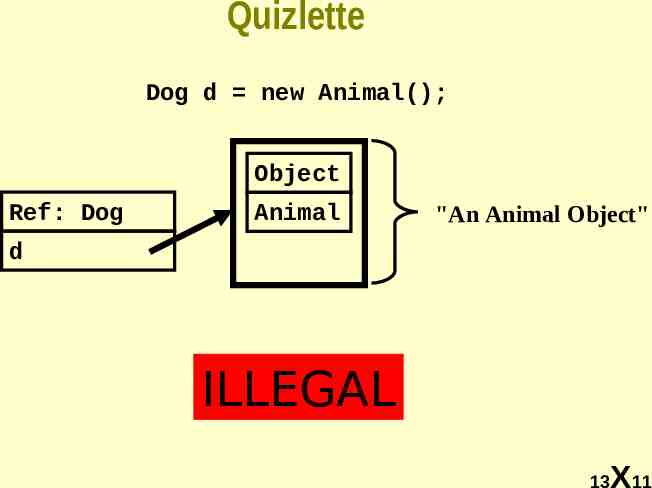
Quizlette Dog d new Animal(); Object Ref: Dog Animal "An Animal Object" d ILLEGAL X11 13
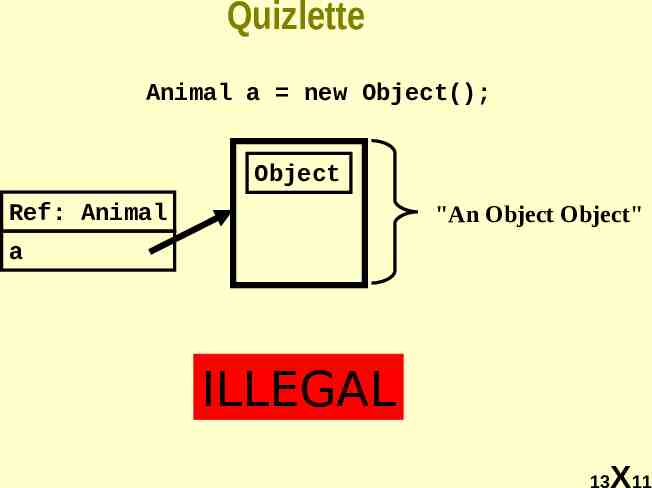
Quizlette Animal a new Object(); Object Ref: Animal "An Object Object" a ILLEGAL X11 13
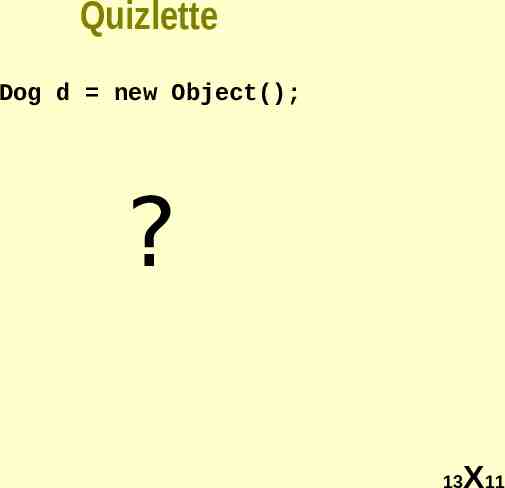
Quizlette Dog d new Object(); ? X11 13
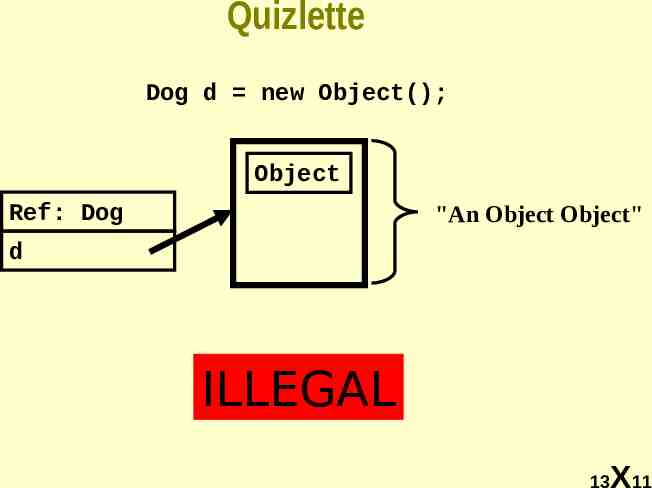
Quizlette Dog d new Object(); Object Ref: Dog "An Object Object" d ILLEGAL X11 13
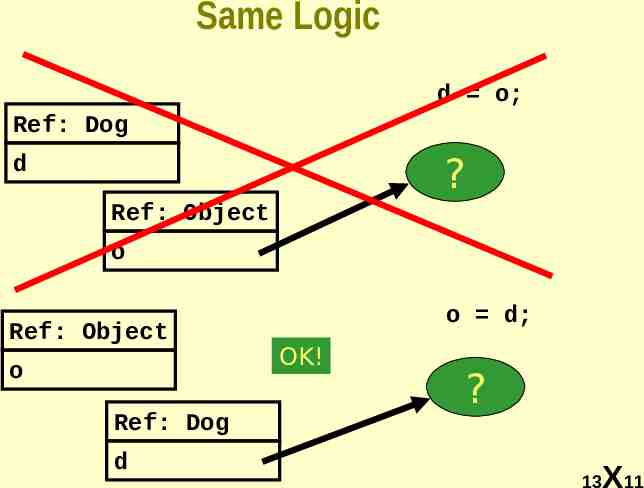
Same Logic d o; Ref: Dog d ? Ref: Object o Ref: Object o Ref: Dog d o d; OK! ? X11 13
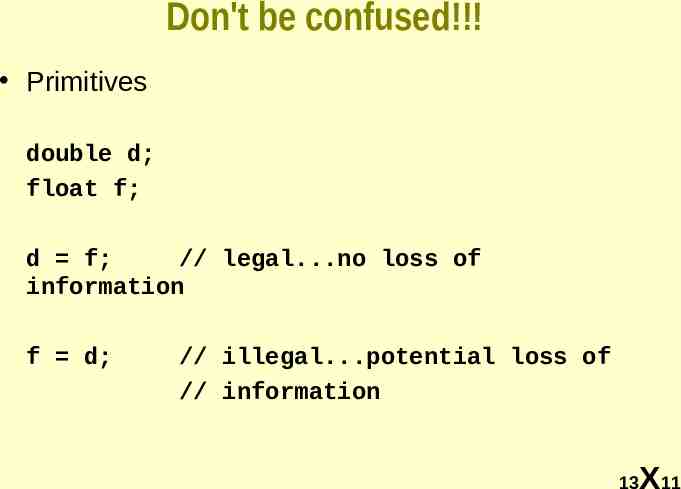
Don't be confused!!! Primitives double d; float f; d f; // legal.no loss of information f d; // illegal.potential loss of // information X11 13
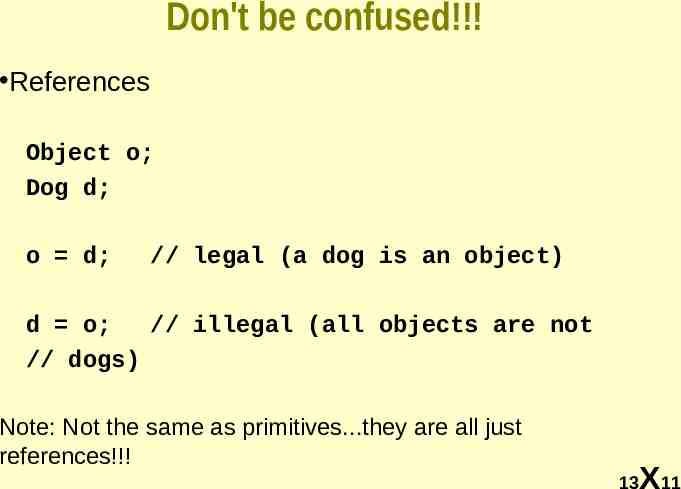
Don't be confused!!! References Object o; Dog d; o d; // legal (a dog is an object) d o; // illegal (all objects are not // dogs) Note: Not the same as primitives.they are all just references!!! X11 13
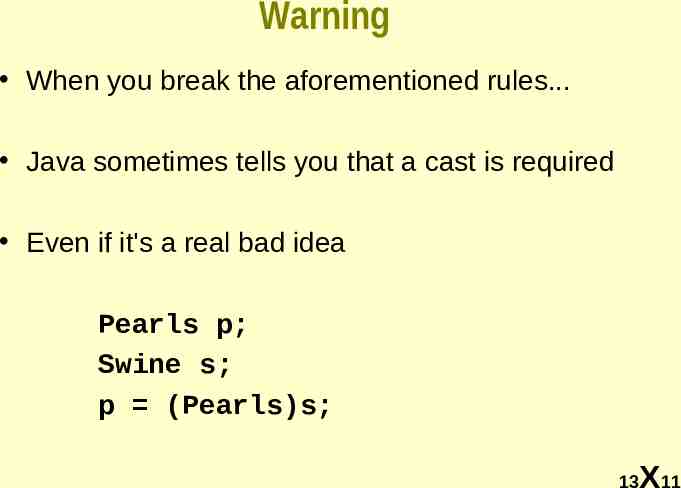
Warning When you break the aforementioned rules. Java sometimes tells you that a cast is required Even if it's a real bad idea Pearls p; Swine s; p (Pearls)s; X11 13
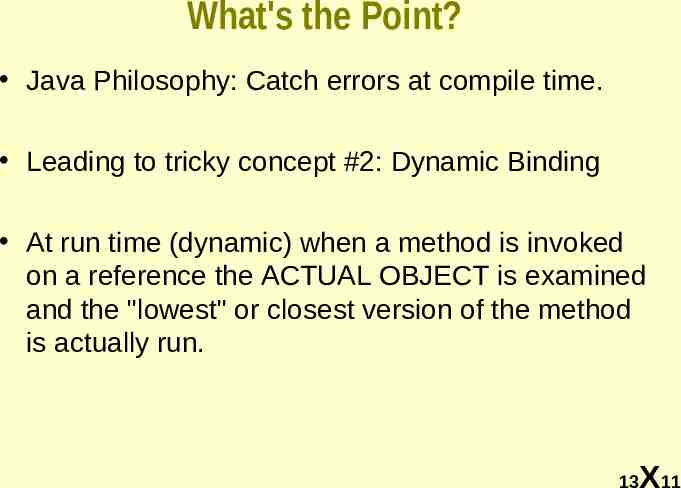
What's the Point? Java Philosophy: Catch errors at compile time. Leading to tricky concept #2: Dynamic Binding At run time (dynamic) when a method is invoked on a reference the ACTUAL OBJECT is examined and the "lowest" or closest version of the method is actually run. X11 13
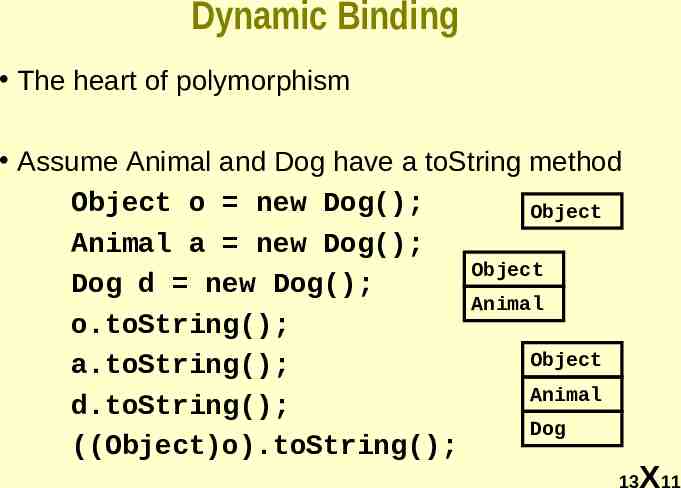
Dynamic Binding The heart of polymorphism Assume Animal and Dog have a toString method Object o new Dog(); Object Animal a new Dog(); Object Dog d new Dog(); Animal o.toString(); Object a.toString(); Animal d.toString(); Dog ((Object)o).toString(); X11 13
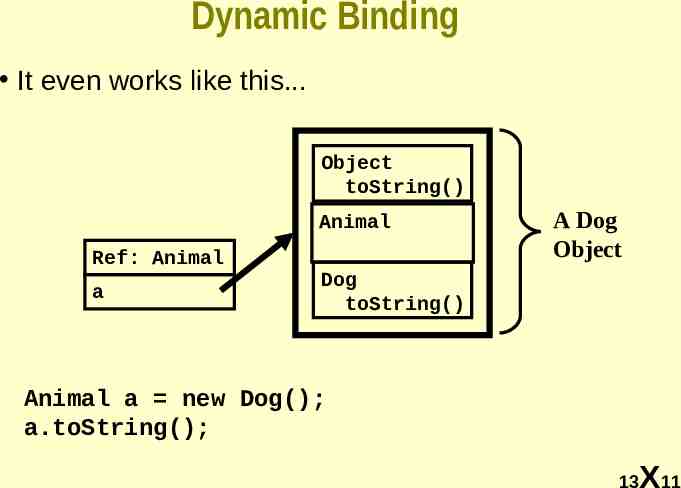
Dynamic Binding It even works like this. Object toString() Animal Ref: Animal a A Dog Object Dog toString() Animal a new Dog(); a.toString(); X11 13
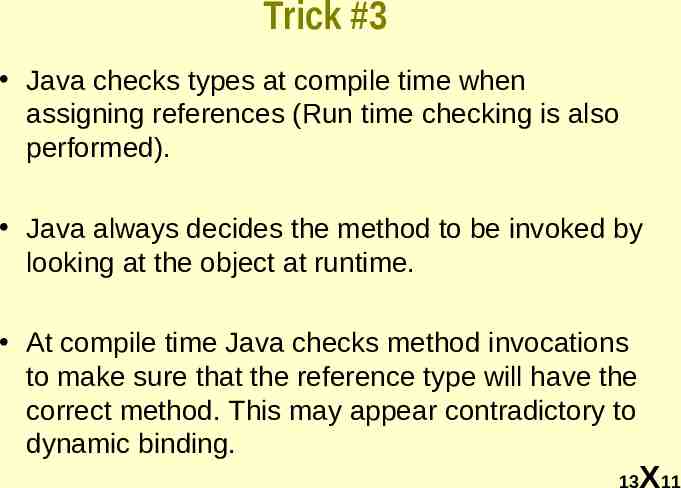
Trick #3 Java checks types at compile time when assigning references (Run time checking is also performed). Java always decides the method to be invoked by looking at the object at runtime. At compile time Java checks method invocations to make sure that the reference type will have the correct method. This may appear contradictory to dynamic binding. X11 13
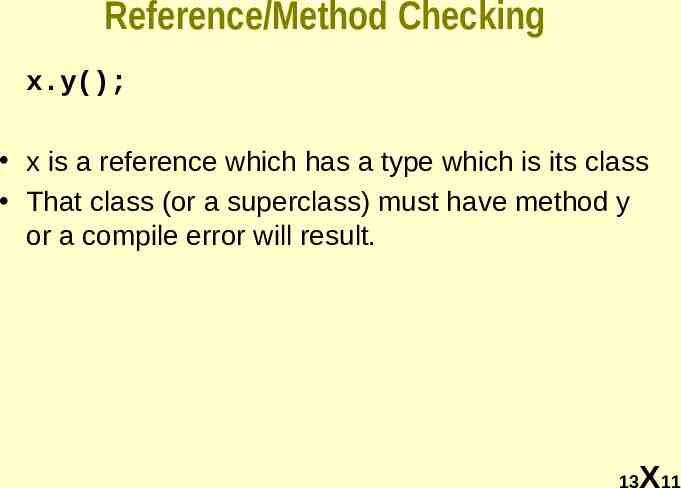
Reference/Method Checking x.y(); x is a reference which has a type which is its class That class (or a superclass) must have method y or a compile error will result. X11 13

More Quizlette Fun Object o new Dog(); Animal a new Dog(); Dog d new Dog(); o.toString(); o.move(); o.bark(); a.toString(); a.move(); a.bark(); d.toString(); d.move(); d.bark(); Object toString() o a d Object Animal toString() move() Animal Dog move() Object move()toString() Dog toString() Animal move() bark() move() toString() bark() Dog move() toString() bark() X11 13
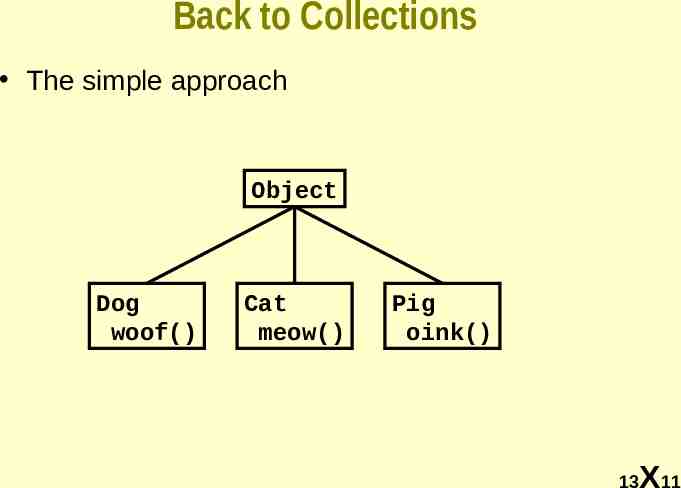
Back to Collections The simple approach Object Dog woof() Cat meow() Pig oink() X11 13
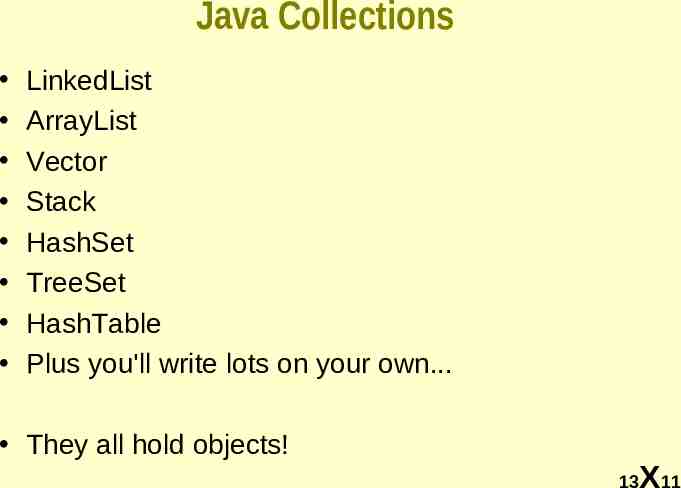
Java Collections LinkedList ArrayList Vector Stack HashSet TreeSet HashTable Plus you'll write lots on your own. They all hold objects! X11 13
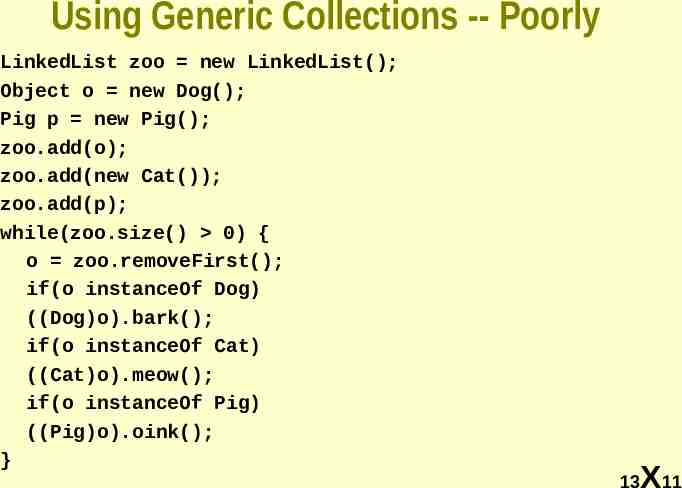
Using Generic Collections -- Poorly LinkedList zoo new LinkedList(); Object o new Dog(); Pig p new Pig(); zoo.add(o); zoo.add(new Cat()); zoo.add(p); while(zoo.size() 0) { o zoo.removeFirst(); if(o instanceOf Dog) ((Dog)o).bark(); if(o instanceOf Cat) ((Cat)o).meow(); if(o instanceOf Pig) ((Pig)o).oink(); } X11 13
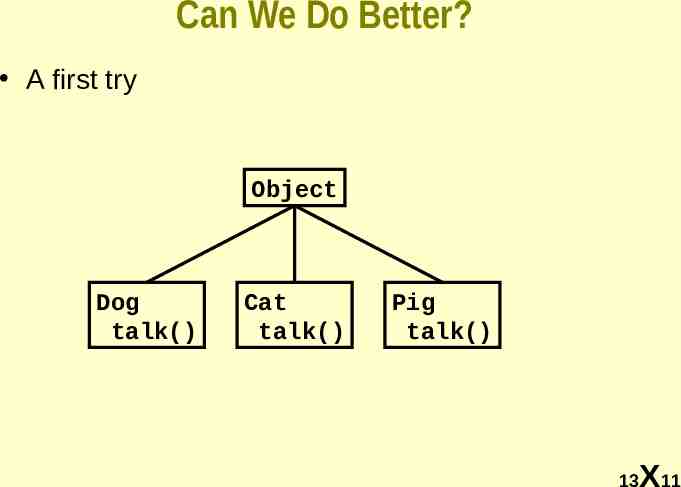
Can We Do Better? A first try Object Dog talk() Cat talk() Pig talk() X11 13
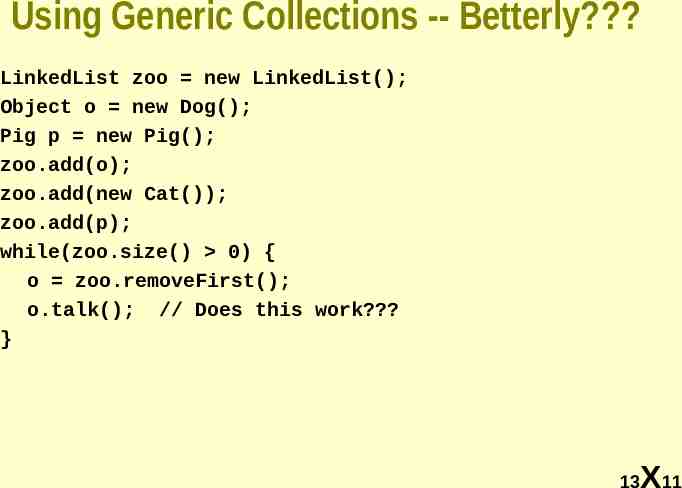
Using Generic Collections -- Betterly? LinkedList zoo new LinkedList(); Object o new Dog(); Pig p new Pig(); zoo.add(o); zoo.add(new Cat()); zoo.add(p); while(zoo.size() 0) { o zoo.removeFirst(); o.talk(); // Does this work? } X11 13
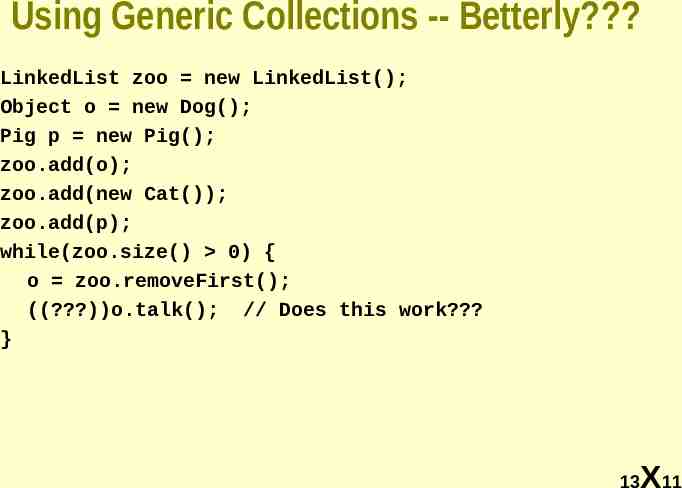
Using Generic Collections -- Betterly? LinkedList zoo new LinkedList(); Object o new Dog(); Pig p new Pig(); zoo.add(o); zoo.add(new Cat()); zoo.add(p); while(zoo.size() 0) { o zoo.removeFirst(); ((?))o.talk(); // Does this work? } X11 13
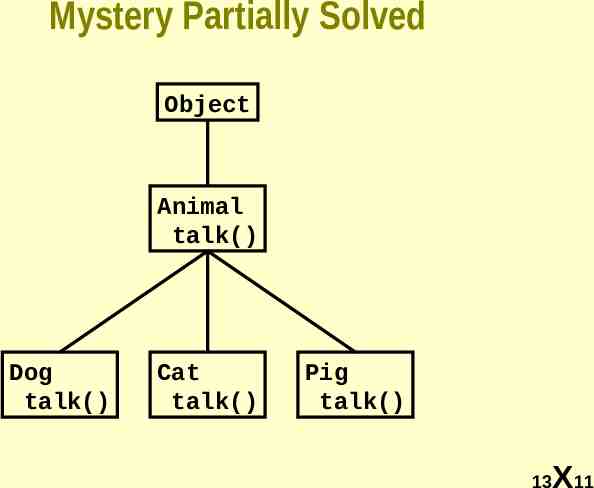
Mystery Partially Solved Object Animal talk() Dog talk() Cat talk() Pig talk() X11 13
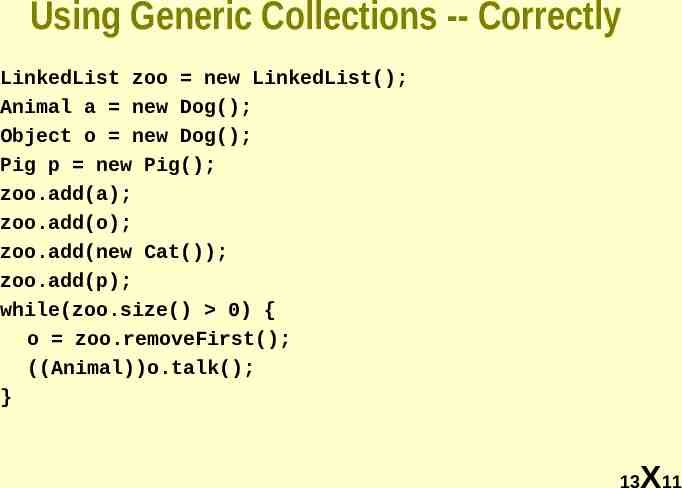
Using Generic Collections -- Correctly LinkedList zoo new LinkedList(); Animal a new Dog(); Object o new Dog(); Pig p new Pig(); zoo.add(a); zoo.add(o); zoo.add(new Cat()); zoo.add(p); while(zoo.size() 0) { o zoo.removeFirst(); ((Animal))o.talk(); } X11 13
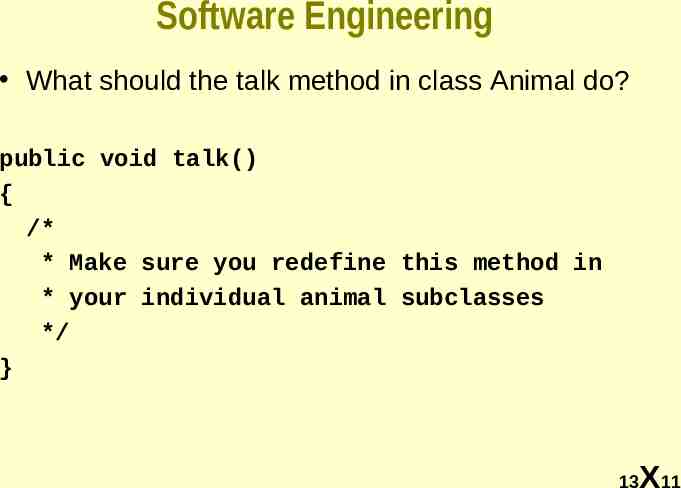
Software Engineering What should the talk method in class Animal do? public void talk() { /* * Make sure you redefine this method in * your individual animal subclasses */ } X11 13
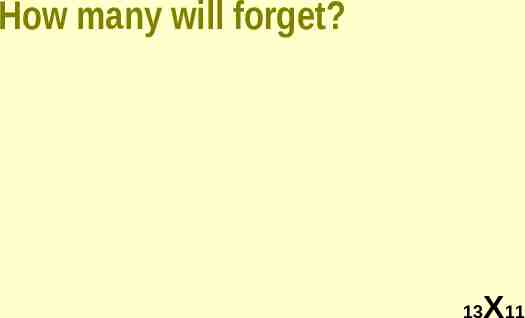
How many will forget? X11 13
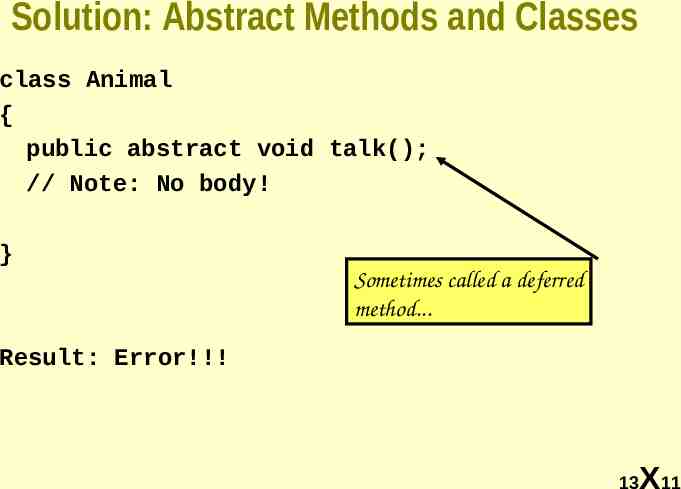
Solution: Abstract Methods and Classes class Animal { public abstract void talk(); // Note: No body! } Sometimes called a deferred method. Result: Error!!! X11 13
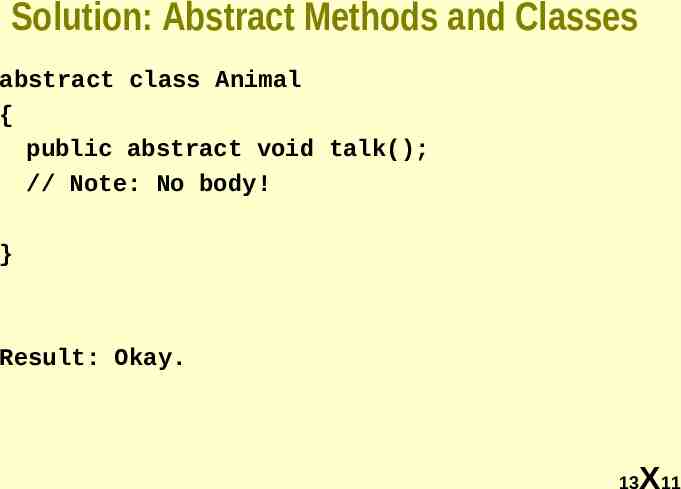
Solution: Abstract Methods and Classes abstract class Animal { public abstract void talk(); // Note: No body! } Result: Okay. X11 13
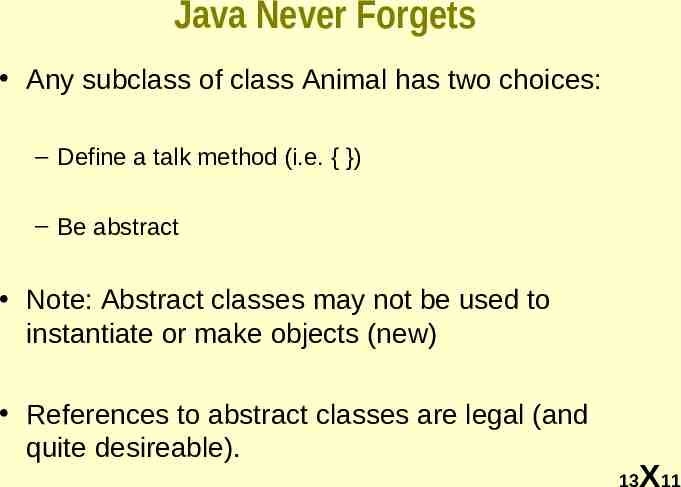
Java Never Forgets Any subclass of class Animal has two choices: – Define a talk method (i.e. { }) – Be abstract Note: Abstract classes may not be used to instantiate or make objects (new) References to abstract classes are legal (and quite desireable). X11 13
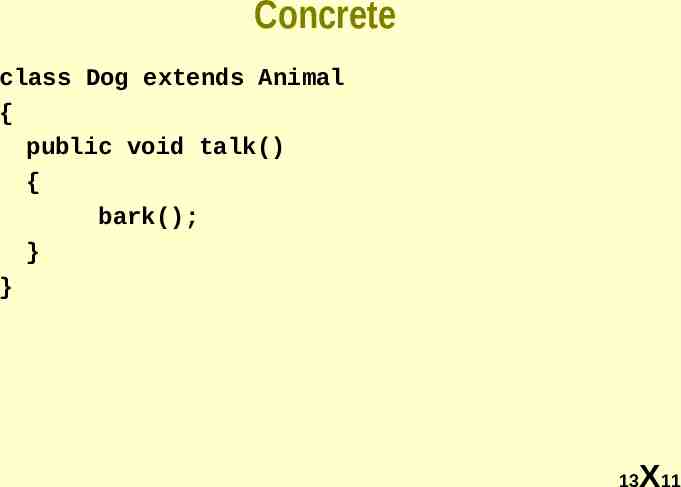
Concrete class Dog extends Animal { public void talk() { bark(); } } X11 13
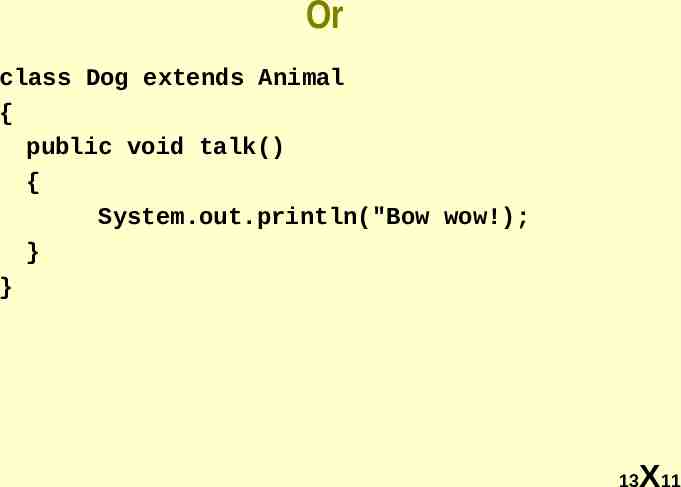
Or class Dog extends Animal { public void talk() { System.out.println("Bow wow!); } } X11 13
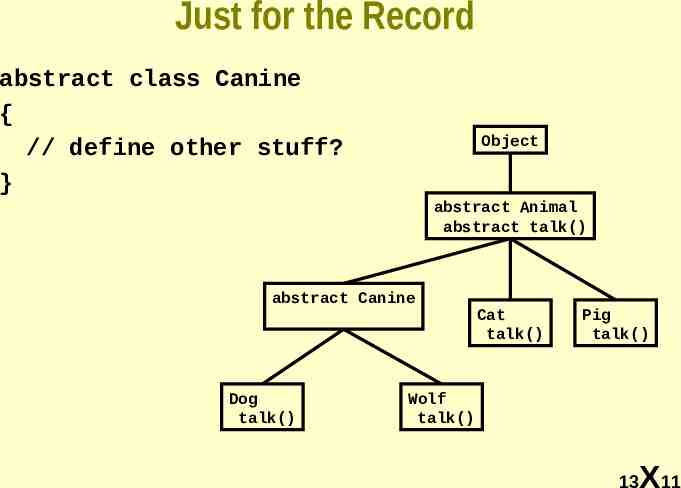
Just for the Record abstract class Canine { // define other stuff? } Object abstract Animal abstract talk() abstract Canine Dog talk() Cat talk() Pig talk() Wolf talk() X11 13
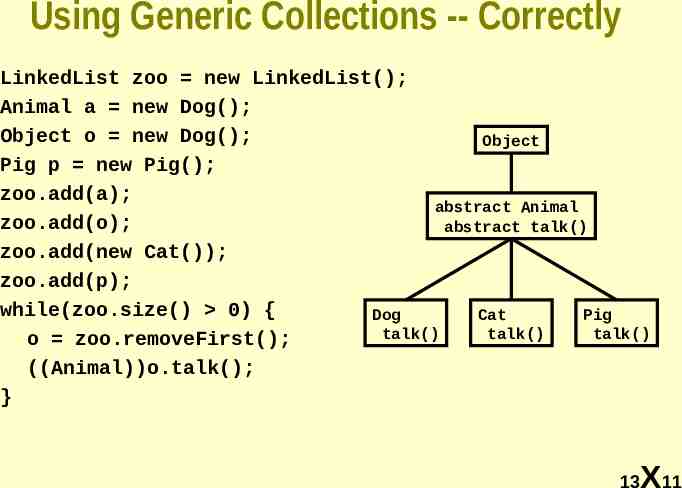
Using Generic Collections -- Correctly LinkedList zoo new LinkedList(); Animal a new Dog(); Object o new Dog(); Object Pig p new Pig(); zoo.add(a); abstract Animal zoo.add(o); abstract talk() zoo.add(new Cat()); zoo.add(p); while(zoo.size() 0) { Dog Cat Pig talk() talk() talk() o zoo.removeFirst(); ((Animal))o.talk(); } X11 13
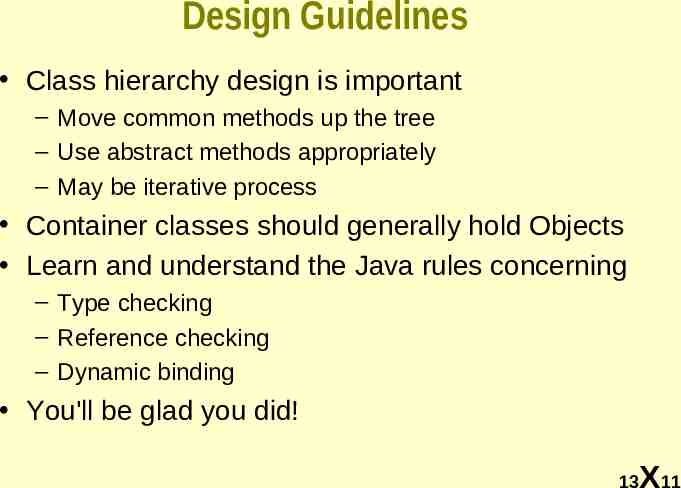
Design Guidelines Class hierarchy design is important – Move common methods up the tree – Use abstract methods appropriately – May be iterative process Container classes should generally hold Objects Learn and understand the Java rules concerning – Type checking – Reference checking – Dynamic binding You'll be glad you did! X11 13
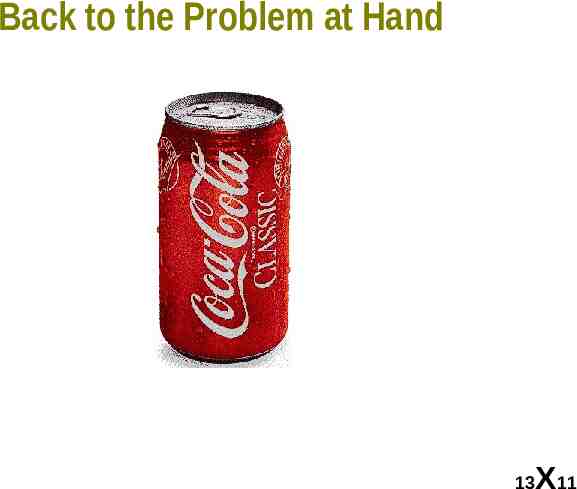
Back to the Problem at Hand X11 13
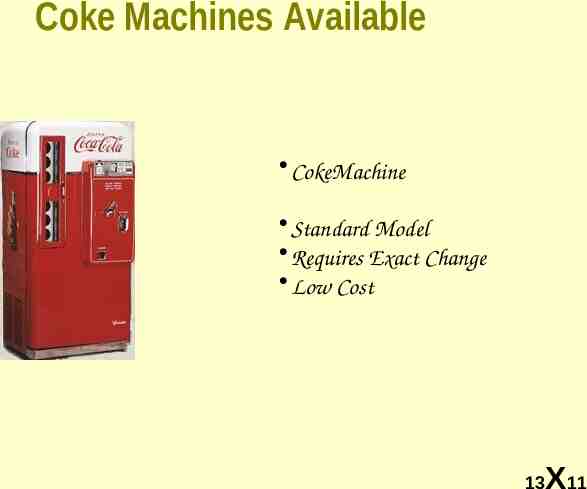
Coke Machines Available CokeMachine Standard Model Requires Exact Change Low Cost X11 13
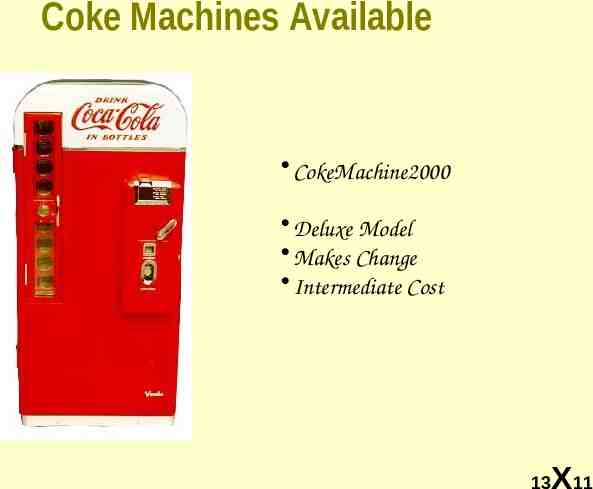
Coke Machines Available CokeMachine2000 Deluxe Model Makes Change Intermediate Cost X11 13
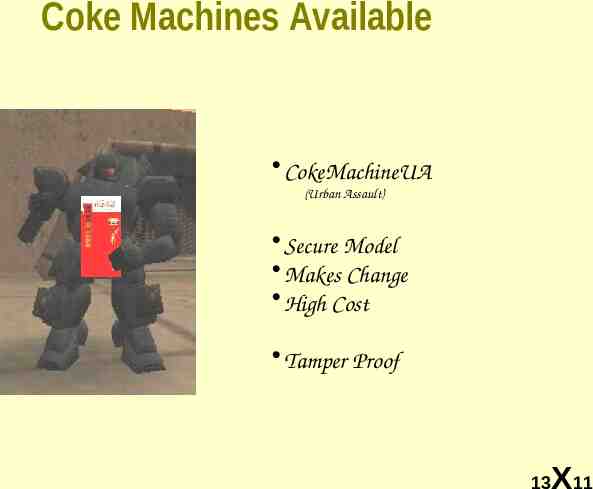
Coke Machines Available CokeMachineUA (Urban Assault) Secure Model Makes Change High Cost Tamper Proof X11 13
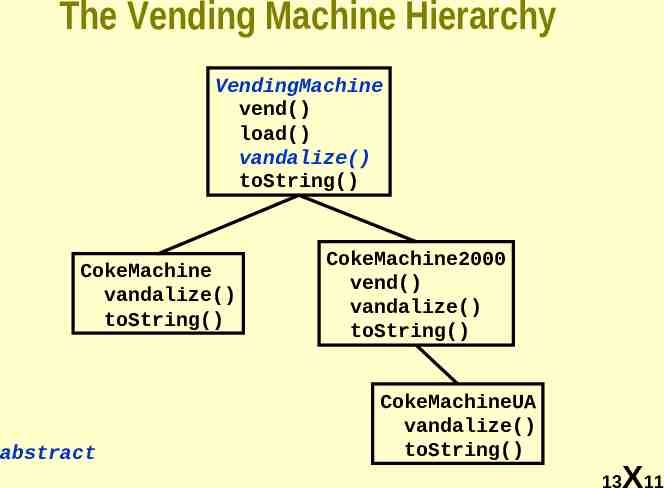
The Vending Machine Hierarchy VendingMachine vend() load() vandalize() toString() CokeMachine vandalize() toString() abstract CokeMachine2000 vend() vandalize() toString() CokeMachineUA vandalize() toString() X11 13
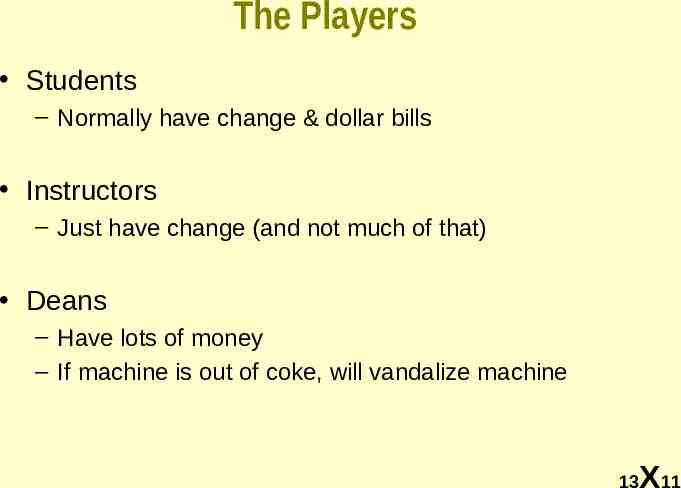
The Players Students – Normally have change & dollar bills Instructors – Just have change (and not much of that) Deans – Have lots of money – If machine is out of coke, will vandalize machine X11 13
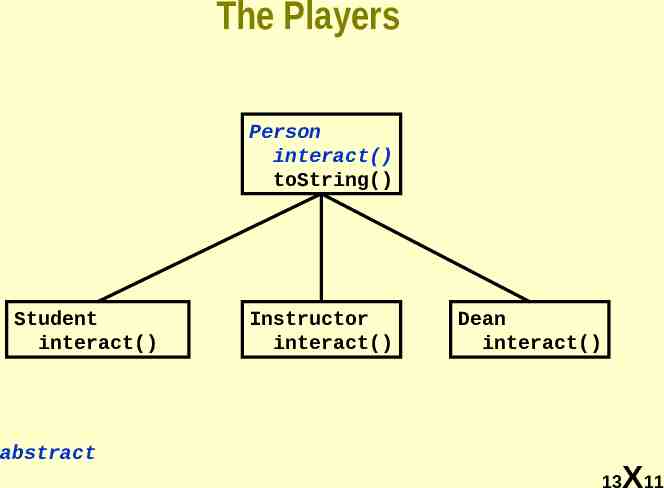
The Players Person interact() toString() Student interact() abstract Instructor interact() Dean interact() X11 13
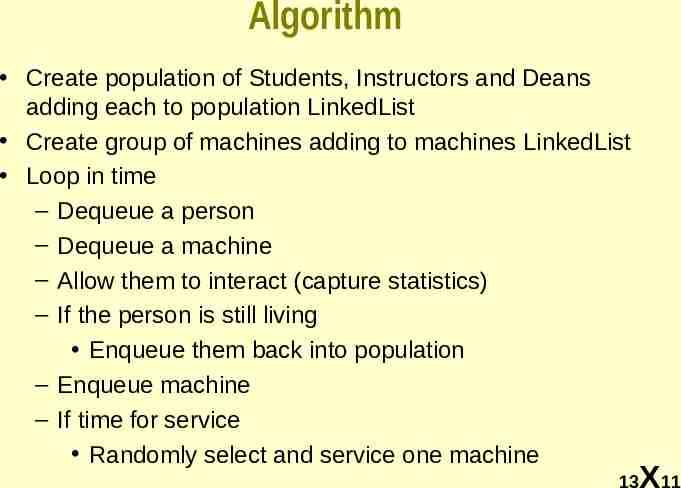
Algorithm Create population of Students, Instructors and Deans adding each to population LinkedList Create group of machines adding to machines LinkedList Loop in time – Dequeue a person – Dequeue a machine – Allow them to interact (capture statistics) – If the person is still living Enqueue them back into population – Enqueue machine – If time for service Randomly select and service one machine X11 13
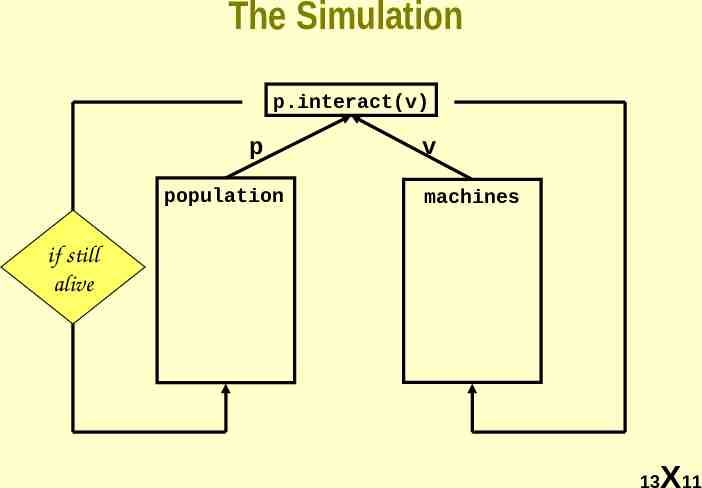
The Simulation p.interact(v) p population v machines if still alive X11 13
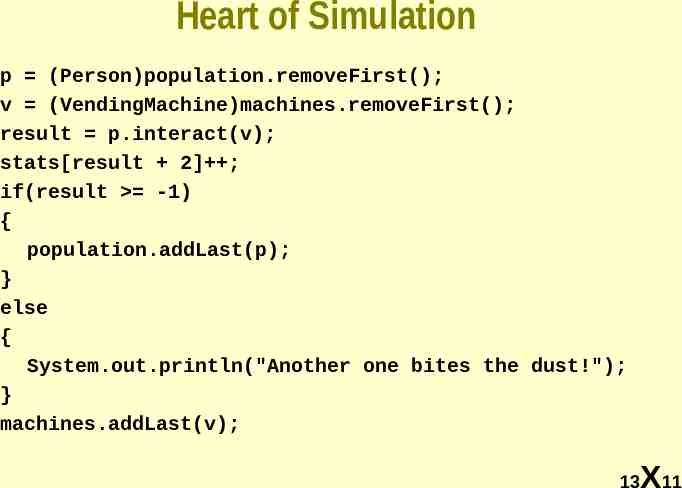
Heart of Simulation p (Person)population.removeFirst(); v (VendingMachine)machines.removeFirst(); result p.interact(v); stats[result 2] ; if(result -1) { population.addLast(p); } else { System.out.println("Another one bites the dust!"); } machines.addLast(v); X11 13
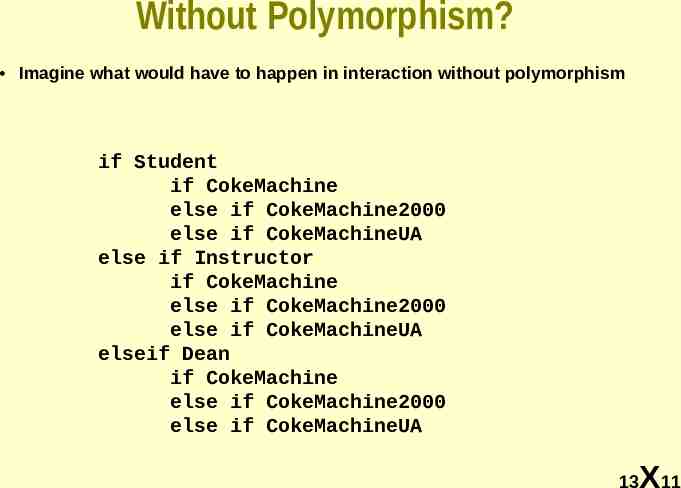
Without Polymorphism? Imagine what would have to happen in interaction without polymorphism if Student if CokeMachine else if CokeMachine2000 else if CokeMachineUA else if Instructor if CokeMachine else if CokeMachine2000 else if CokeMachineUA elseif Dean if CokeMachine else if CokeMachine2000 else if CokeMachineUA X11 13
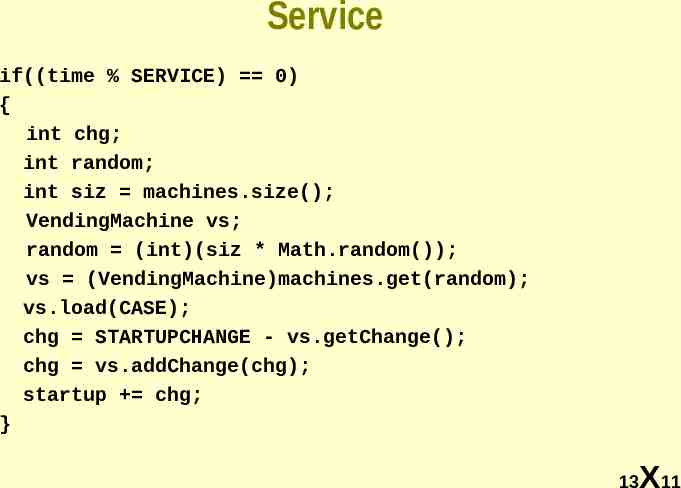
Service if((time % SERVICE) 0) { int chg; int random; int siz machines.size(); VendingMachine vs; random (int)(siz * Math.random()); vs (VendingMachine)machines.get(random); vs.load(CASE); chg STARTUPCHANGE - vs.getChange(); chg vs.addChange(chg); startup chg; } X11 13
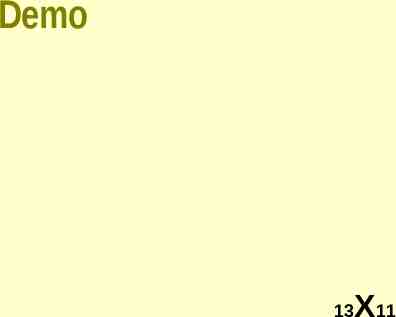
Demo X11 13

X11 13

X11 13