JavaScript Overview
69 Slides1.12 MB
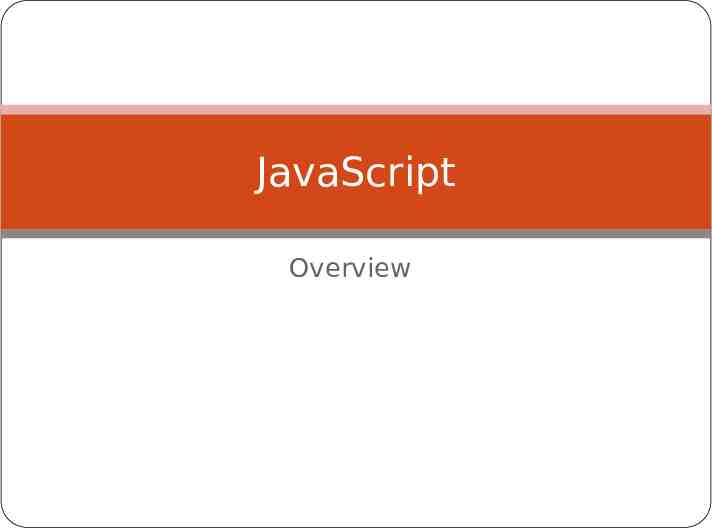
JavaScript Overview
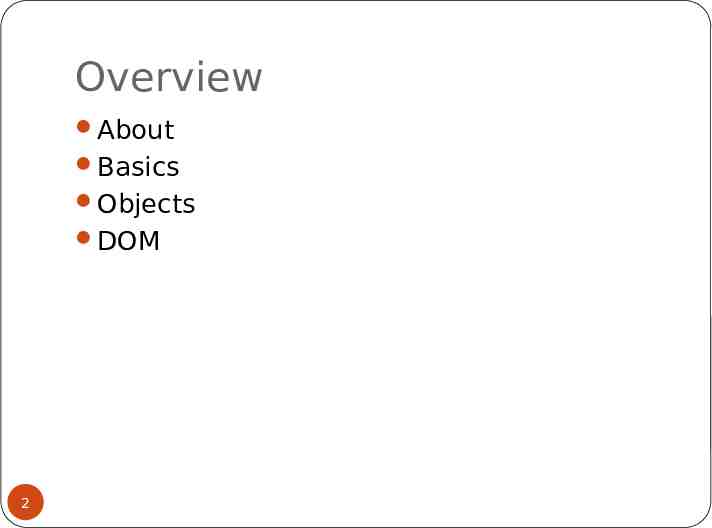
Overview About Basics Objects DOM 2
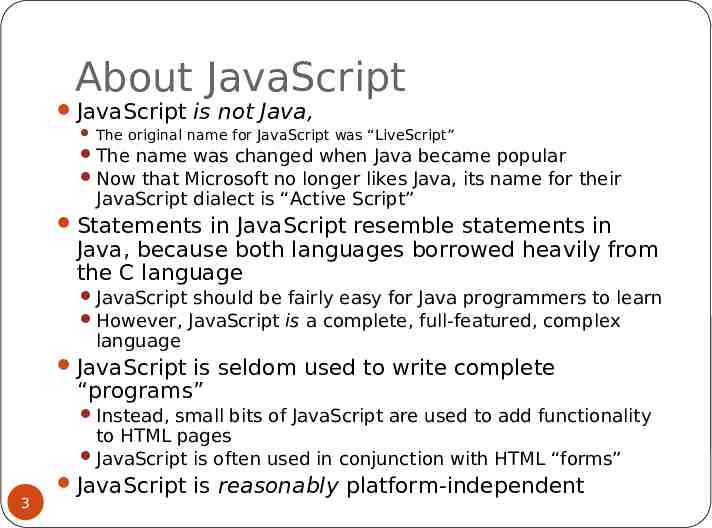
About JavaScript JavaScript is not Java, The original name for JavaScript was “LiveScript” The name was changed when Java became popular Now that Microsoft no longer likes Java, its name for their JavaScript dialect is “Active Script” Statements in JavaScript resemble statements in Java, because both languages borrowed heavily from the C language JavaScript should be fairly easy for Java programmers to learn However, JavaScript is a complete, full-featured, complex language JavaScript is seldom used to write complete “programs” Instead, small bits of JavaScript are used to add functionality to HTML pages JavaScript is often used in conjunction with HTML “forms” 3 JavaScript is reasonably platform-independent
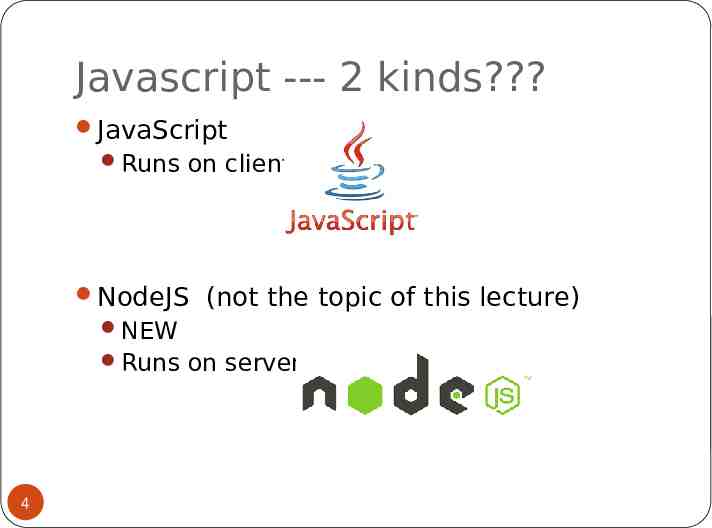
Javascript --- 2 kinds? JavaScript Runs on client NodeJS (not the topic of this lecture) NEW Runs on server 4
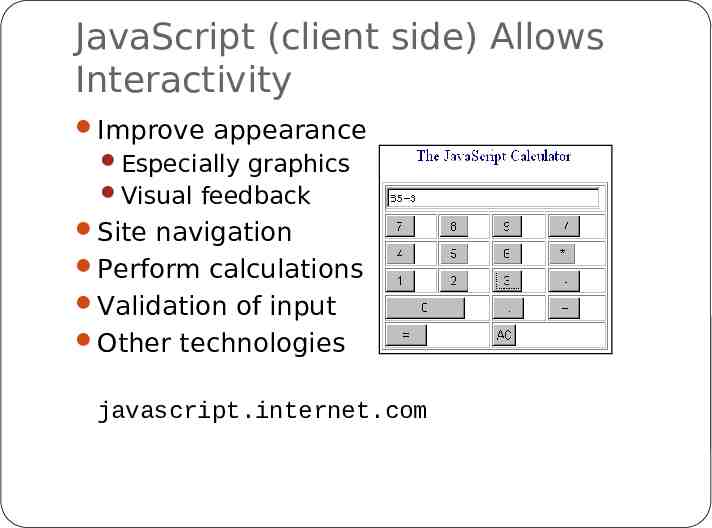
JavaScript (client side) Allows Interactivity Improve appearance Especially graphics Visual feedback Site navigation Perform calculations Validation of input Other technologies javascript.internet.com
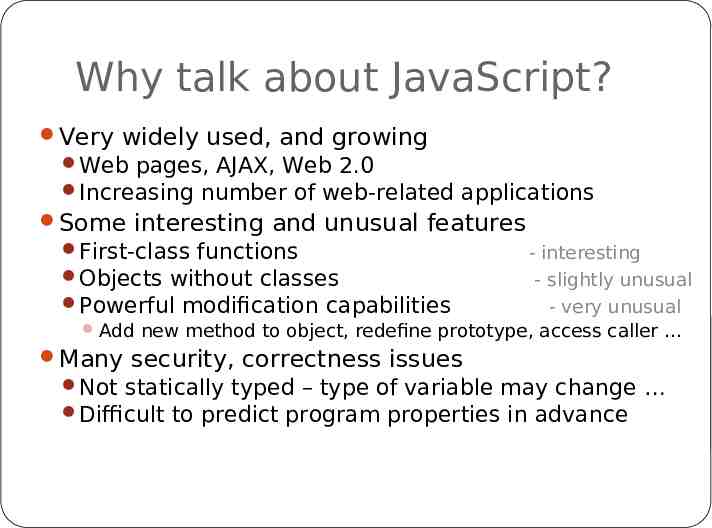
Why talk about JavaScript? Very widely used, and growing Web pages, AJAX, Web 2.0 Increasing number of web-related applications Some interesting and unusual features First-class functions - interesting Objects without classes - slightly unusual Powerful modification capabilities - very unusual Add new method to object, redefine prototype, access caller Many security, correctness issues Not statically typed – type of variable may change Difficult to predict program properties in advance
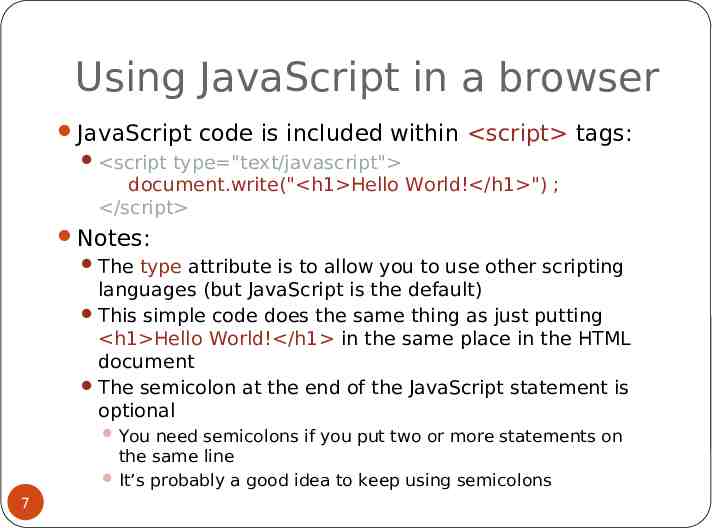
Using JavaScript in a browser JavaScript code is included within script tags: script type "text/javascript" document.write(" h1 Hello World! /h1 ") ; /script Notes: The type attribute is to allow you to use other scripting languages (but JavaScript is the default) This simple code does the same thing as just putting h1 Hello World! /h1 in the same place in the HTML document The semicolon at the end of the JavaScript statement is optional You need semicolons if you put two or more statements on the same line It’s probably a good idea to keep using semicolons 7
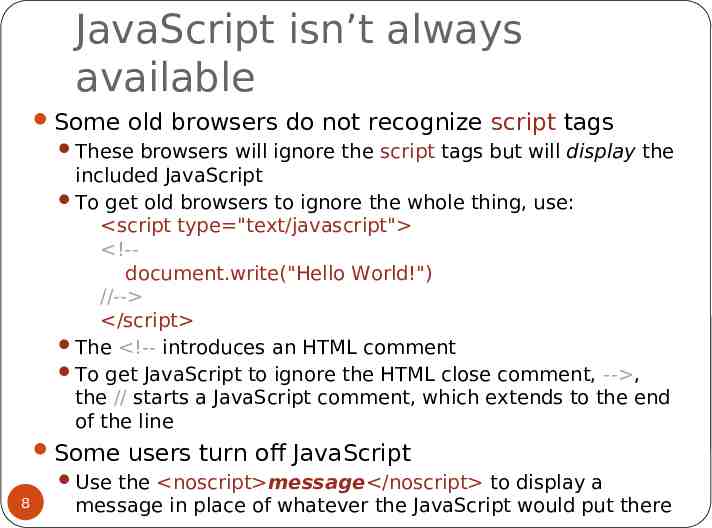
JavaScript isn’t always available Some old browsers do not recognize script tags These browsers will ignore the script tags but will display the included JavaScript To get old browsers to ignore the whole thing, use: script type "text/javascript" !-document.write("Hello World!") //-- /script The !-- introduces an HTML comment To get JavaScript to ignore the HTML close comment, -- , the // starts a JavaScript comment, which extends to the end of the line Some users turn off JavaScript Use the noscript message /noscript to display a 8 message in place of whatever the JavaScript would put there
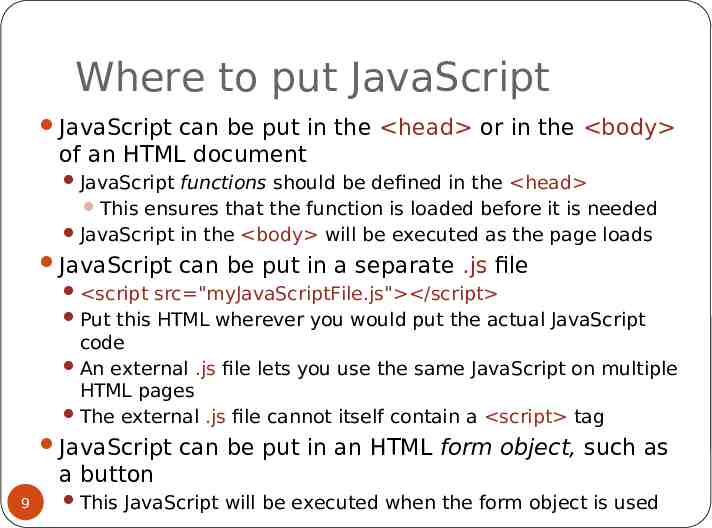
Where to put JavaScript JavaScript can be put in the head or in the body of an HTML document JavaScript functions should be defined in the head This ensures that the function is loaded before it is needed JavaScript in the body will be executed as the page loads JavaScript can be put in a separate .js file script src "myJavaScriptFile.js" /script Put this HTML wherever you would put the actual JavaScript code An external .js file lets you use the same JavaScript on multiple HTML pages The external .js file cannot itself contain a script tag JavaScript can be put in an HTML form object, such as a button 9 This JavaScript will be executed when the form object is used
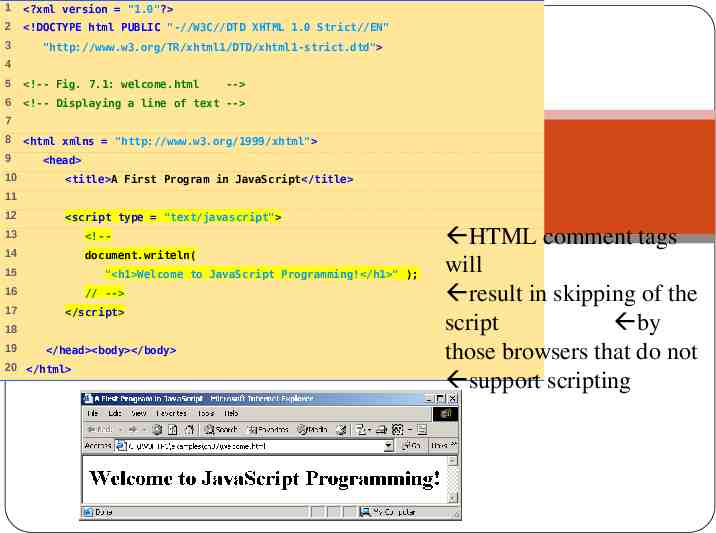
1 ?xml version "1.0"? 2 !DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" 3 "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd" 4 5 !-- Fig. 7.1: welcome.html 6 !-- Displaying a line of text -- -- 7 8 9 10 welcome.html (1 of 1) html xmlns "http://www.w3.org/1999/xhtml" head title A First Program in JavaScript /title 11 12 script type "text/javascript" 13 !-- 14 document.writeln( 15 " h1 Welcome to JavaScript Programming! /h1 " ); 16 // -- 17 /script 18 19 /head body /body 20 /html HTML comment tags will result in skipping of the script by those browsers that do not support scripting
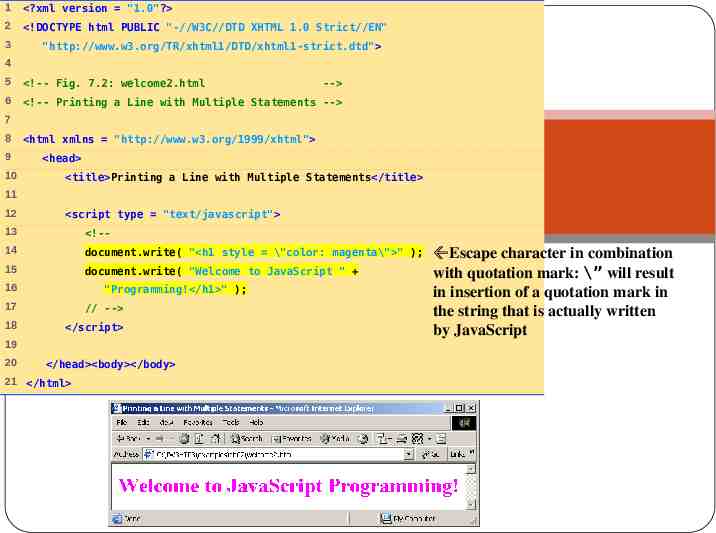
1 ?xml version "1.0"? 2 !DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" 3 "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd" 4 5 !-- Fig. 7.2: welcome2.html 6 !-- Printing a Line with Multiple Statements -- -- 7 8 9 10 welcome2.html (1 of 1) html xmlns "http://www.w3.org/1999/xhtml" head title Printing a Line with Multiple Statements /title 11 12 script type "text/javascript" 13 !-- 14 document.write( " h1 style \"color: magenta\" " ); 15 document.write( "Welcome to JavaScript " 16 "Programming! /h1 " ); 17 // -- 18 /script 19 20 /head body /body 21 /html Escape character in combination with quotation mark: \” will result in insertion of a quotation mark in the string that is actually written by JavaScript
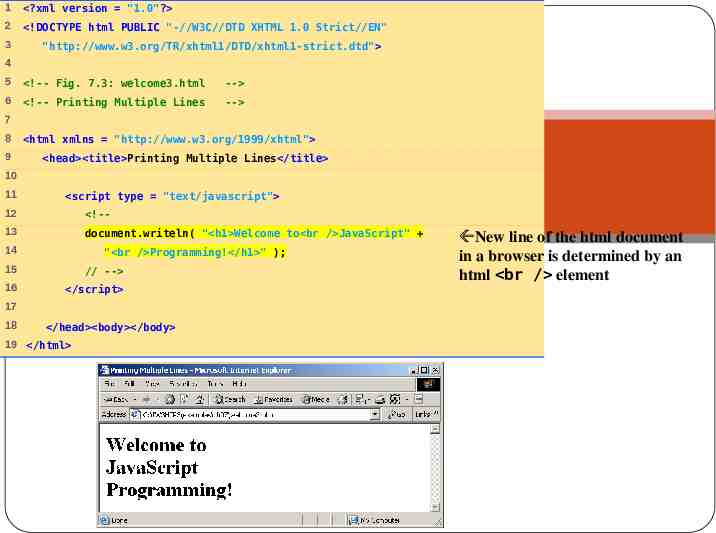
1 ?xml version "1.0"? 2 !DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" 3 "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd" 4 5 !-- Fig. 7.3: welcome3.html -- 6 !-- Printing Multiple Lines -- 7 8 9 head title Printing Multiple Lines /title 10 11 welcome3.html 1 of 1 html xmlns "http://www.w3.org/1999/xhtml" script type "text/javascript" 12 !-- 13 document.writeln( " h1 Welcome to br / JavaScript" 14 " br / Programming! /h1 " ); 15 // -- 16 /script 17 18 /head body /body 19 /html New line of the html document in a browser is determined by an html br / element
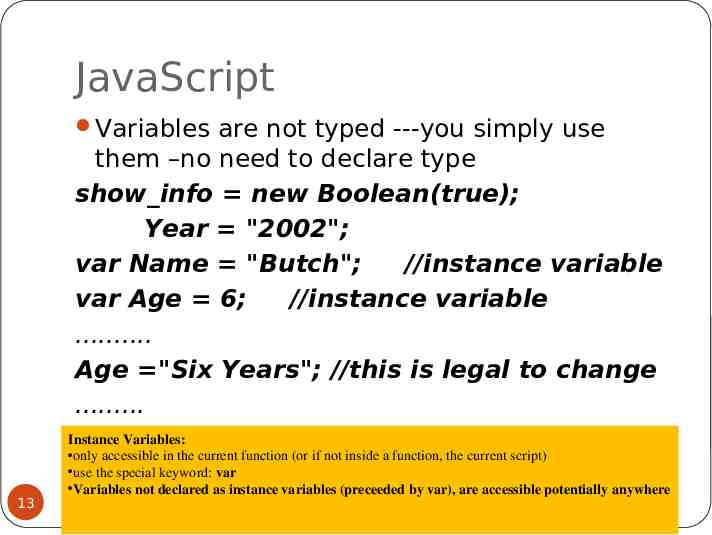
JavaScript Variables are not typed ---you simply use them –no need to declare type show info new Boolean(true); Year "2002"; var Name "Butch"; //instance variable var Age 6; //instance variable . Age "Six Years"; //this is legal to change . 13 Instance Variables: only accessible in the current function (or if not inside a function, the current script) use the special keyword: var Variables not declared as instance variables (preceeded by var), are accessible potentially anywhere
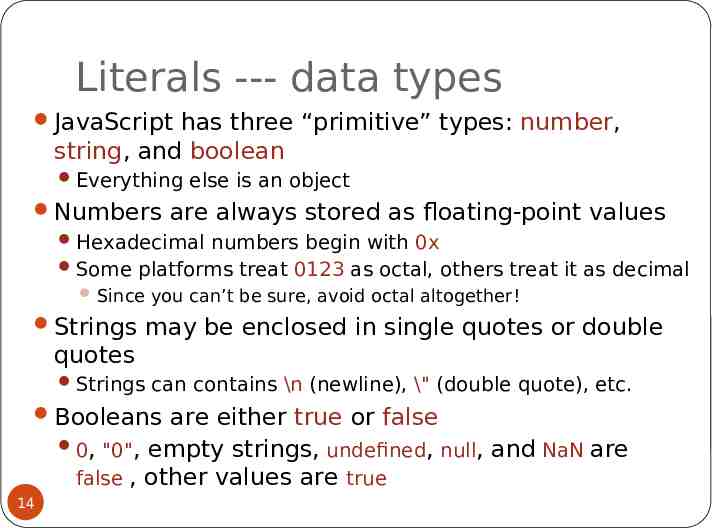
Literals --- data types JavaScript has three “primitive” types: number, string, and boolean Everything else is an object Numbers are always stored as floating-point values Hexadecimal numbers begin with 0x Some platforms treat 0123 as octal, others treat it as decimal Since you can’t be sure, avoid octal altogether! Strings may be enclosed in single quotes or double quotes Strings can contains \n (newline), \" (double quote), etc. Booleans are either true or false 0, "0", empty strings, undefined, null, and NaN are false , other values are true 14
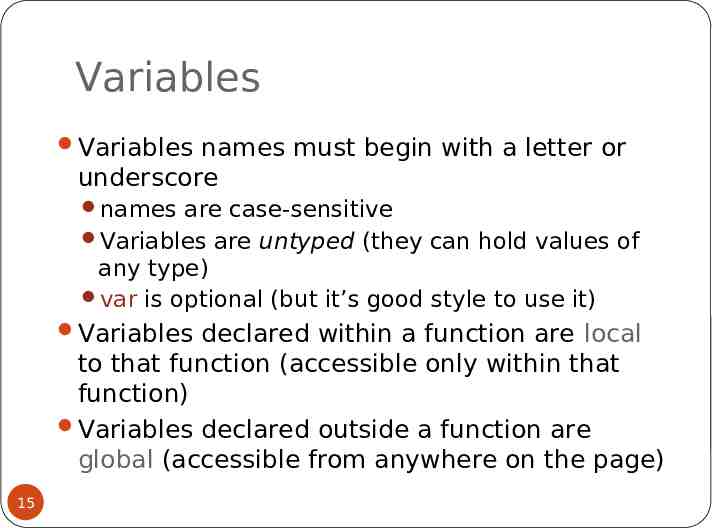
Variables Variables names must begin with a letter or underscore names are case-sensitive Variables are untyped (they can hold values of any type) var is optional (but it’s good style to use it) Variables declared within a function are local to that function (accessible only within that function) Variables declared outside a function are global (accessible from anywhere on the page) 15
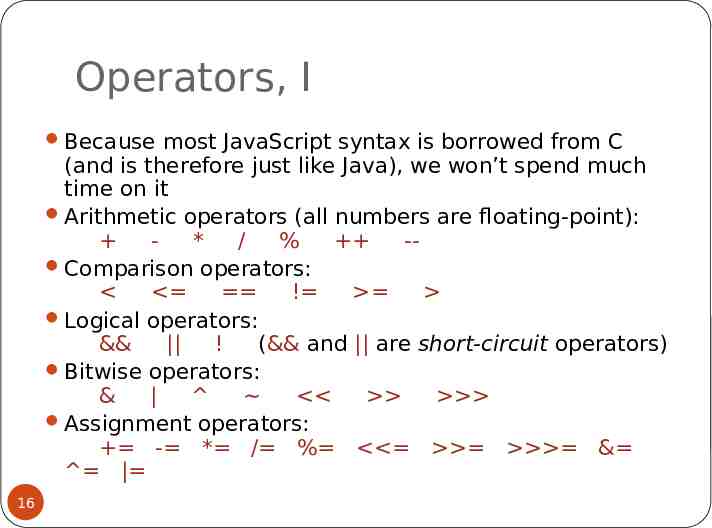
Operators, I Because most JavaScript syntax is borrowed from C (and is therefore just like Java), we won’t spend much time on it Arithmetic operators (all numbers are floating-point): * / % - Comparison operators: ! Logical operators: && ! (&& and are short-circuit operators) Bitwise operators: & Assignment operators: - * / % & 16
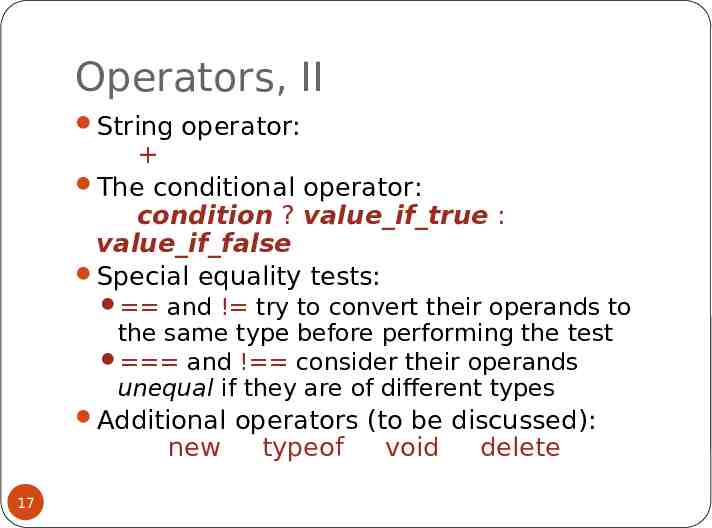
Operators, II String operator: The conditional operator: condition ? value if true : value if false Special equality tests: and ! try to convert their operands to the same type before performing the test and ! consider their operands unequal if they are of different types Additional operators (to be discussed): new 17 typeof void delete
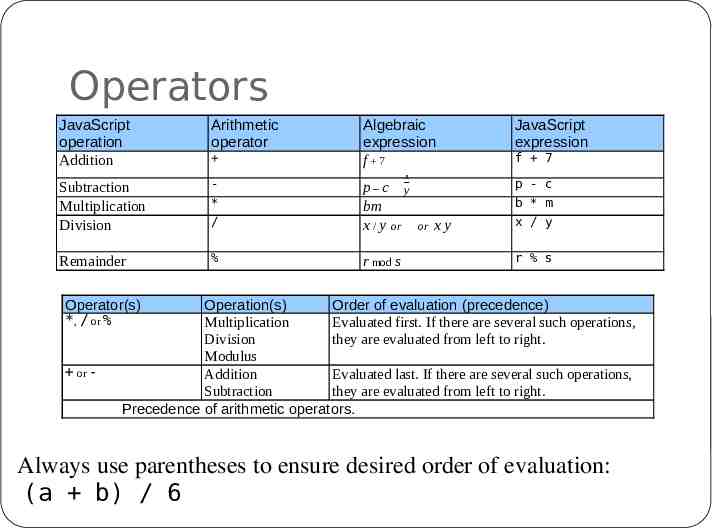
Operators JavaScript operation Addition Arithmetic operator Algebraic expression f 7 JavaScript expression Subtraction Multiplication Division * / p–c bm x / y or p - c b * m x / y Remainder % r mod s x-y or xy f 7 r % s Operator(s) *, / or % or - Operation(s) Order of evaluation (precedence) Multiplication Evaluated first. If there are several such operations, Division they are evaluated from left to right. Modulus Addition Evaluated last. If there are several such operations, Subtraction they are evaluated from left to right. Precedence of arithmetic operators. Always use parentheses to ensure desired order of evaluation: (a b) / 6
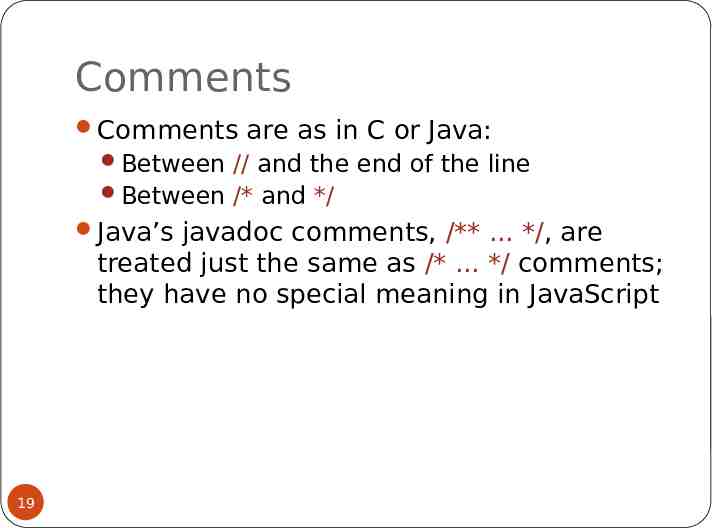
Comments Comments are as in C or Java: Between // and the end of the line Between /* and */ Java’s javadoc comments, /** . */, are treated just the same as /* . */ comments; they have no special meaning in JavaScript 19
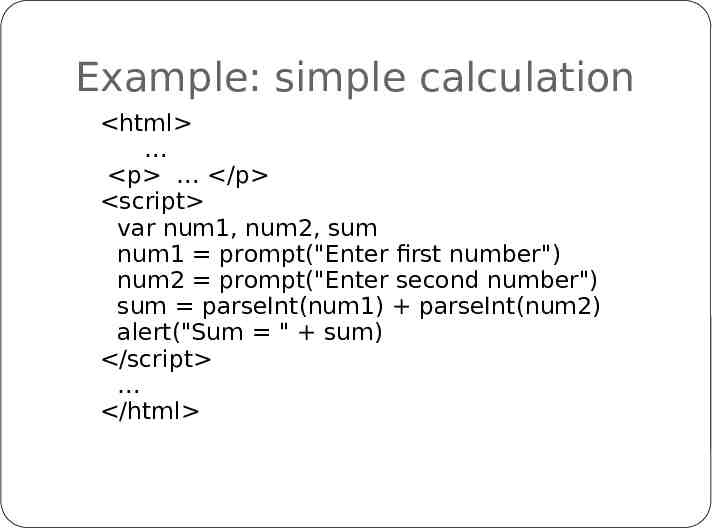
Example: simple calculation html p /p script var num1, num2, sum num1 prompt("Enter first number") num2 prompt("Enter second number") sum parseInt(num1) parseInt(num2) alert("Sum " sum) /script /html
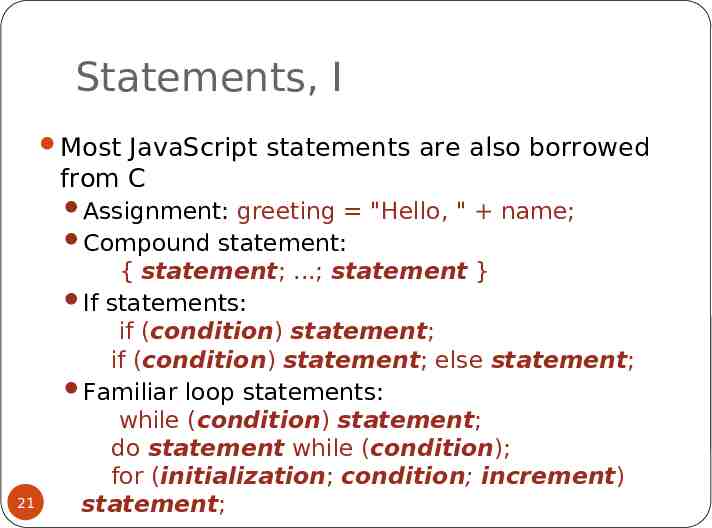
Statements, I Most JavaScript statements are also borrowed from C Assignment: greeting "Hello, " name; Compound statement: 21 { statement; .; statement } If statements: if (condition) statement; if (condition) statement; else statement; Familiar loop statements: while (condition) statement; do statement while (condition); for (initialization; condition; increment) statement;
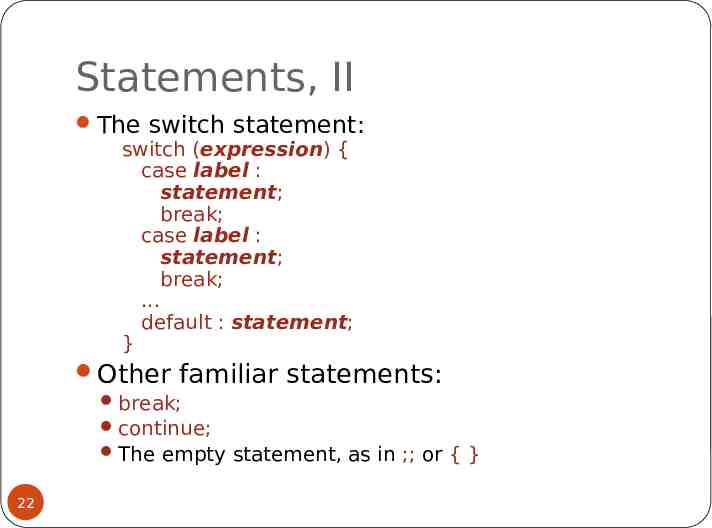
Statements, II The switch statement: switch (expression) { case label : statement; break; case label : statement; break; . default : statement; } Other familiar statements: break; continue; The empty statement, as in ;; or { } 22
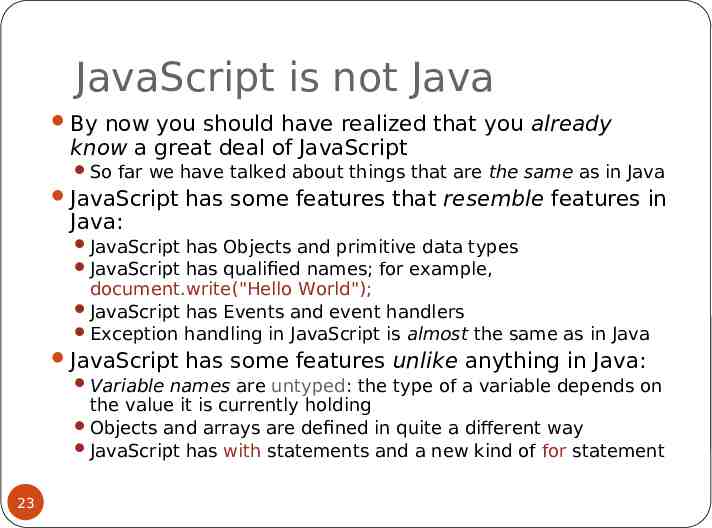
JavaScript is not Java By now you should have realized that you already know a great deal of JavaScript So far we have talked about things that are the same as in Java JavaScript has some features that resemble features in Java: JavaScript has Objects and primitive data types JavaScript has qualified names; for example, document.write("Hello World"); JavaScript has Events and event handlers Exception handling in JavaScript is almost the same as in Java JavaScript has some features unlike anything in Java: Variable names are untyped: the type of a variable depends on the value it is currently holding Objects and arrays are defined in quite a different way JavaScript has with statements and a new kind of for statement 23
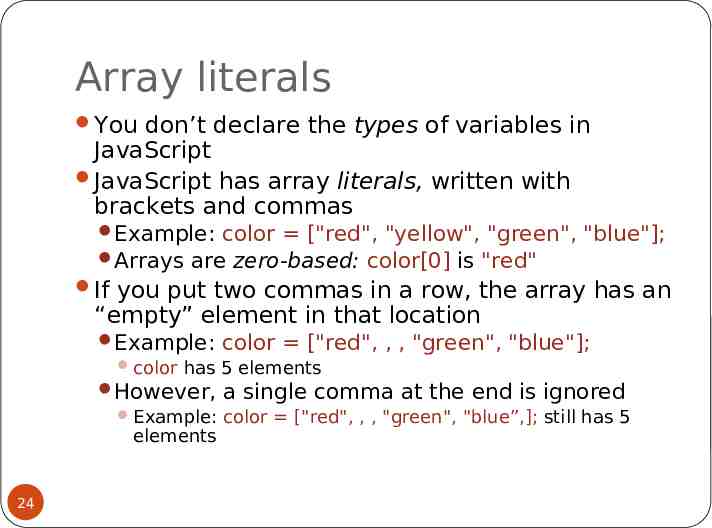
Array literals You don’t declare the types of variables in JavaScript JavaScript has array literals, written with brackets and commas Example: color ["red", "yellow", "green", "blue"]; Arrays are zero-based: color[0] is "red" If you put two commas in a row, the array has an “empty” element in that location Example: color ["red", , , "green", "blue"]; color has 5 elements However, a single comma at the end is ignored Example: color ["red", , , "green", "blue”,]; still has 5 elements 24
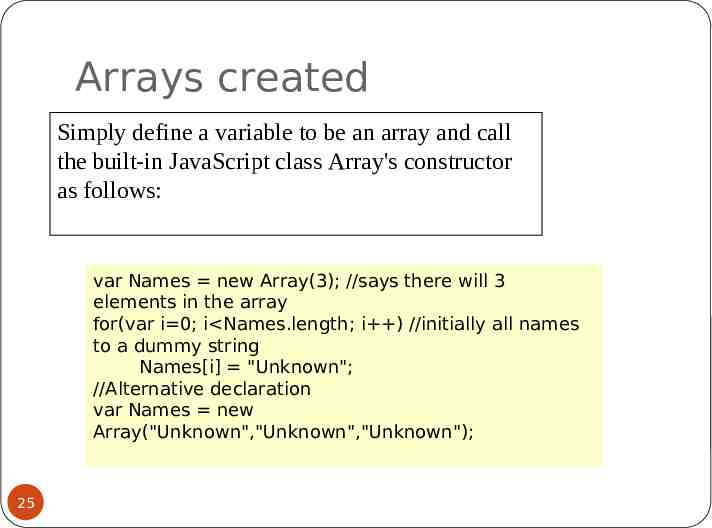
Arrays created Simply define a variable to be an array and call the built-in JavaScript class Array's constructor as follows: var Names new Array(3); //says there will 3 elements in the array for(var i 0; i Names.length; i ) //initially all names to a dummy string Names[i] "Unknown"; //Alternative declaration var Names new Array("Unknown","Unknown","Unknown"); 25
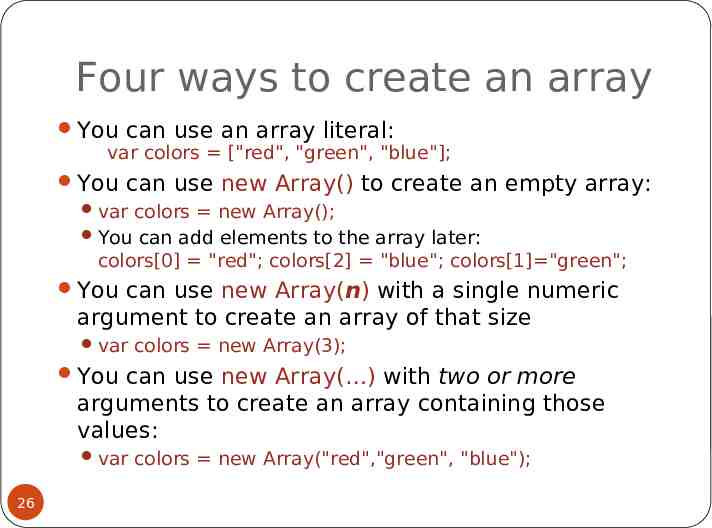
Four ways to create an array You can use an array literal: var colors ["red", "green", "blue"]; You can use new Array() to create an empty array: var colors new Array(); You can add elements to the array later: colors[0] "red"; colors[2] "blue"; colors[1] "green"; You can use new Array(n) with a single numeric argument to create an array of that size var colors new Array(3); You can use new Array( ) with two or more arguments to create an array containing those values: var colors new Array("red","green", "blue"); 26
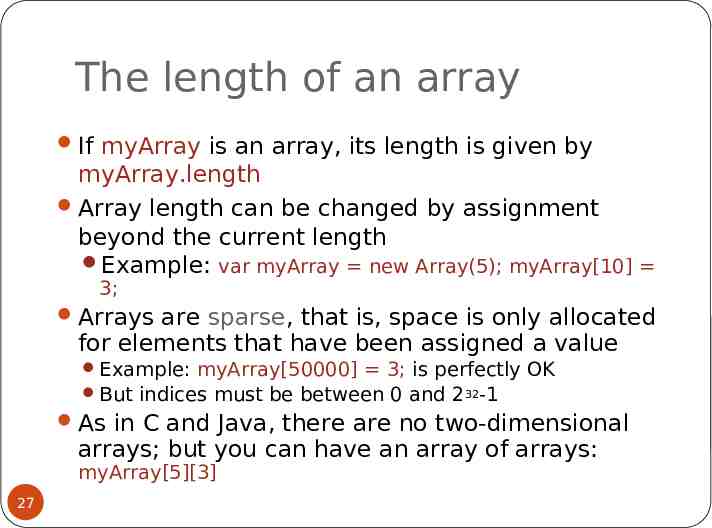
The length of an array If myArray is an array, its length is given by myArray.length Array length can be changed by assignment beyond the current length Example: var myArray new Array(5); myArray[10] 3; Arrays are sparse, that is, space is only allocated for elements that have been assigned a value Example: myArray[50000] 3; is perfectly OK But indices must be between 0 and 232-1 As in C and Java, there are no two-dimensional arrays; but you can have an array of arrays: myArray[5][3] 27
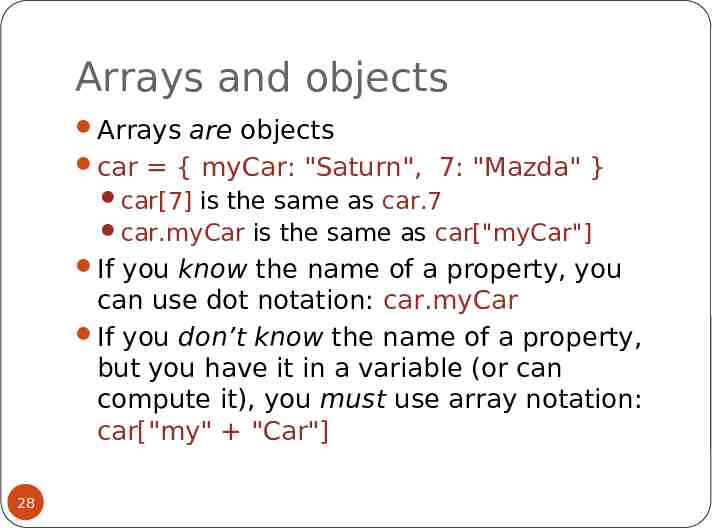
Arrays and objects Arrays are objects car { myCar: "Saturn", 7: "Mazda" } car[7] is the same as car.7 car.myCar is the same as car["myCar"] If you know the name of a property, you can use dot notation: car.myCar If you don’t know the name of a property, but you have it in a variable (or can compute it), you must use array notation: car["my" "Car"] 28
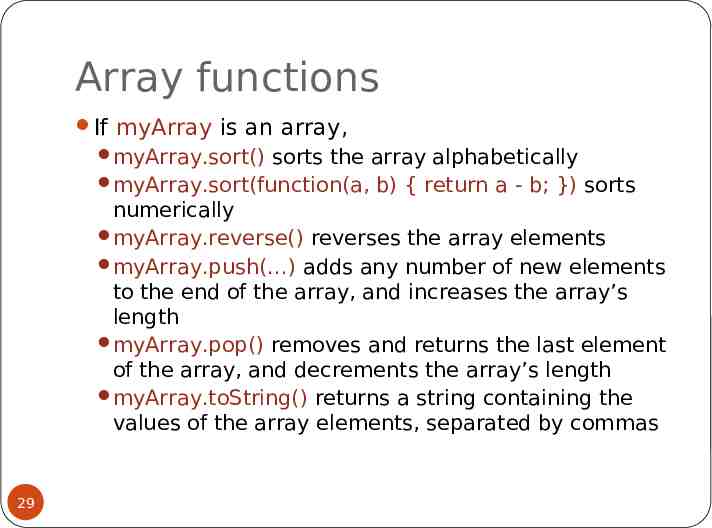
Array functions If myArray is an array, myArray.sort() sorts the array alphabetically myArray.sort(function(a, b) { return a - b; }) sorts numerically myArray.reverse() reverses the array elements myArray.push( ) adds any number of new elements to the end of the array, and increases the array’s length myArray.pop() removes and returns the last element of the array, and decrements the array’s length myArray.toString() returns a string containing the values of the array elements, separated by commas 29
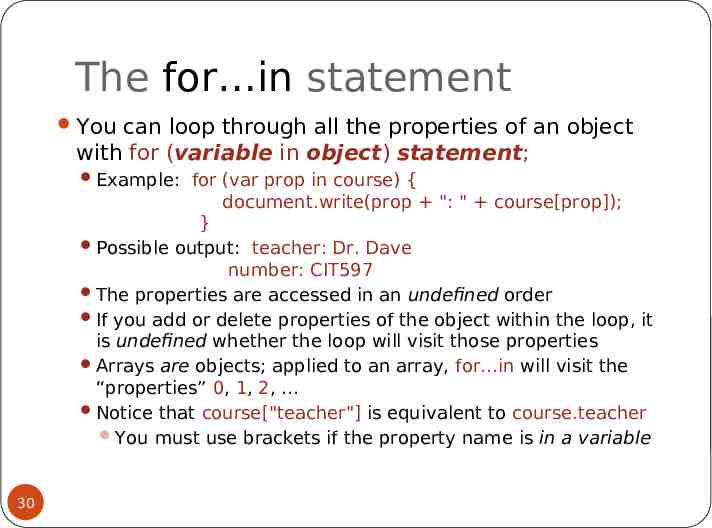
The for in statement You can loop through all the properties of an object with for (variable in object) statement; Example: for (var prop in course) { document.write(prop ": " course[prop]); } Possible output: teacher: Dr. Dave number: CIT597 The properties are accessed in an undefined order If you add or delete properties of the object within the loop, it is undefined whether the loop will visit those properties Arrays are objects; applied to an array, for in will visit the “properties” 0, 1, 2, Notice that course["teacher"] is equivalent to course.teacher You must use brackets if the property name is in a variable 30
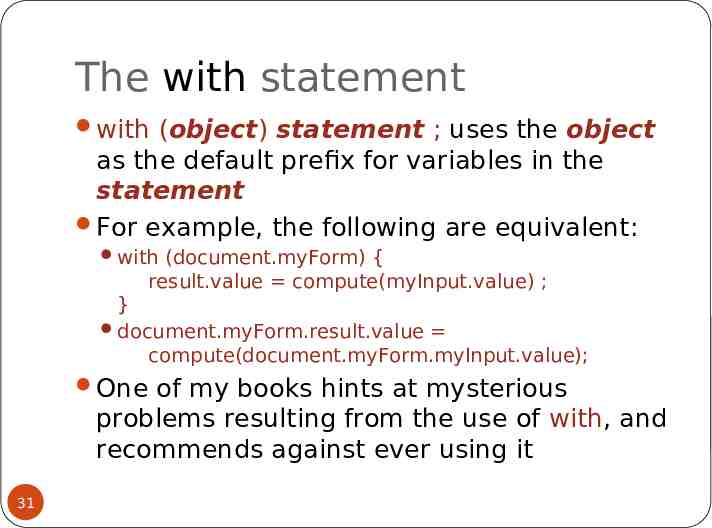
The with statement with (object) statement ; uses the object as the default prefix for variables in the statement For example, the following are equivalent: with (document.myForm) { result.value compute(myInput.value) ; } document.myForm.result.value compute(document.myForm.myInput.value); One of my books hints at mysterious problems resulting from the use of with, and recommends against ever using it 31
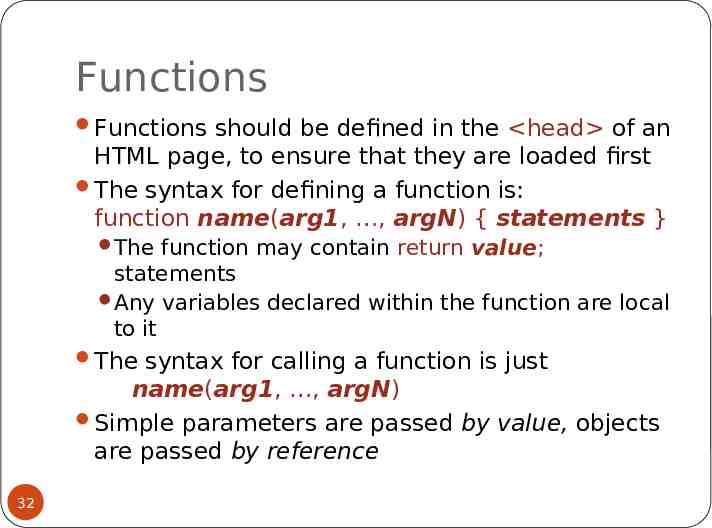
Functions Functions should be defined in the head of an HTML page, to ensure that they are loaded first The syntax for defining a function is: function name(arg1, , argN) { statements } The function may contain return value; statements Any variables declared within the function are local to it The syntax for calling a function is just name(arg1, , argN) Simple parameters are passed by value, objects are passed by reference 32
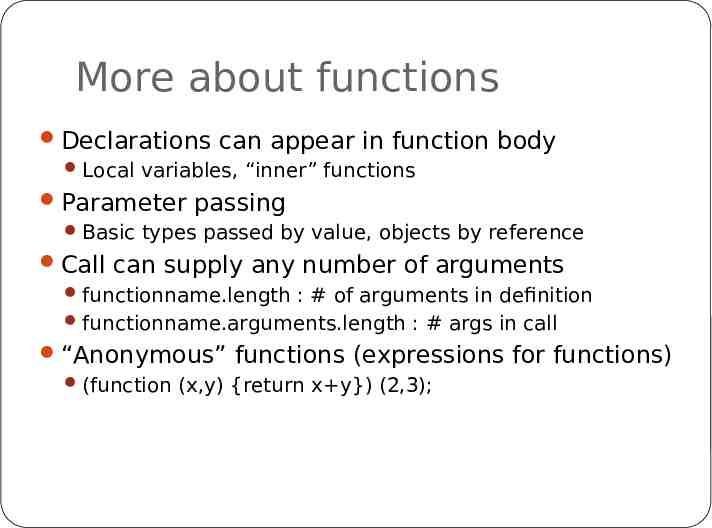
More about functions Declarations can appear in function body Local variables, “inner” functions Parameter passing Basic types passed by value, objects by reference Call can supply any number of arguments functionname.length : # of arguments in definition functionname.arguments.length : # args in call “Anonymous” functions (expressions for functions) (function (x,y) {return x y}) (2,3);
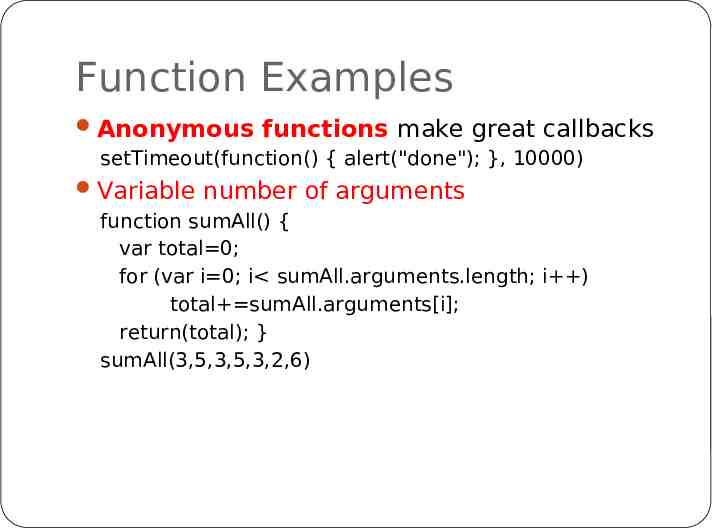
Function Examples Anonymous functions make great callbacks setTimeout(function() { alert("done"); }, 10000) Variable number of arguments function sumAll() { var total 0; for (var i 0; i sumAll.arguments.length; i ) total sumAll.arguments[i]; return(total); } sumAll(3,5,3,5,3,2,6)
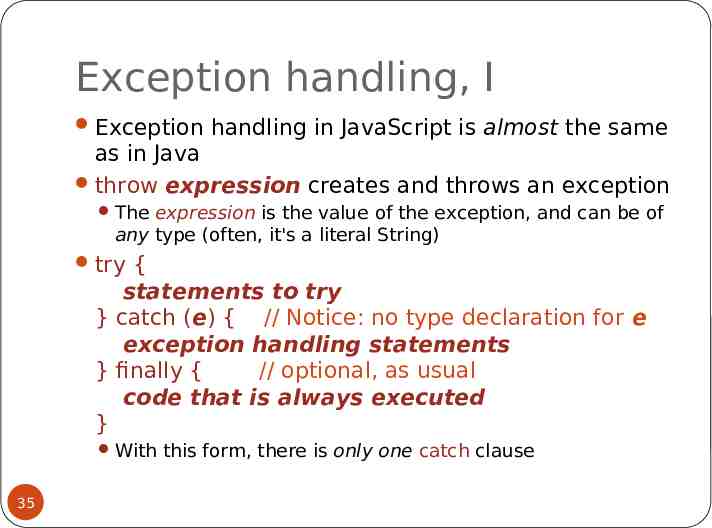
Exception handling, I Exception handling in JavaScript is almost the same as in Java throw expression creates and throws an exception The expression is the value of the exception, and can be of any type (often, it's a literal String) try { statements to try } catch (e) { // Notice: no type declaration for e exception handling statements } finally { // optional, as usual code that is always executed } With this form, there is only one catch clause 35
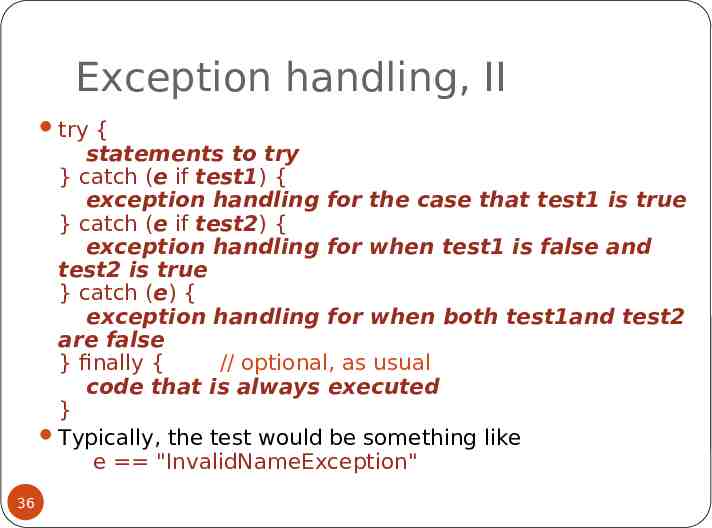
Exception handling, II try { statements to try } catch (e if test1) { exception handling for the case that test1 is true } catch (e if test2) { exception handling for when test1 is false and test2 is true } catch (e) { exception handling for when both test1and test2 are false } finally { // optional, as usual code that is always executed } Typically, the test would be something like e "InvalidNameException" 36
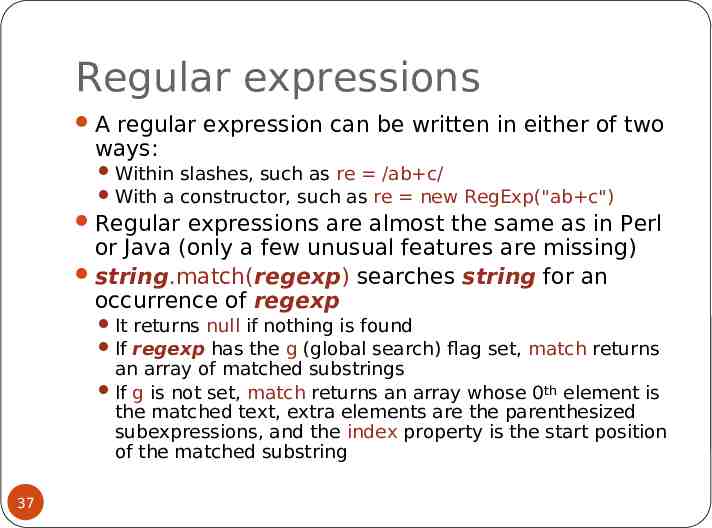
Regular expressions A regular expression can be written in either of two ways: Within slashes, such as re /ab c/ With a constructor, such as re new RegExp("ab c") Regular expressions are almost the same as in Perl or Java (only a few unusual features are missing) string.match(regexp) searches string for an occurrence of regexp It returns null if nothing is found If regexp has the g (global search) flag set, match returns an array of matched substrings If g is not set, match returns an array whose 0 th element is the matched text, extra elements are the parenthesized subexpressions, and the index property is the start position of the matched substring 37
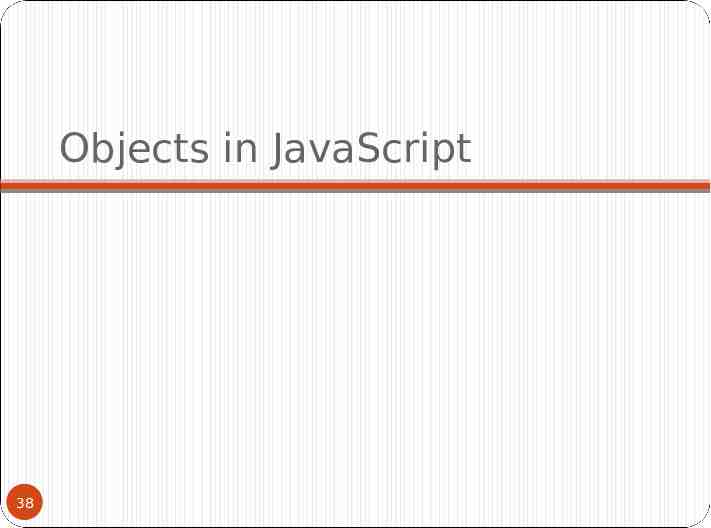
Objects in JavaScript 38
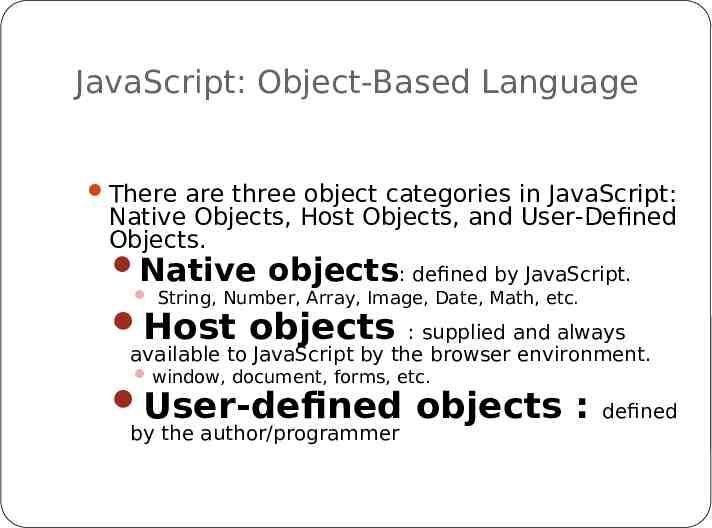
JavaScript: Object-Based Language There are three object categories in JavaScript: Native Objects, Host Objects, and User-Defined Objects. Native objects: defined by JavaScript. String, Number, Array, Image, Date, Math, etc. Host objects : supplied and always available to JavaScript by the browser environment. window, document, forms, etc. User-defined objects : by the author/programmer defined
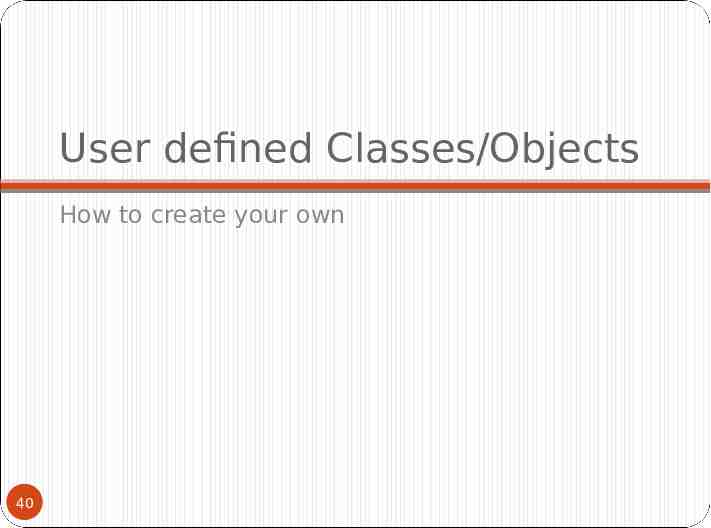
User defined Classes/Objects How to create your own 40
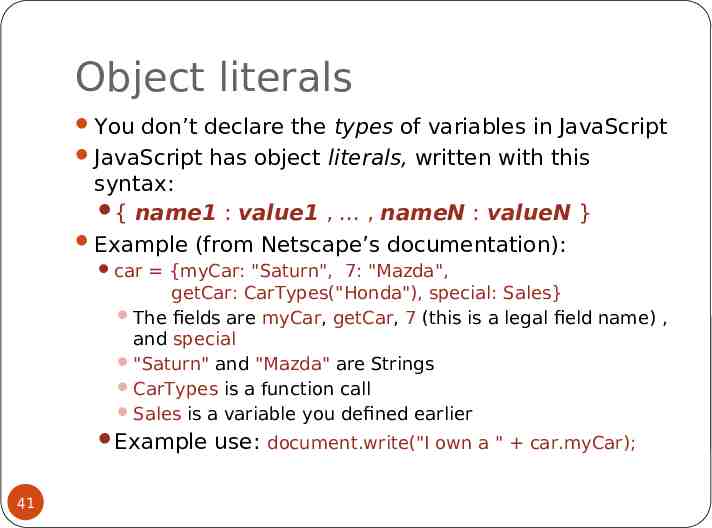
Object literals You don’t declare the types of variables in JavaScript JavaScript has object literals, written with this syntax: { name1 : value1 , . , nameN : valueN } Example (from Netscape’s documentation): car {myCar: "Saturn", 7: "Mazda", getCar: CarTypes("Honda"), special: Sales} The fields are myCar, getCar, 7 (this is a legal field name) , and special "Saturn" and "Mazda" are Strings CarTypes is a function call Sales is a variable you defined earlier Example use: document.write("I own a " car.myCar); 41
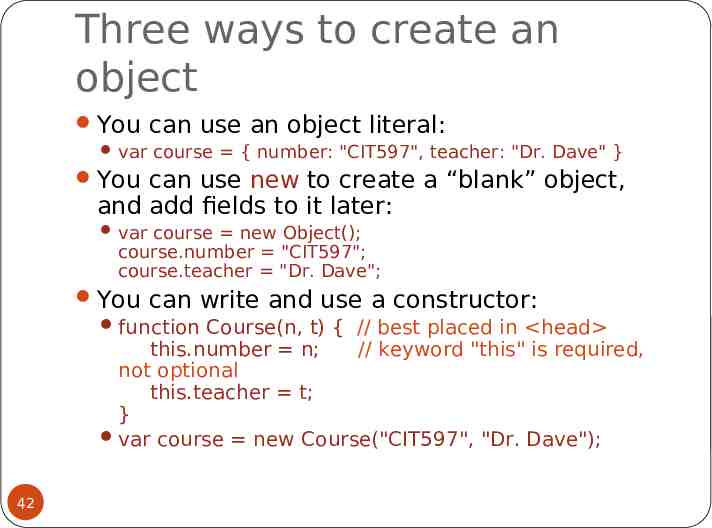
Three ways to create an object You can use an object literal: var course { number: "CIT597", teacher: "Dr. Dave" } You can use new to create a “blank” object, and add fields to it later: var course new Object(); course.number "CIT597"; course.teacher "Dr. Dave"; You can write and use a constructor: function Course(n, t) { // best placed in head this.number n; // keyword "this" is required, not optional this.teacher t; } var course new Course("CIT597", "Dr. Dave"); 42
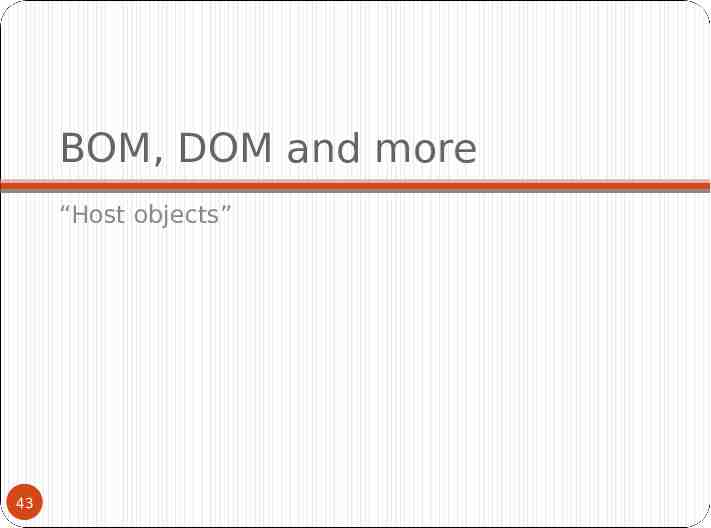
BOM, DOM and more “Host objects” 43
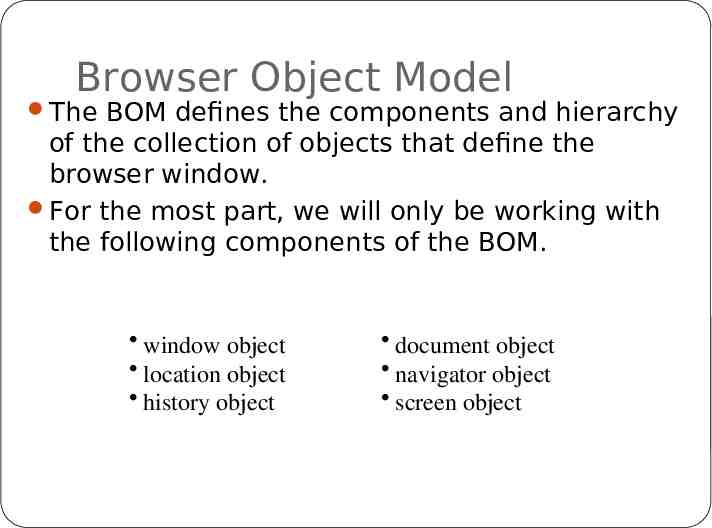
Browser Object Model The BOM defines the components and hierarchy of the collection of objects that define the browser window. For the most part, we will only be working with the following components of the BOM. window object location object history object document object navigator object screen object
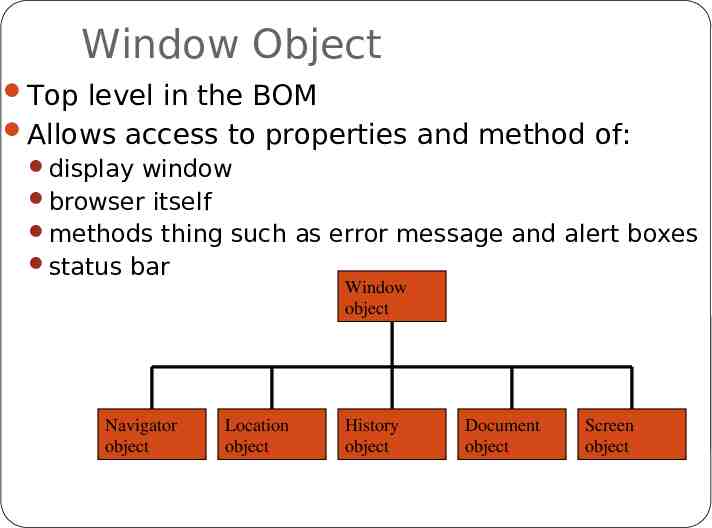
Window Object Top level in the BOM Allows access to properties and method of: display window browser itself methods thing such as error message and alert boxes status bar Window object Navigator object Location object History object Document object Screen object
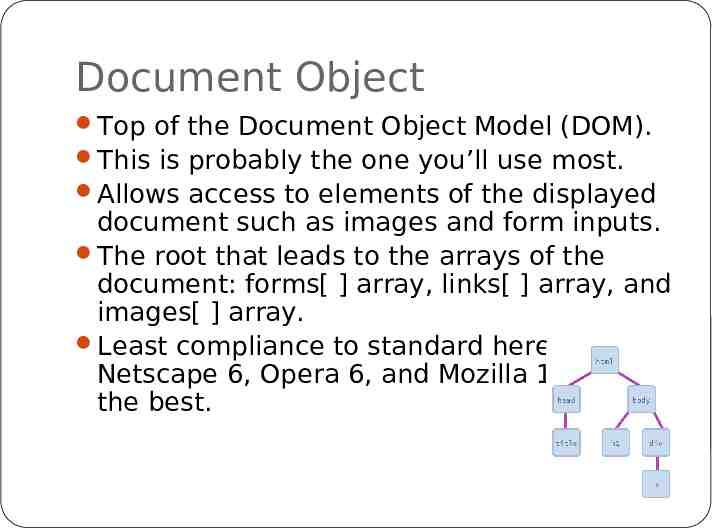
Document Object Top of the Document Object Model (DOM). This is probably the one you’ll use most. Allows access to elements of the displayed document such as images and form inputs. The root that leads to the arrays of the document: forms[ ] array, links[ ] array, and images[ ] array. Least compliance to standard here – Netscape 6, Opera 6, and Mozilla 1.0 are the best.
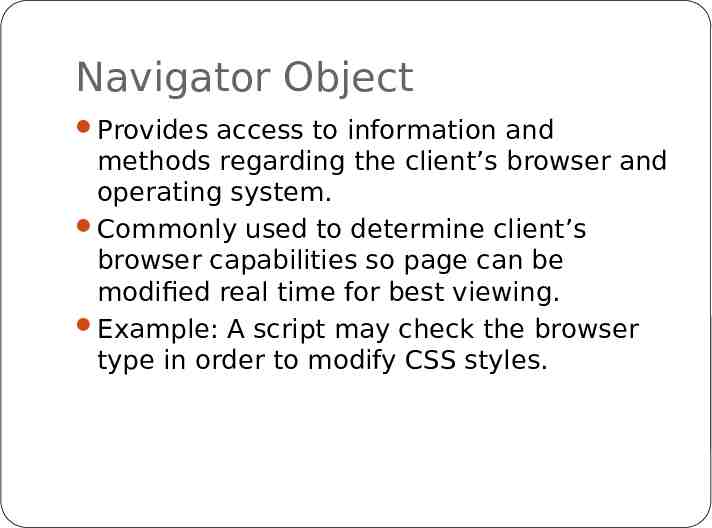
Navigator Object Provides access to information and methods regarding the client’s browser and operating system. Commonly used to determine client’s browser capabilities so page can be modified real time for best viewing. Example: A script may check the browser type in order to modify CSS styles.
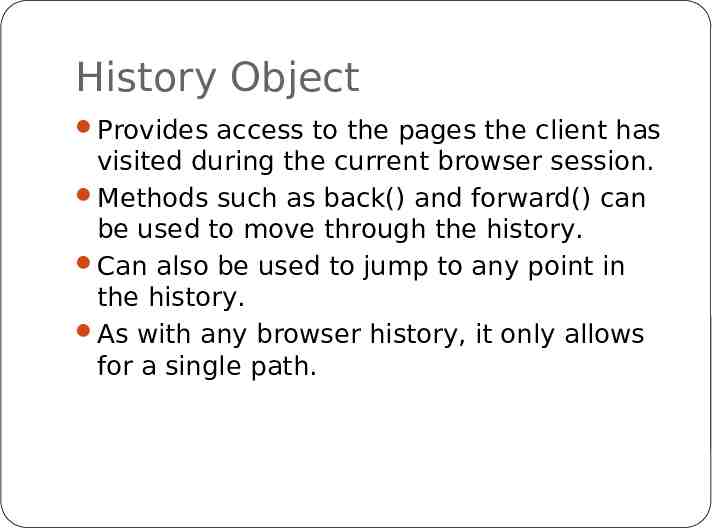
History Object Provides access to the pages the client has visited during the current browser session. Methods such as back() and forward() can be used to move through the history. Can also be used to jump to any point in the history. As with any browser history, it only allows for a single path.
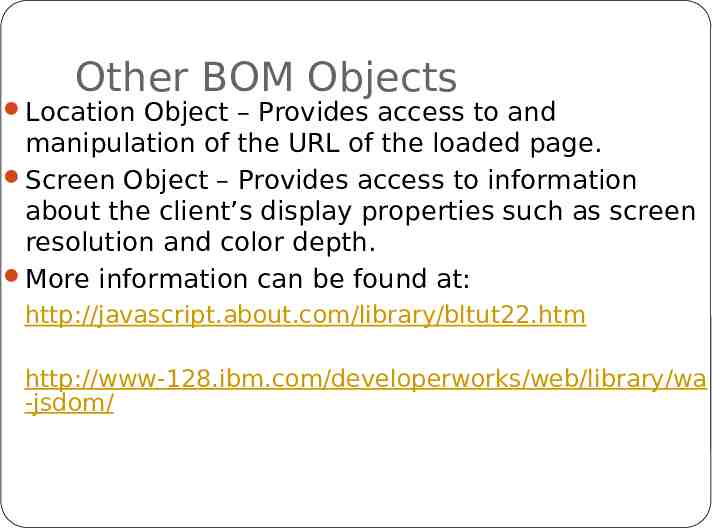
Other BOM Objects Location Object – Provides access to and manipulation of the URL of the loaded page. Screen Object – Provides access to information about the client’s display properties such as screen resolution and color depth. More information can be found at: http://javascript.about.com/library/bltut22.htm http://www-128.ibm.com/developerworks/web/library/wa -jsdom/
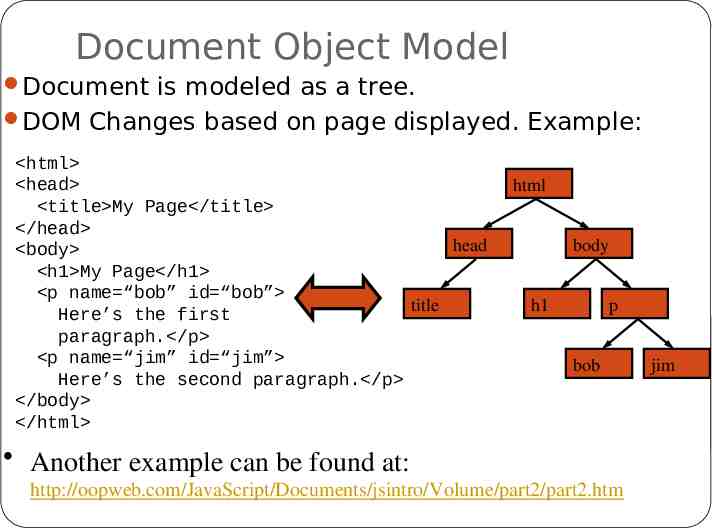
Document Object Model Document is modeled as a tree. DOM Changes based on page displayed. Example: html head title My Page /title /head head body h1 My Page /h1 p name “bob” id “bob” title Here’s the first paragraph. /p p name “jim” id “jim” Here’s the second paragraph. /p /body /html html body h1 p bob Another example can be found at: http://oopweb.com/JavaScript/Documents/jsintro/Volume/part2/part2.htm jim
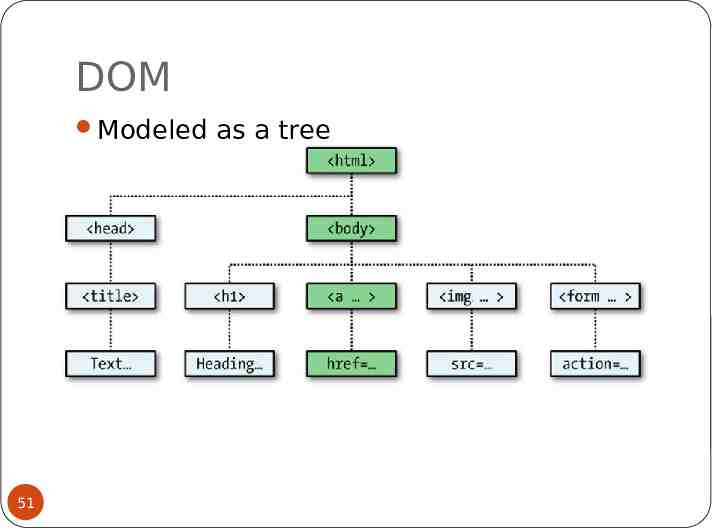
DOM Modeled as a tree 51
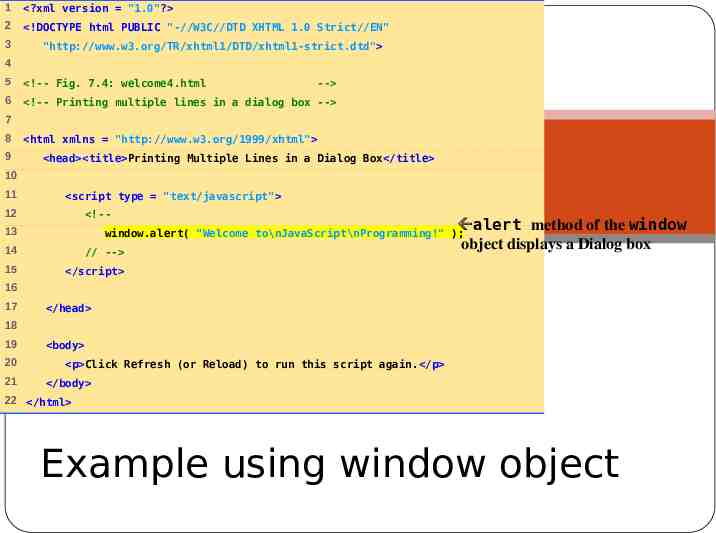
1 ?xml version "1.0"? 2 !DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" 3 "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd" 4 5 !-- Fig. 7.4: welcome4.html 6 !-- Printing multiple lines in a dialog box -- -- 7 8 9 head title Printing Multiple Lines in a Dialog Box /title 10 11 welcome4.html 1 of 1 html xmlns "http://www.w3.org/1999/xhtml" script type "text/javascript" 12 !-- 13 alert method of the window object displays a Dialog box window.alert( "Welcome to\nJavaScript\nProgramming!" ); 14 // -- 15 /script 16 17 /head 18 19 20 21 body p Click Refresh (or Reload) to run this script again. /p /body 22 /html Example using window object
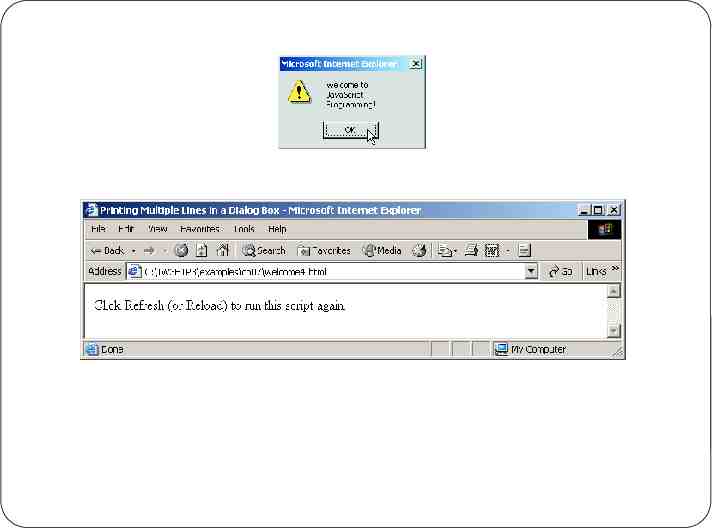
1 ?xml version "1.0"? 2 !DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" 3 "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd" 4 5 !-- Fig. 7.6: welcome5.html -- 6 !-- Using Prompt Boxes -- 7 8 9 10 welcome5.html (1 of 2) html xmlns "http://www.w3.org/1999/xhtml" head title Using Prompt and Alert Boxes /title 11 12 script type "text/javascript" 13 !-- 14 var name; // string entered by the user 15 JavaScript is a loosely typed language. Variables take on any data type depending on the value assigned. 16 // read the name from the prompt box as a string 17 name window.prompt( "Please enter your name", "GalAnt" ); 18 19 20 document.writeln( " h1 Hello, " name Value returned by the prompt method of the window object is assigned to the variable name ", welcome to JavaScript programming! /h1 " ); “ ” 21 // -- 22 /script symbol can be used for text concatenation as well as arithmetic operator More using window object - prompt
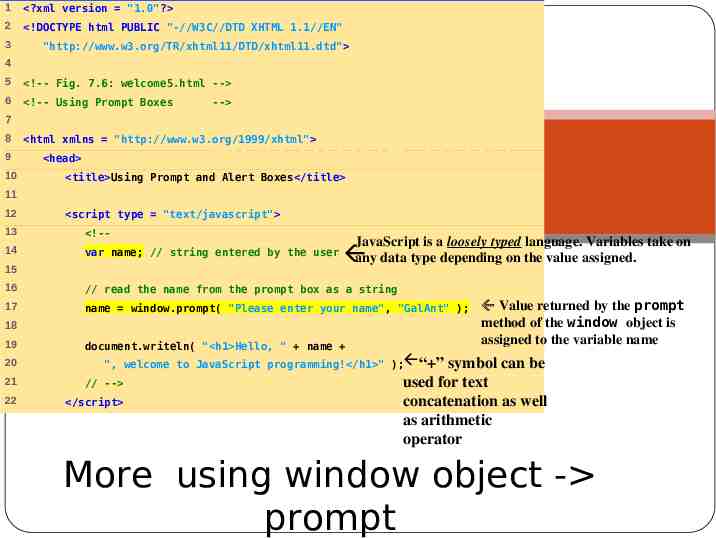
23 24 /head 25 26 body 27 p Click Refresh (or Reload) to run this script again. /p 28 /body 29 /html
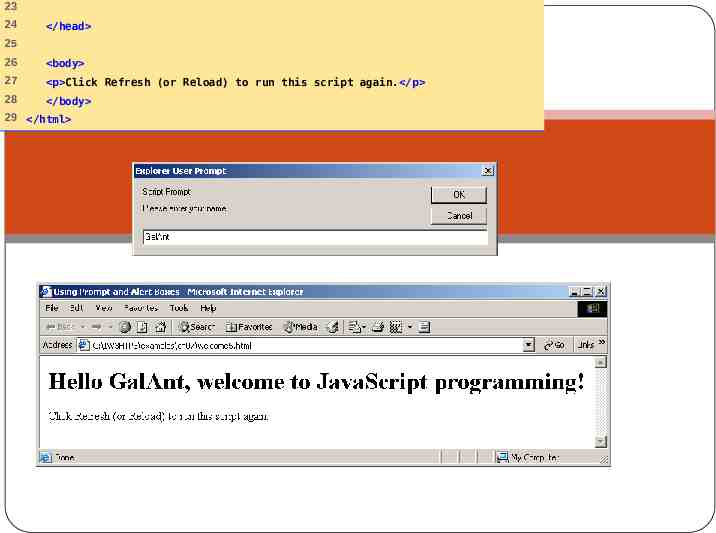
When the user clicks OK, the value typed by the user is returned to the program as a string. This is the prompt to the user. This is the default value that appears when the dialog opens. This is the text field in which the user types the value. If the user clicks Cancel, the null value will be returned to the program and no value will be assigned to the variable.
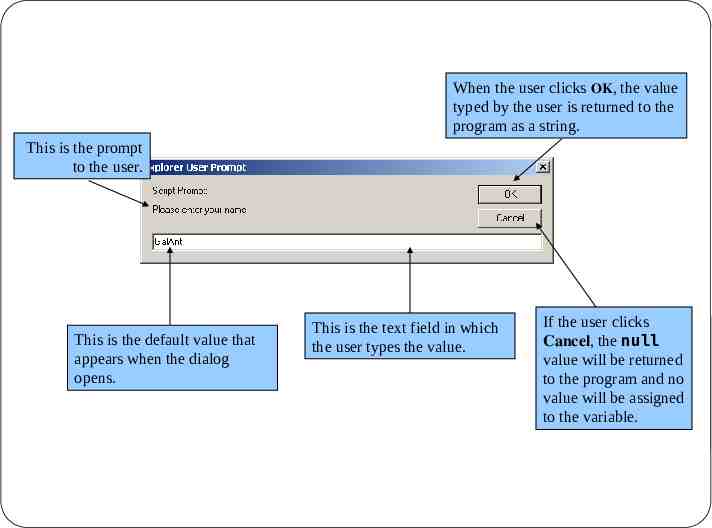
Another example using window object Simple Script Example: Adding Integers The values of numbers to be added are obtained as user inputs colleted through the window.prompt method parseInt Converts its string argument to an integer What happens if the conversion is not done? See example on our web site (not a number): value returned if nonnumerical values are passed to the paresInt method NaN
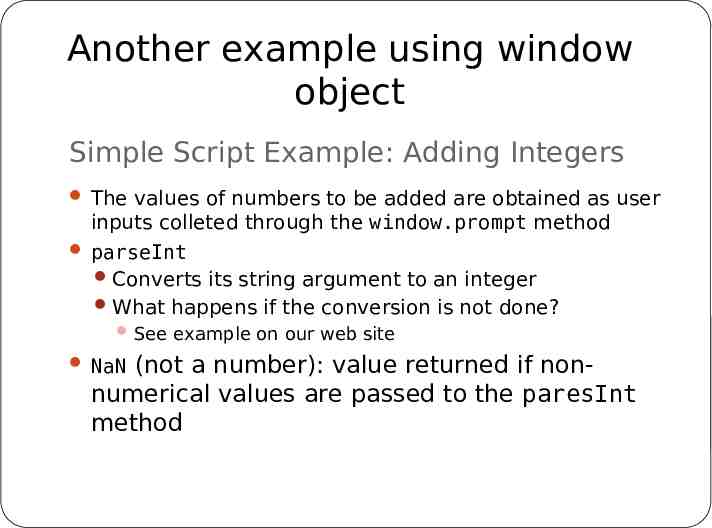
1 ?xml version "1.0"? 2 !DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" 3 "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd" 4 5 !-- Fig. 7.8: Addition.html -- 6 !-- Addition Program -- 7 8 9 10 Addition.html (1 of 2) html xmlns "http://www.w3.org/1999/xhtml" head title An Addition Program /title 11 12 script type "text/javascript" 13 !-- 14 var firstNumber, // first string entered by user 15 secondNumber, // second string entered by user 16 number1, // first number to add 17 number2, // second number to add 18 sum; // sum of number1 and number2 19 20 // read in first number from user as a string 21 firstNumber 22 23 window.prompt( "Enter first integer", "0" );
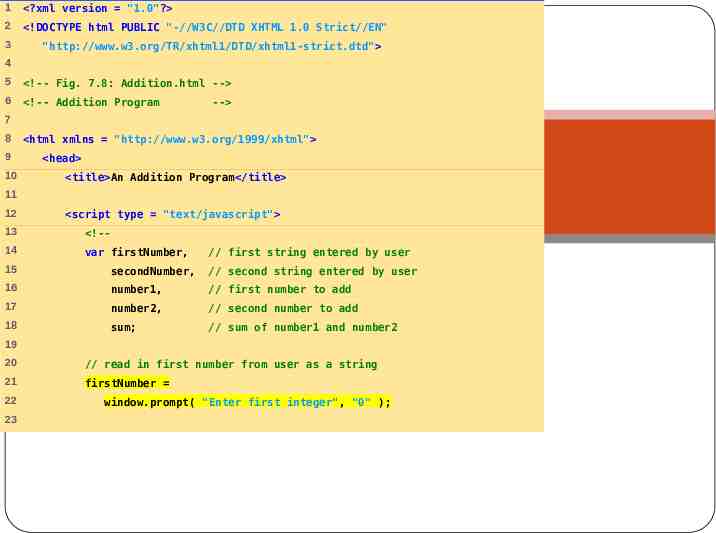
24 // read in second number from user as a string 25 secondNumber 26 window.prompt( "Enter second integer", "0" ); 27 28 // convert numbers from strings to integers 29 number1 parseInt( firstNumber ); 30 number2 parseInt( secondNumber ); 31 Addition.html (2 of 2) 32 // add the numbers 33 sum number1 number2; 34 35 // display the results 36 document.writeln( " h1 The sum is " sum " /h1 " ); 37 // -- 38 /script 39 40 /head 41 body 42 43 p Click Refresh (or Reload) to run the script again /p /body 44 /html
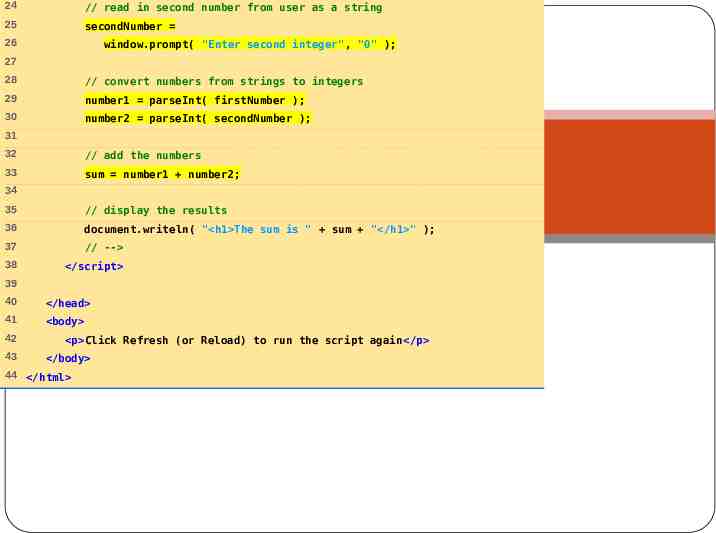
JavaScript Alert window html head title My Page /title /head body p a href "myfile.html" My Page /a br / a href "myfile.html" onMouseover "window.alert('Hello');" My Page /A /p JavaScript written /body An Event inside HTML /html
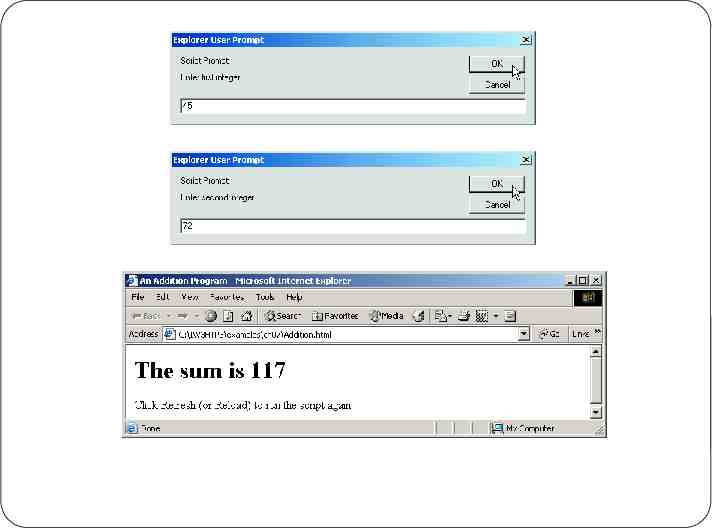
HTML Forms and JavaScript Using DOM 62

HTML Forms and JavaScript JavaScript is very good at processing user input in the web browser HTML form elements receive input Forms and form elements have unique names Each unique element can be identified Uses JavaScript Document Object Model (DOM)
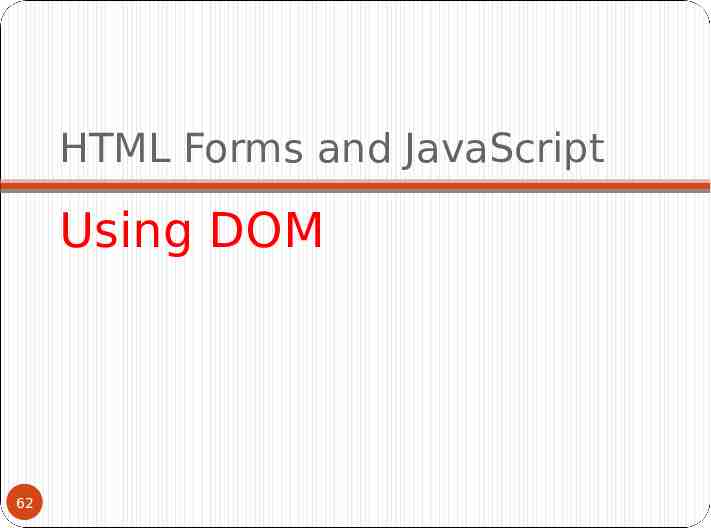
Naming Form Elements in HTML form name "addressform" Name: input name "yourname" br / Phone: input name "phone" br / Email: input name "email" br / /form
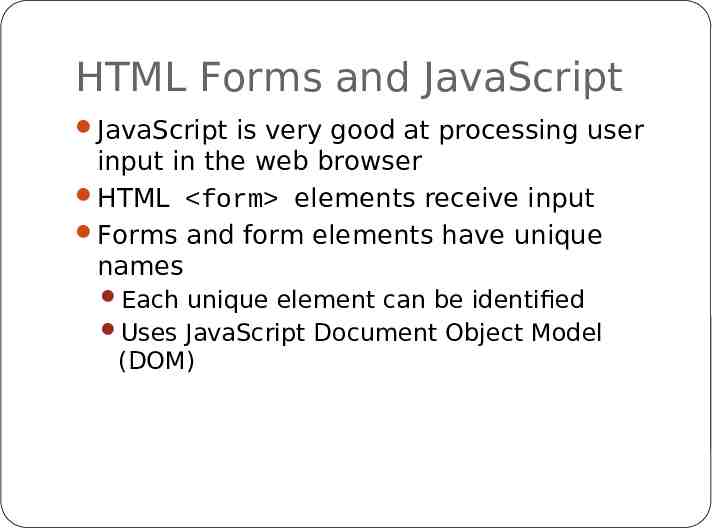
Forms and JavaScript document.formname.elementname.value Thus: document.addressform.yourname.value document.addressform.phone.value document.addressform.email.value
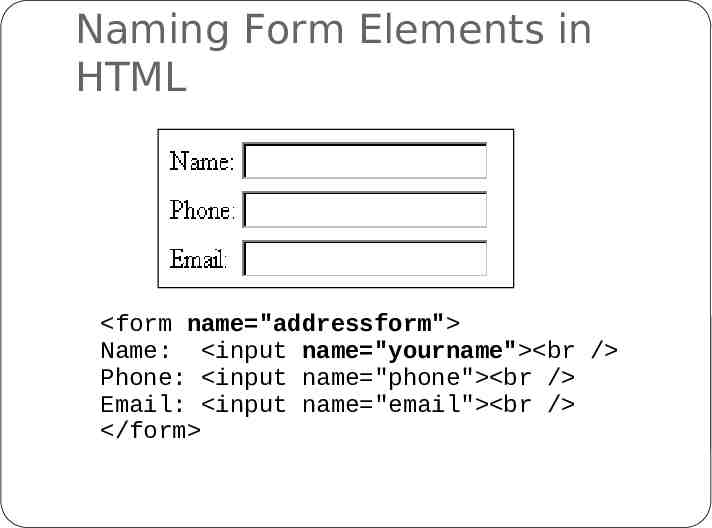
Using Form Data Personalising an alert box form name "alertform" Enter your name: input type "text" name "yourname" input type "button" value "Go" onClick "window.alert('Hello ' document.alertform.yourname.value);" /form
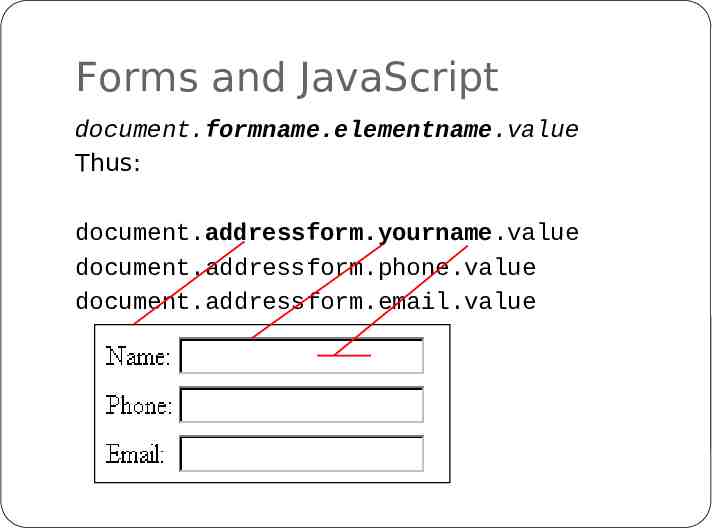
DOM and dynamic manipulation An example 67
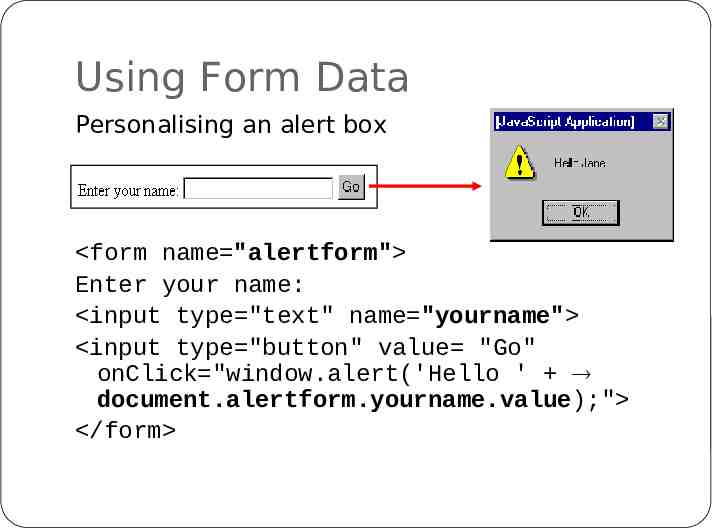
Events –you can based on triggered events run JavaScript (event handling) code Examples of events for your HTML pages include: onLoad onClick onMouseOver onMouseOut Each of these events can execute a script. Events must be placed in a tag. For example: a href "somesite.htm" onClick "javascript:function();" link text /a
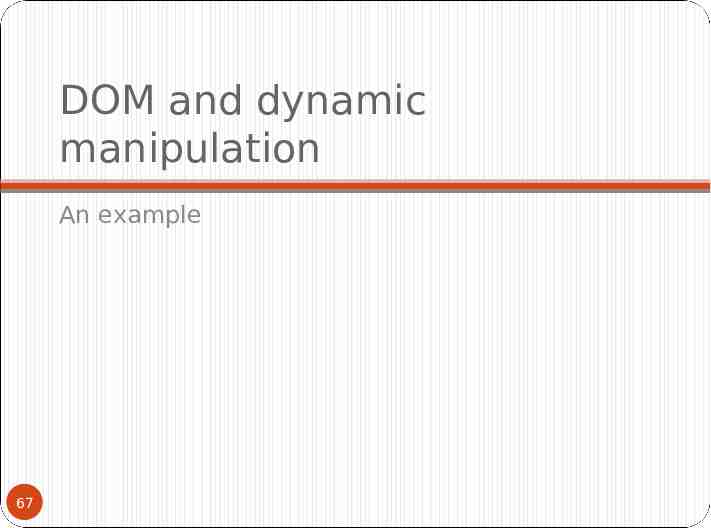
ALSO You can add or change elements on a page Some possibilities createElement(elementName) createTextNode(text) appendChild(newChild) removeChild(node) Example: Add a new list item: var list document.getElementById(‘list1') var newitem document.createElement('li') var newtext document.createTextNode(text) list.appendChild(newitem) newitem.appendChild(newtext) This example uses the browser Document Object Model (DOM).
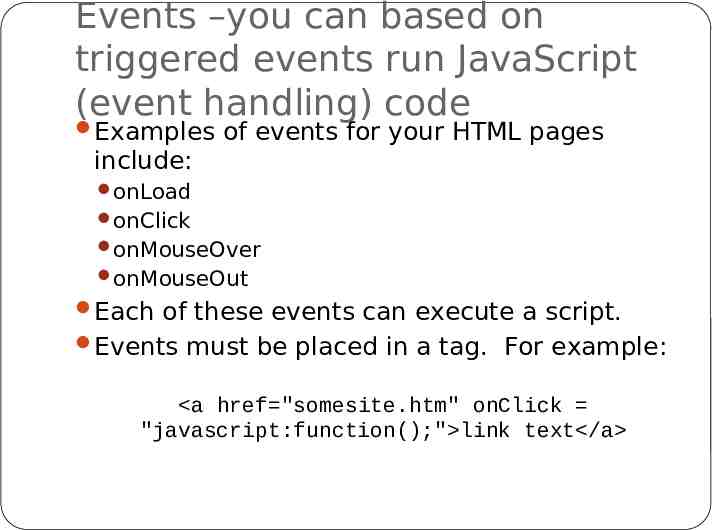
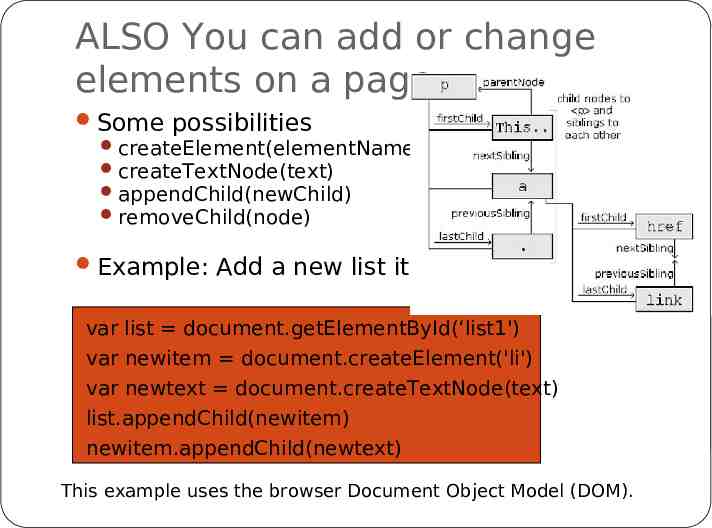