Huffman Coding: An Application of Binary Trees and Priority Queues
44 Slides331.50 KB
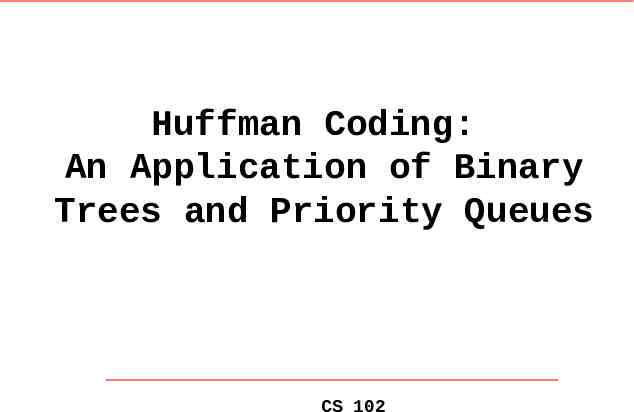
Huffman Coding: An Application of Binary Trees and Priority Queues CS 102
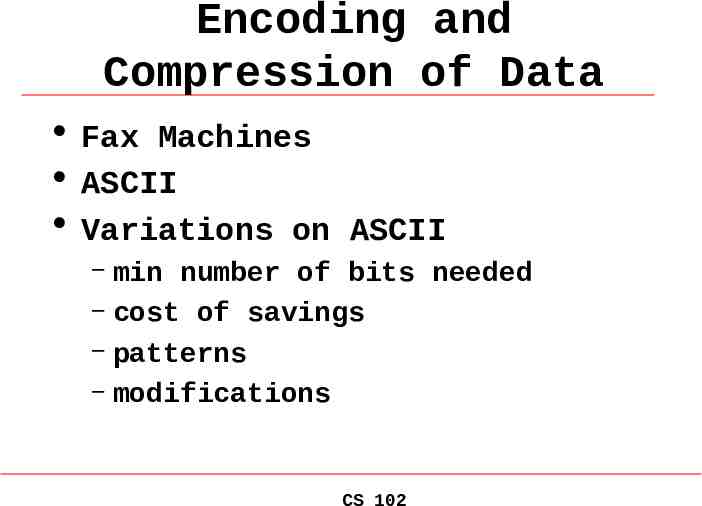
Encoding and Compression of Data Fax Machines ASCII Variations on ASCII – min number of bits needed – cost of savings – patterns – modifications CS 102
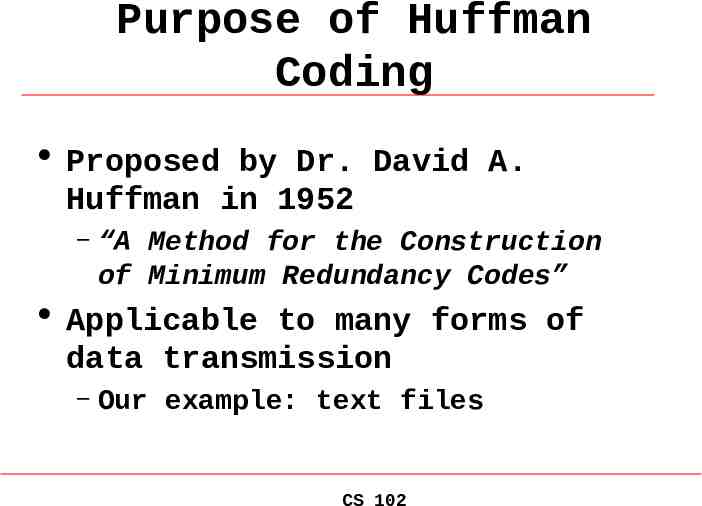
Purpose of Huffman Coding Proposed by Dr. David A. Huffman in 1952 – “A Method for the Construction of Minimum Redundancy Codes” Applicable to many forms of data transmission – Our example: text files CS 102
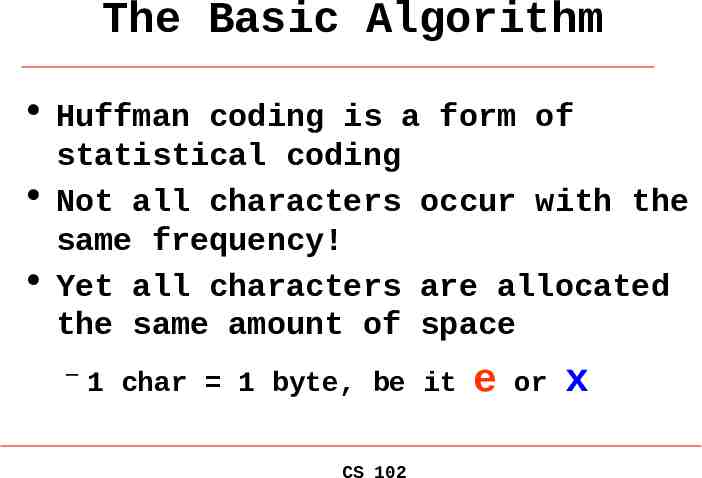
The Basic Algorithm Huffman coding is a form of statistical coding Not all characters occur with the same frequency! Yet all characters are allocated the same amount of space – 1 char 1 byte, be it CS 102 e or x
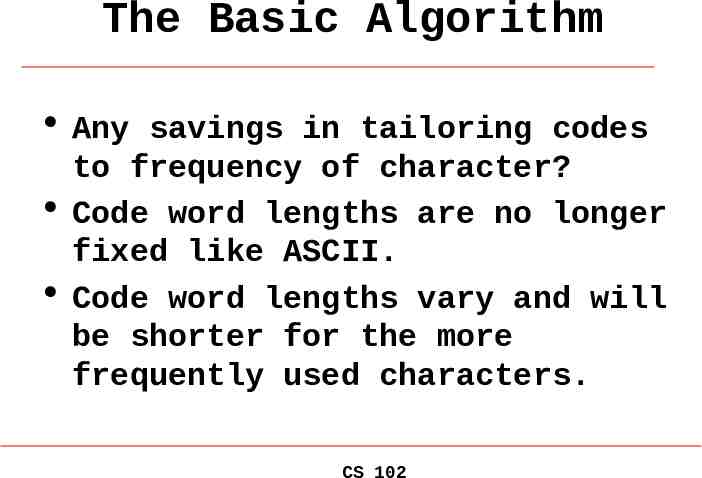
The Basic Algorithm Any savings in tailoring codes to frequency of character? Code word lengths are no longer fixed like ASCII. Code word lengths vary and will be shorter for the more frequently used characters. CS 102
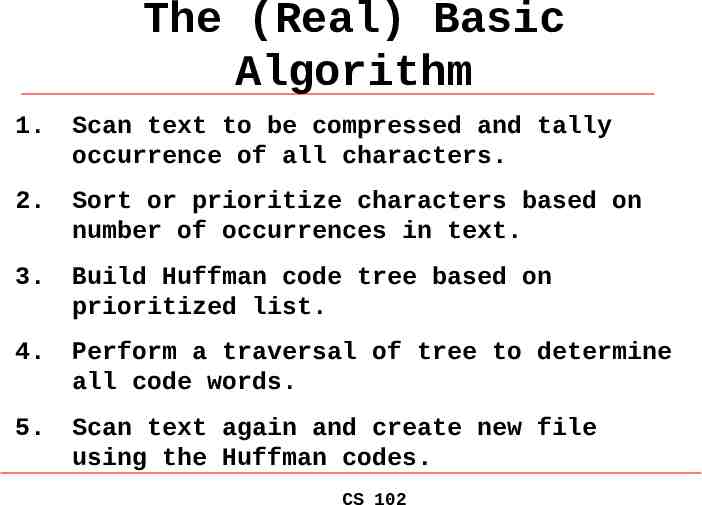
The (Real) Basic Algorithm 1. Scan text to be compressed and tally occurrence of all characters. 2. Sort or prioritize characters based on number of occurrences in text. 3. Build Huffman code tree based on prioritized list. 4. Perform a traversal of tree to determine all code words. 5. Scan text again and create new file using the Huffman codes. CS 102
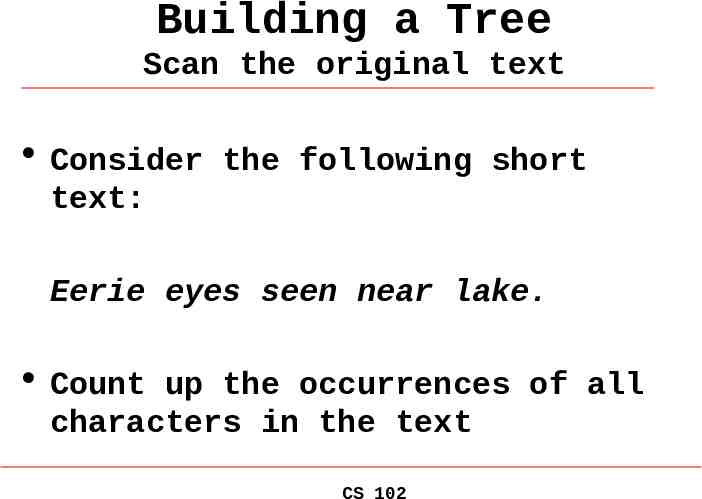
Building a Tree Scan the original text Consider the following short text: Eerie eyes seen near lake. Count up the occurrences of all characters in the text CS 102
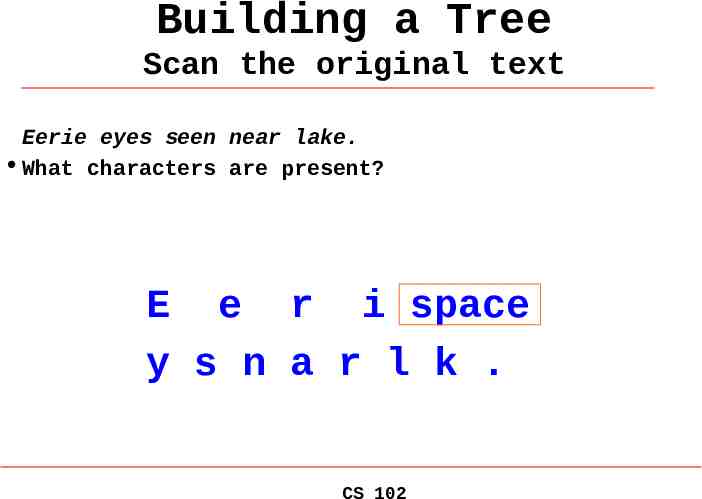
Building a Tree Scan the original text Eerie eyes seen near lake. What characters are present? E e r i space y s n a r l k . CS 102
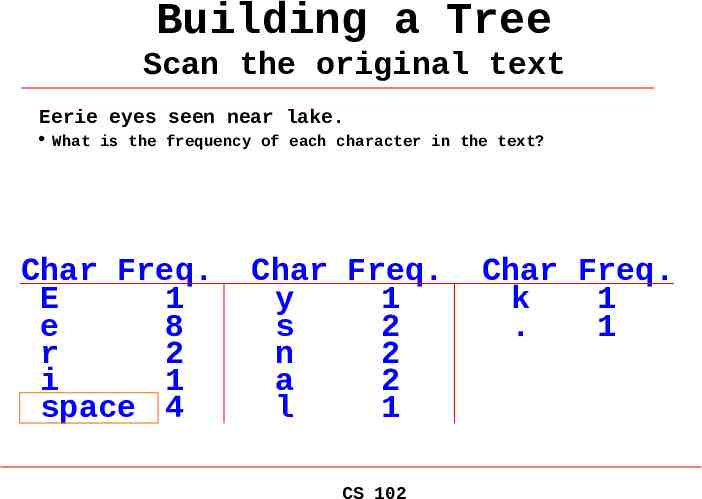
Building a Tree Scan the original text Eerie eyes seen near lake. What is the frequency of each character in the text? Char Freq. E 1 e 8 r 2 i 1 space 4 Char Freq. y 1 s 2 n 2 a 2 l 1 CS 102 Char Freq. k 1 . 1
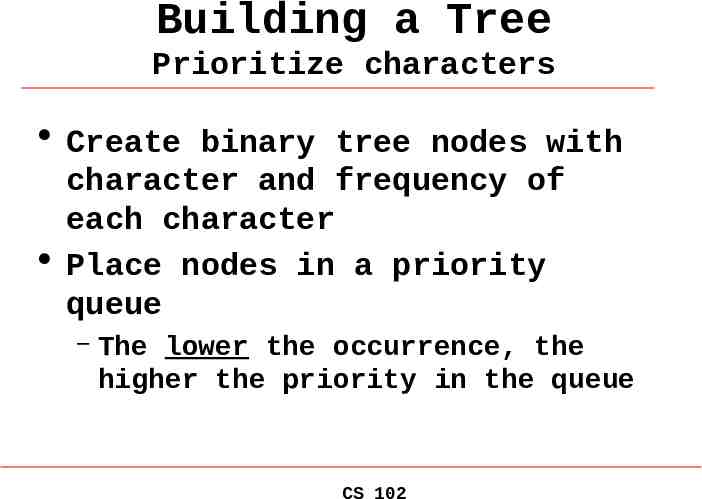
Building a Tree Prioritize characters Create binary tree nodes with character and frequency of each character Place nodes in a priority queue – The lower the occurrence, the higher the priority in the queue CS 102
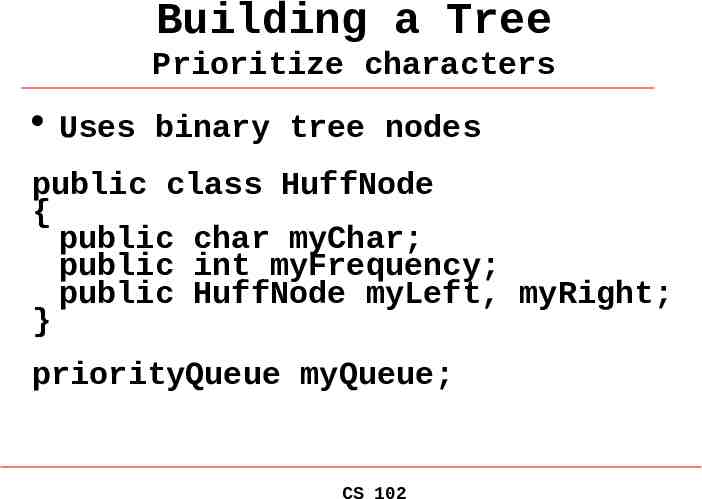
Building a Tree Prioritize characters Uses binary tree nodes public class HuffNode { public char myChar; public int myFrequency; public HuffNode myLeft, myRight; } priorityQueue myQueue; CS 102
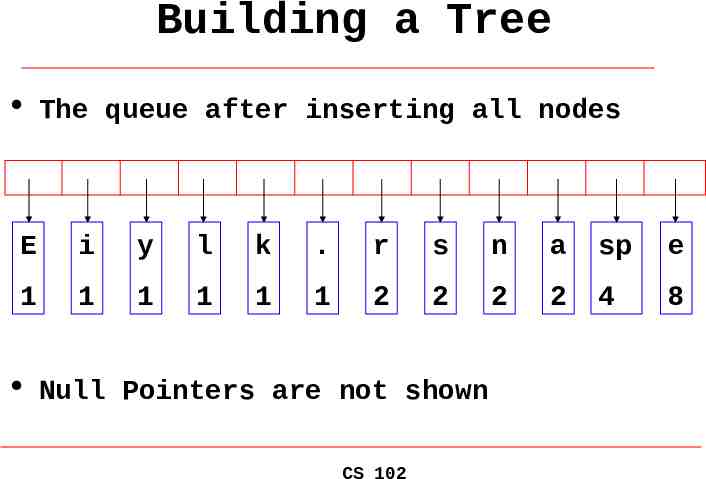
Building a Tree The queue after inserting all nodes E i y l k . r s n a sp e 1 1 1 1 1 1 2 2 2 2 4 8 Null Pointers are not shown CS 102
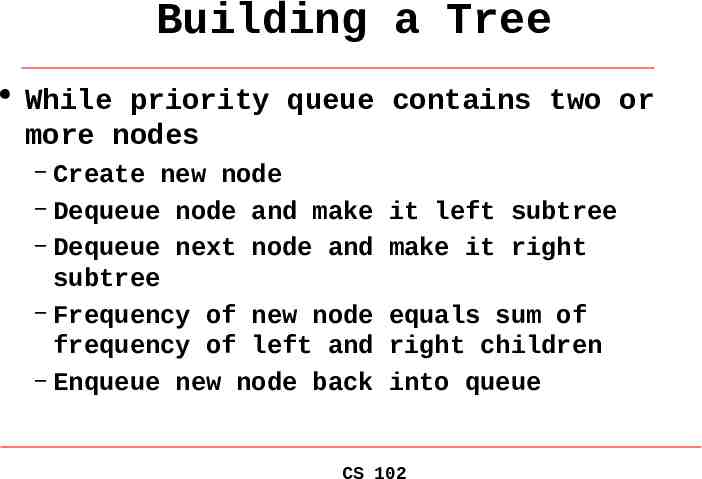
Building a Tree While priority queue contains two or more nodes – Create new node – Dequeue node and make – Dequeue next node and subtree – Frequency of new node frequency of left and – Enqueue new node back it left subtree make it right equals sum of right children into queue CS 102
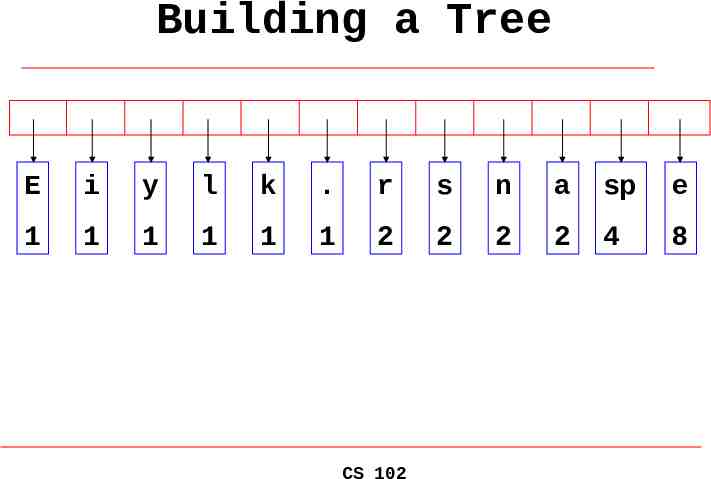
Building a Tree E i y l k . r s n a sp e 1 1 1 1 1 1 2 2 2 2 4 8 CS 102
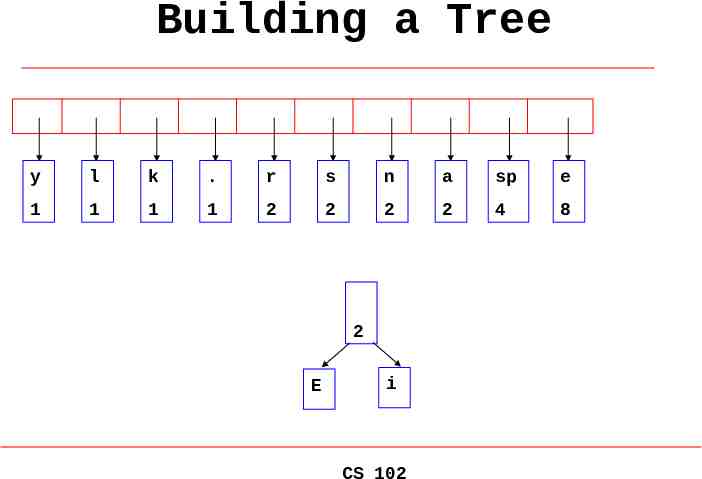
Building a Tree y l k . r s n a sp e 1 1 1 1 2 2 2 2 4 8 2 E i CS 102
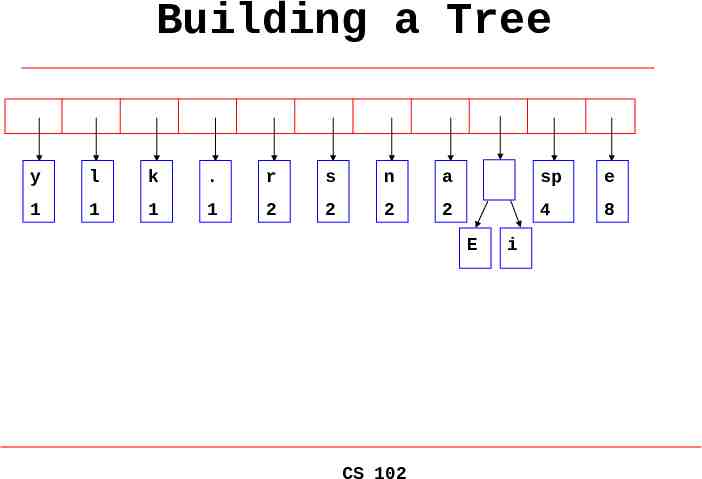
Building a Tree y l k . r s n a sp e 1 1 1 1 2 2 2 2 4 8 E CS 102 i
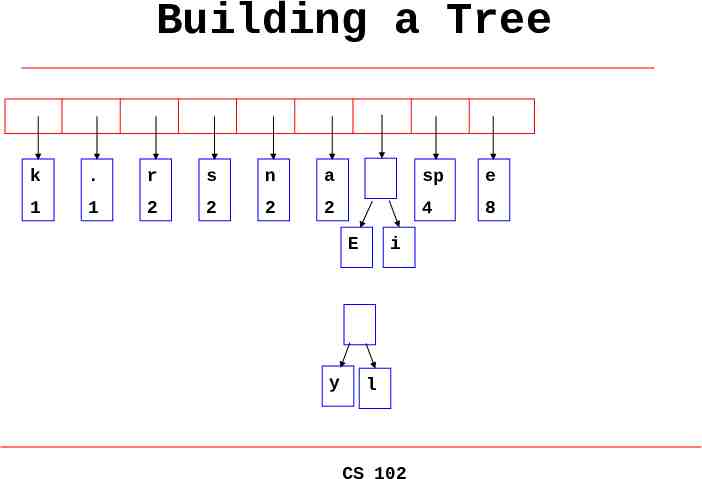
Building a Tree k . r s n a sp e 1 1 2 2 2 2 4 8 E y i l CS 102
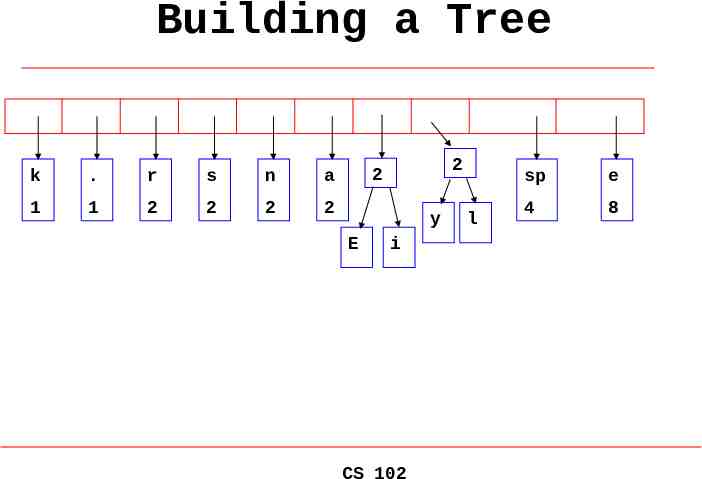
Building a Tree k . r s n a 1 1 2 2 2 2 2 2 y E i CS 102 l sp e 4 8
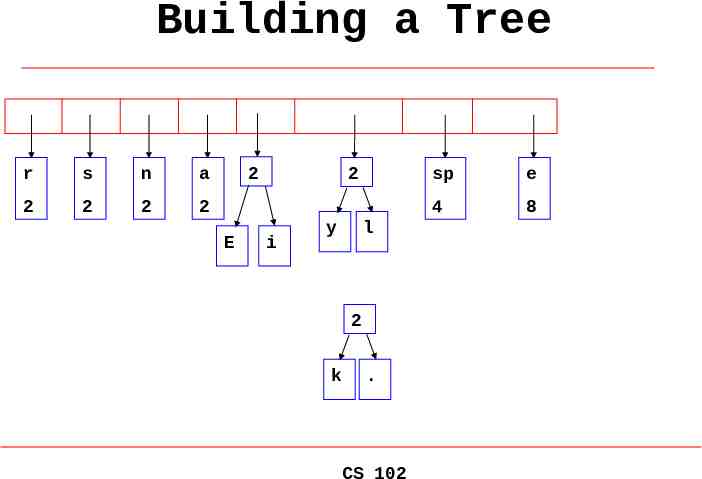
Building a Tree r s n a 2 2 2 2 2 E 2 i y l 2 k . CS 102 sp e 4 8
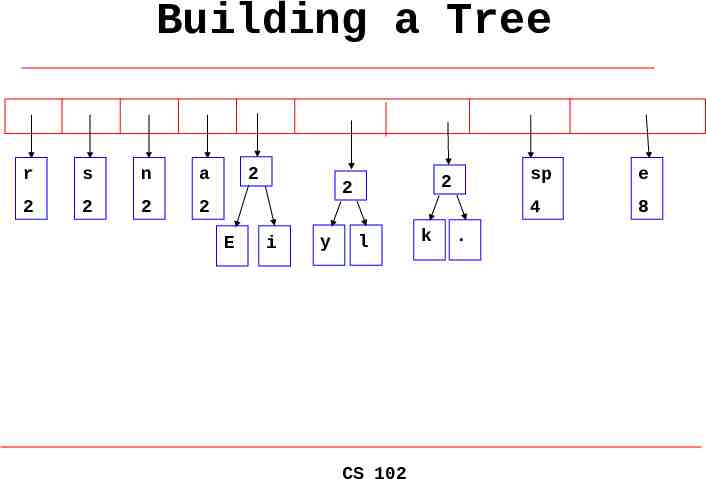
Building a Tree r s n a 2 2 2 2 2 E 2 2 i y l CS 102 k . sp e 4 8
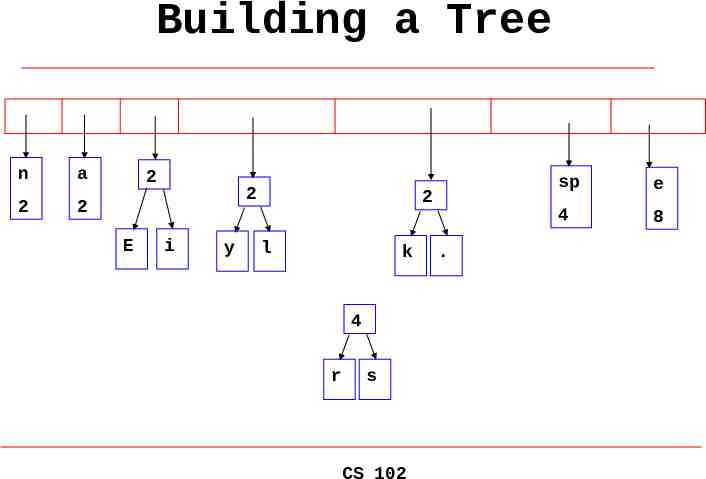
Building a Tree n a 2 2 2 E 2 i y 2 l k 4 r s CS 102 . sp e 4 8
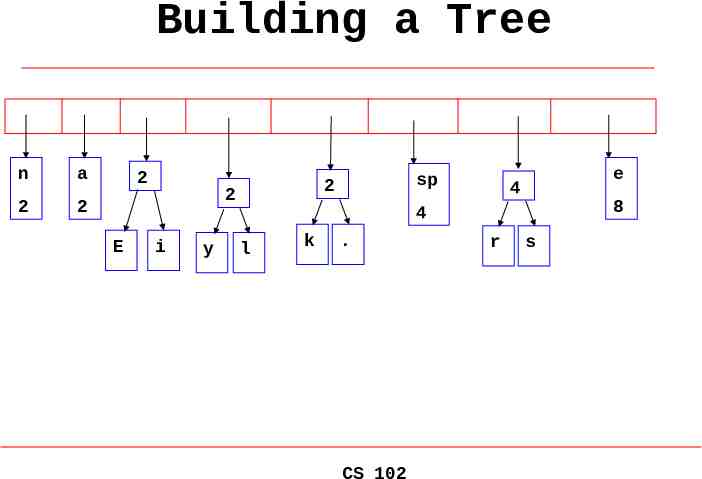
Building a Tree n a 2 2 2 E 2 i y sp 2 e 4 8 4 l k . CS 102 r s
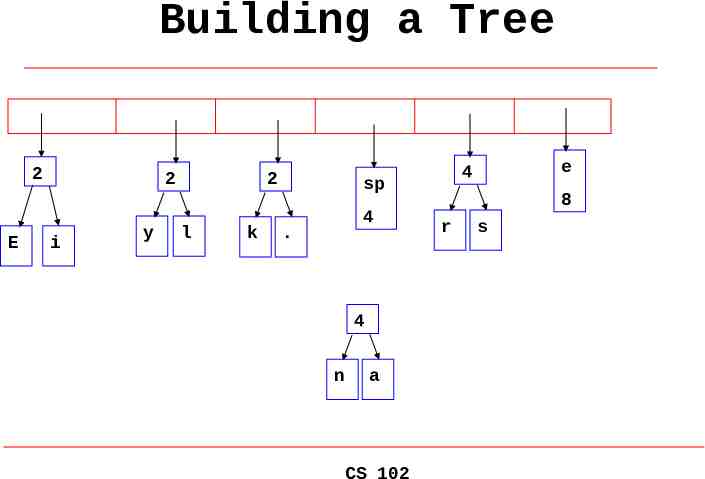
Building a Tree 2 E 2 i y 2 l k sp 4 . 4 n a CS 102 e 4 8 r s
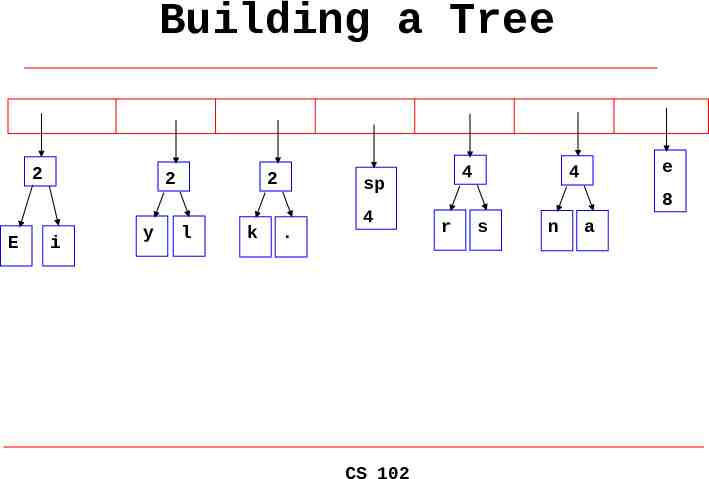
Building a Tree 2 E 2 i y 2 l k 4 sp . 4 CS 102 e 4 8 r s n a
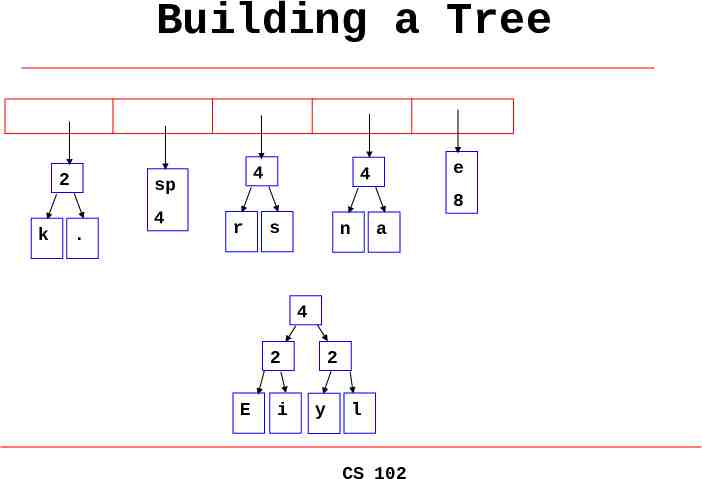
Building a Tree 2 k 4 sp . 4 e 4 8 r s n a 4 2 E i 2 y l CS 102
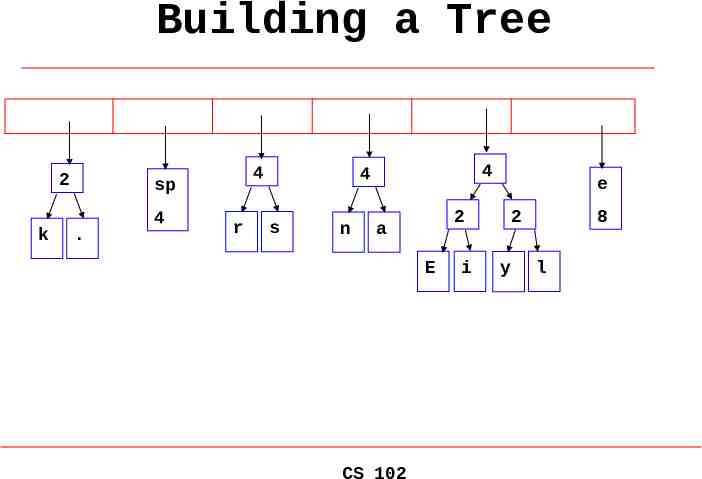
Building a Tree 2 k 4 sp . 4 r 4 4 s n 2 a E CS 102 e i 2 y 8 l
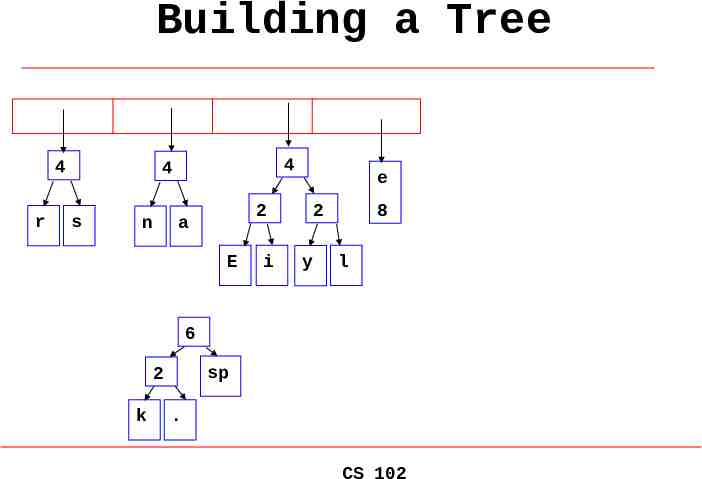
Building a Tree 4 r 4 4 s n e 2 a E i 2 y 8 l 6 sp 2 k . CS 102
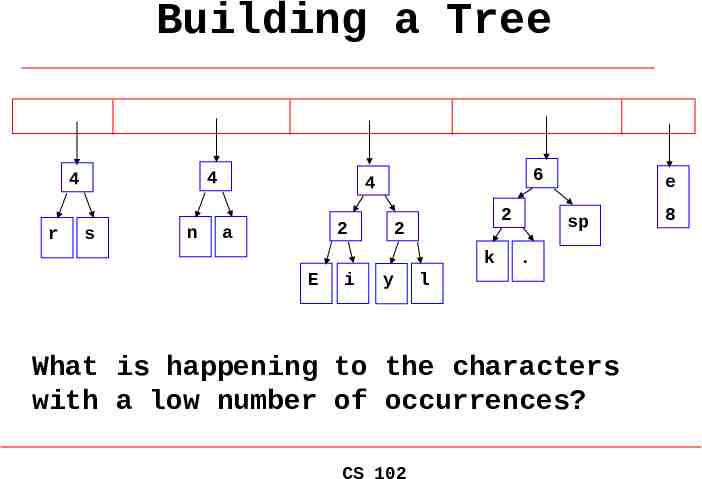
Building a Tree 4 4 r s n 6 4 2 a 2 2 k E i y e sp . l What is happening to the characters with a low number of occurrences? CS 102 8
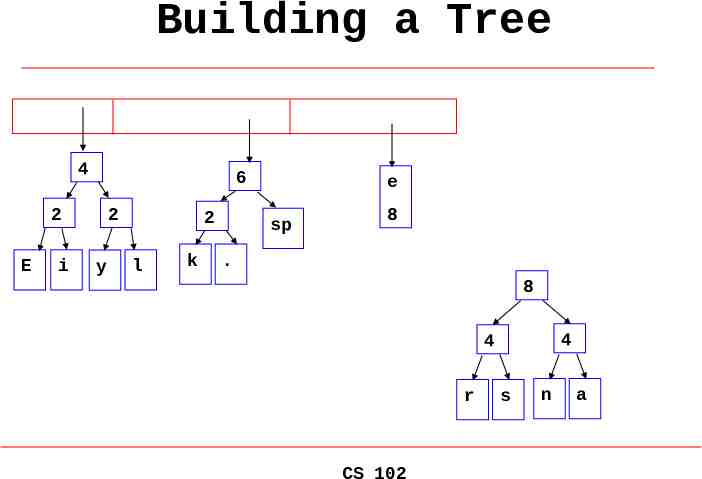
Building a Tree 4 6 2 E i 2 y 2 l k e sp 8 . 8 4 4 r CS 102 s n a
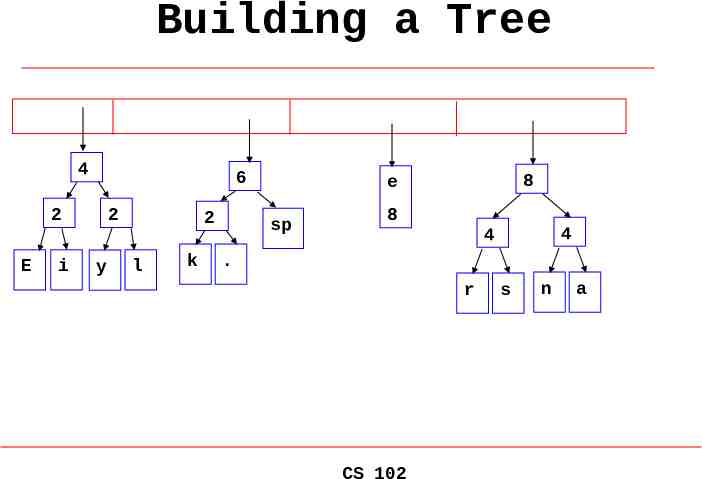
Building a Tree 4 6 2 E i 2 y 2 l k 8 e sp 8 4 4 . r CS 102 s n a
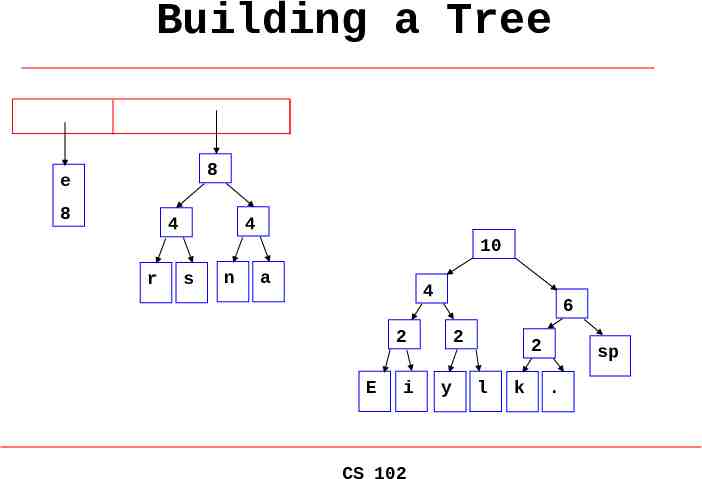
Building a Tree 8 e 8 4 4 10 r s n a 4 6 2 E i CS 102 2 y 2 l k sp .
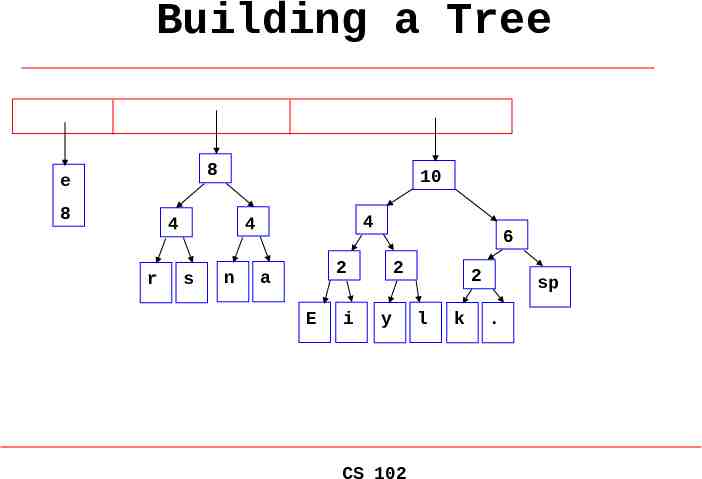
Building a Tree 8 e 8 10 r 4 4 4 s n 6 2 a E i 2 y CS 102 2 l k sp .
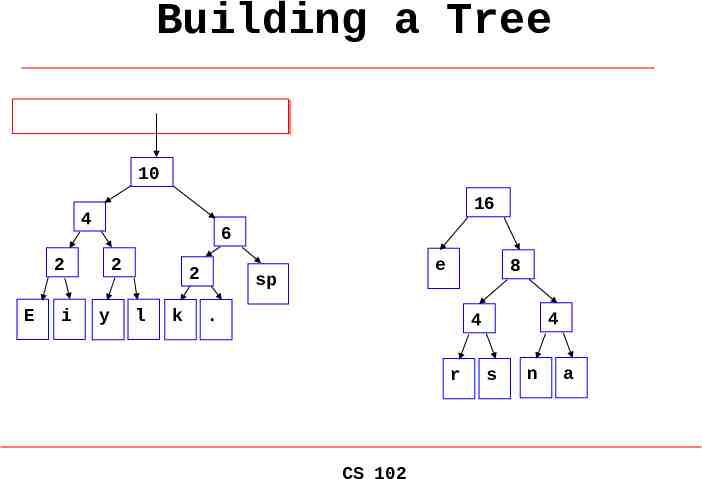
Building a Tree 10 16 4 6 2 E i 2 y 2 l k e sp 8 . 4 4 r CS 102 s n a
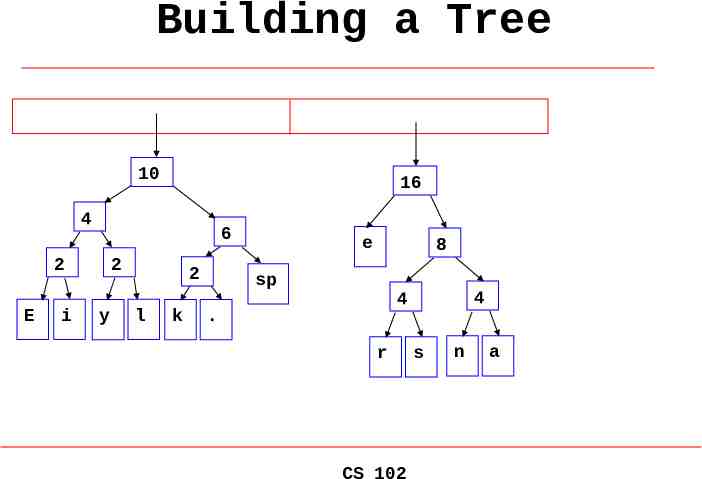
Building a Tree 10 16 4 6 2 E i 2 y 2 l k e 8 sp 4 4 . r CS 102 s n a
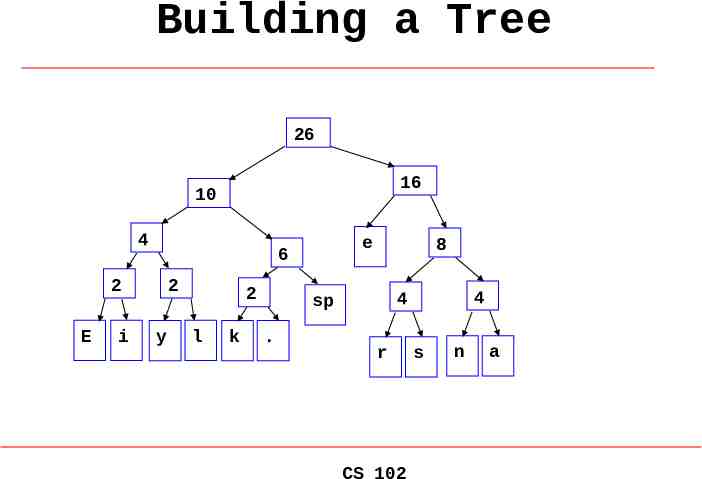
Building a Tree 26 16 10 4 2 E i e 6 2 y 2 l k 8 . 4 4 sp r CS 102 s n a
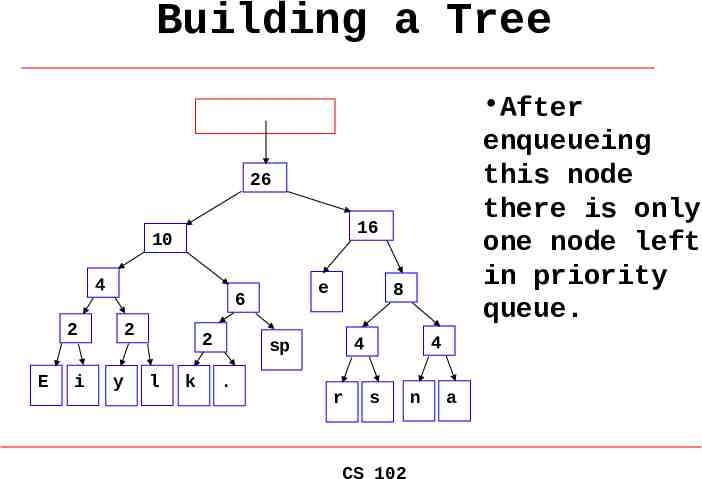
Building a Tree After enqueueing this node there is only one node left in priority queue. 26 16 10 4 2 E i e 6 2 y 2 l k 8 . 4 4 sp r s CS 102 n a
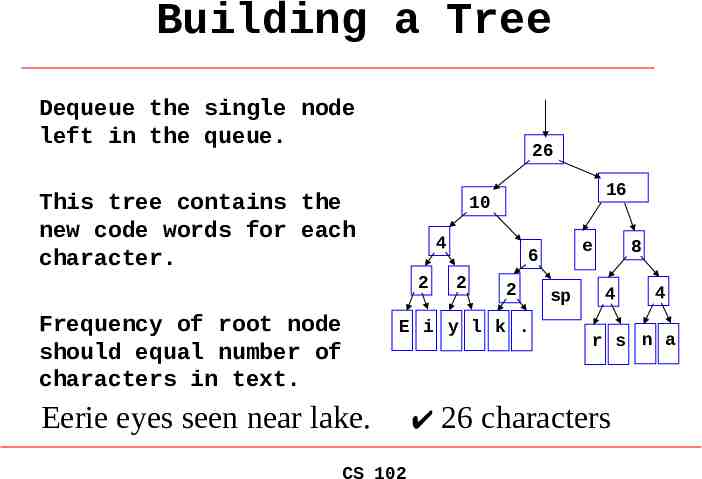
Building a Tree Dequeue the single node left in the queue. 26 This tree contains the new code words for each character. 4 2 Frequency of root node should equal number of characters in text. 16 10 2 2 E i y l k . Eerie eyes seen near lake. CS 102 e 6 sp 8 4 4 r s n a 26 characters
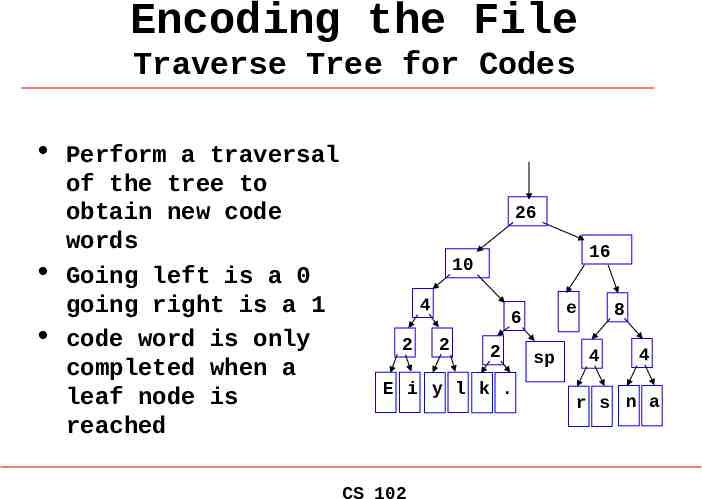
Encoding the File Traverse Tree for Codes Perform a traversal of the tree to obtain new code words Going left is a 0 going right is a 1 code word is only completed when a leaf node is reached 26 16 10 4 2 2 2 E i y l k . CS 102 e 6 sp 8 4 4 r s n a
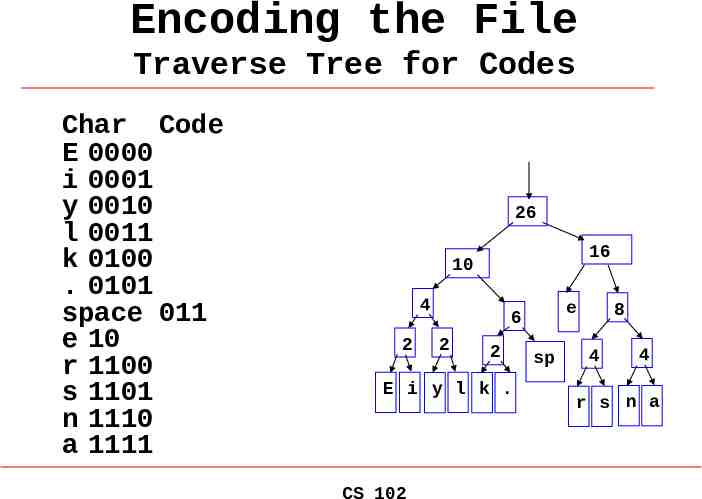
Encoding the File Traverse Tree for Codes Char Code E 0000 i 0001 y 0010 l 0011 k 0100 . 0101 space 011 e 10 r 1100 s 1101 n 1110 a 1111 26 16 10 4 2 2 2 E i y l k . CS 102 e 6 sp 8 4 4 r s n a
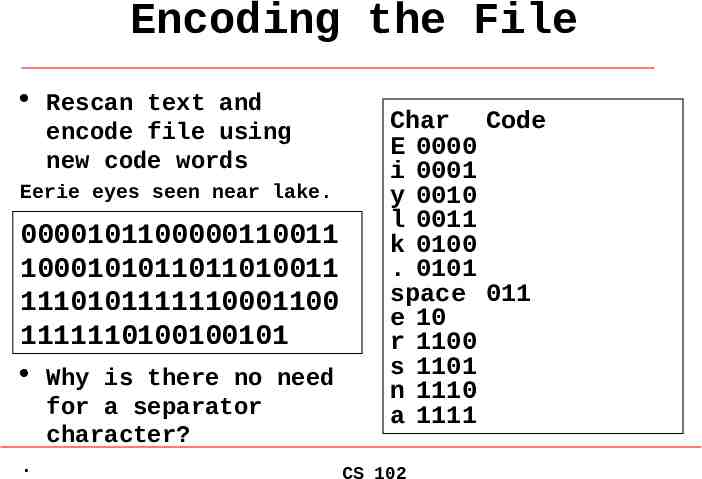
Encoding the File Rescan text and encode file using new code words Eerie eyes seen near lake. 0000101100000110011 1000101011011010011 1110101111110001100 1111110100100101 Why is there no need for a separator character? . Char Code E 0000 i 0001 y 0010 l 0011 k 0100 . 0101 space 011 e 10 r 1100 s 1101 n 1110 a 1111 CS 102
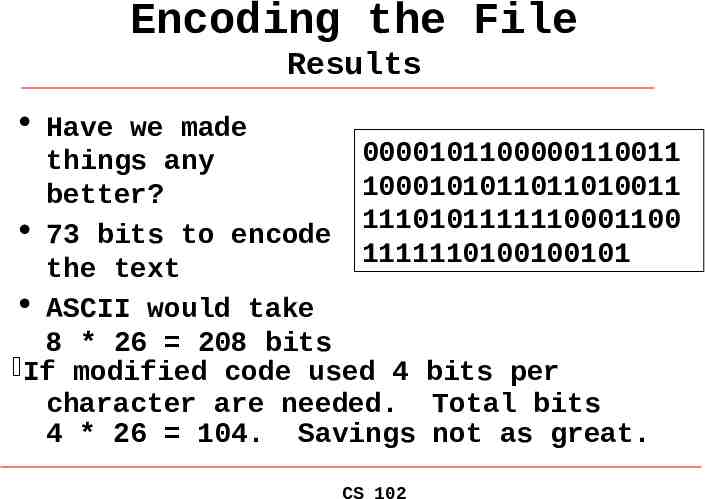
Encoding the File Results Have we made 0000101100000110011 things any 1000101011011010011 better? 73 bits to encode 1110101111110001100 1111110100100101 the text ASCII would take 8 * 26 208 bits If modified code used 4 bits per character are needed. Total bits 4 * 26 104. Savings not as great. CS 102
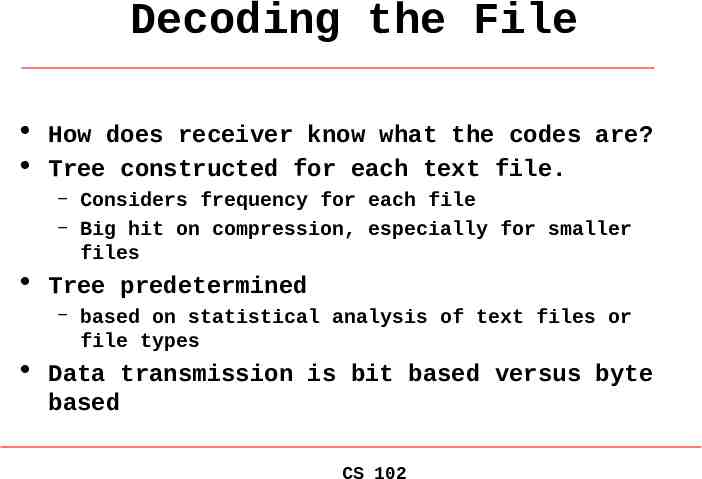
Decoding the File How does receiver know what the codes are? Tree constructed for each text file. – Considers frequency for each file – Big hit on compression, especially for smaller files Tree predetermined – based on statistical analysis of text files or file types Data transmission is bit based versus byte based CS 102
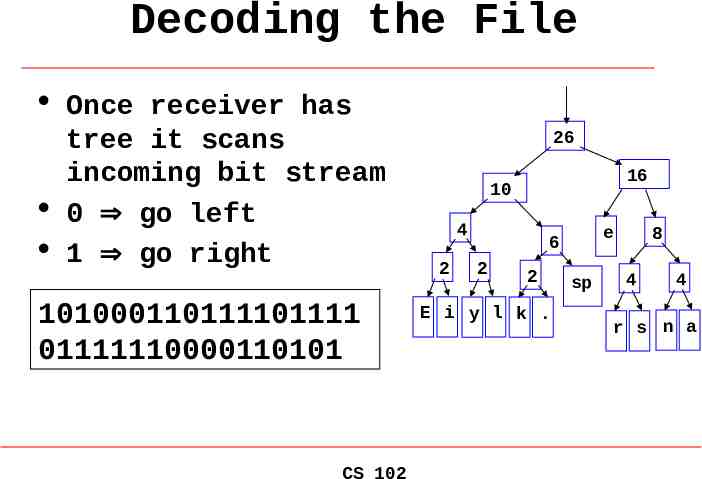
Decoding the File Once receiver has tree it scans incoming bit stream 0 go left 1 go right 101000110111101111 01111110000110101 CS 102 26 16 10 4 2 e 6 2 2 E i y l k . sp 8 4 4 r s n a
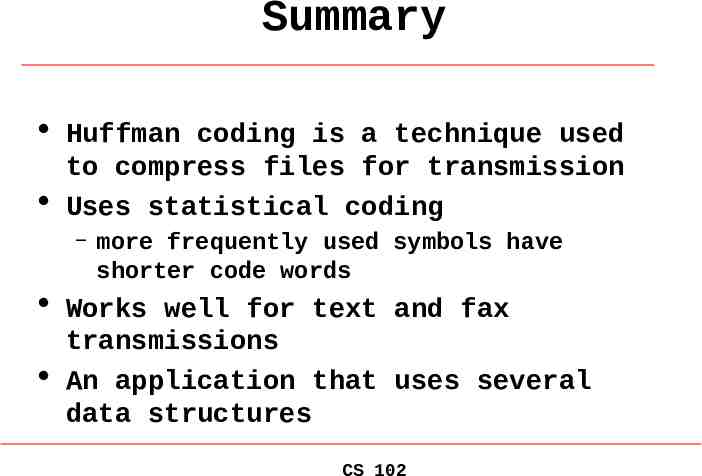
Summary Huffman coding is a technique used to compress files for transmission Uses statistical coding – more frequently used symbols have shorter code words Works well for text and fax transmissions An application that uses several data structures CS 102