TOOL-532T Using Windows Runtime With C++ Herb Sutter Partner
31 Slides1.41 MB
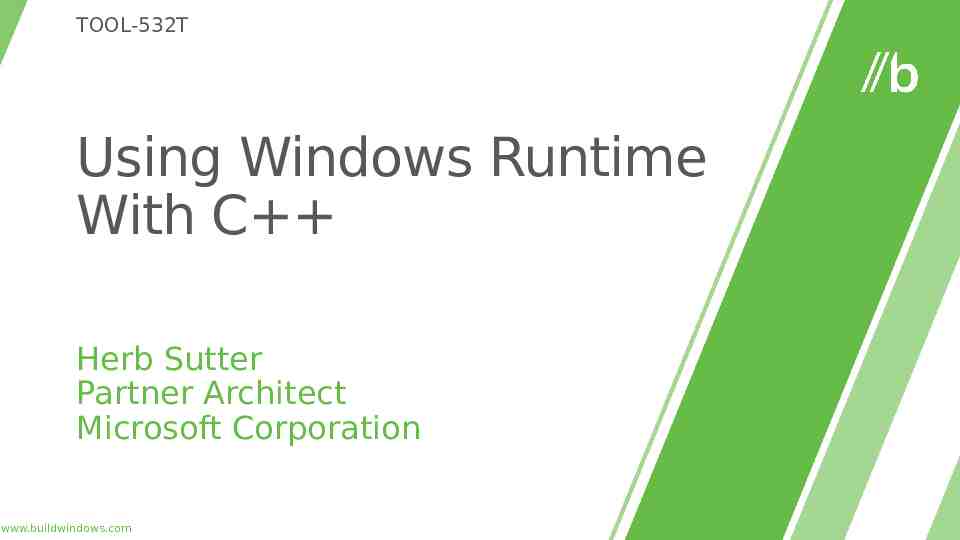
TOOL-532T Using Windows Runtime With C Herb Sutter Partner Architect Microsoft Corporation www.buildwindows.com

Agenda Overview Language Libraries Q&A www.buildwindows.com

Overview

Windows 8 Metro style Apps Cor Cor e e System System Services Services Model Model Controlle Controlle rr Vie Vie w w XAML www.buildwindows.com C C Desktop Apps HTML / CSS C# VB JavaScript (Chakra) WinRT APIs Communicatio n & Data Graphics & Media Application Model HTML JavaScri pt C C C# VB Internet Explore r Win3 2 .NE T/ SL Devices & Printing Windows Core OS Services

C in a Metro style World Overview Metro style Applications (Hybrid) HTML5 JS front-end C components XAML UI Applications XAML interface C code behind Metro style Games DirectX & C www.buildwindows.com

demo Magical Music Vikas Bhatia Program Manager Windows C www.buildwindows.com

C for Windows Runtime Overview Set of language extensions and libraries to allow direct consumption and authoring of Windows Runtime types. Strongly-typed system for Windows Runtime Automatically reference counted Exception-based Deep integration with STL Well defined binary contract across module boundaries www.buildwindows.com

C Component Extensions BINDINGS TO FOREIGN TYPE SYSTEMS (C /CX) Key Bindings Feature Summary 1. Data Types ref class Reference type value class Value type interface class Interface property Property with get/set event “Delegate property” with add/remove/raise delegate Type-safe function pointer generic Type-safe generics gcnew Garbage-collected allocation ref new Reference-counted allocation Strong pointer (“hat” or “handle”) % Strong reference 2. Allocation 3. Pointer & Reference

WinRT Types: For Cross-Language Use C/C External Surface for native callers/callees WinRT External Surface for WinRT callers/callees Module Internals written in C www.buildwindows.com

C for Windows Runtime delegate void CarouselInitDoneHandler(IUIAnimationVariable rotation); void CarouselAnimation::Initialize(double angle, CarouselInitDoneHandler callback) { // Create Animation Manager using namespace Engine::Graphics; UIAnimationManager animationManager; // Create rotation animation variable IUIAnimationVariable rotation animationManager.CreateAnimationVariable(angle); // Set the event handler for the story board rotation- GetCurrentStoryBoard()- SetStoryBoardEventHandler( ref new CarouselStoryBoardHandler(this, CarouselAnimation::StoryBoard) ); } // Invoke the callback when done callback(rotation);

Language

Lifetime Management Handle ( ) is a pointer to a Windows Runtime object for which the compiler performs automatic reference counting ref new instantiates or activates a Windows Runtime class. Person p; { Person p2 ref new Person(); // refcount 1 p2- Name “John”; // refcount 1 p p2; // refcount 2 } // refcount 1 www.buildwindows.com p nullptr; // refcount 0; Person()

Runtime Class Defining public ref class Person { public: Person(String name, String email); void Greet(Person other); internal: Person(); void SetPassword(const std::wstring& passwd); }; ABI-safe cross-language class Public methods restricted to WinRT typed parameters Private/internal methods can use any legal C type parameters Using Person p ref new Person(“John Surname”); p- Greet(ref new Person(“Jim Surename”); www.buildwindows.com

Interface Defining public interface class IAnimal { void Play(); }; Methods are implicitly public Using IAnimal animal ref new Cat(); animal- Play(); www.buildwindows.com Inheriting public interface class IFeline : IAnimal { void Scratch(); }; Implementing ref class Cat : IFeline { public: virtual void Play(); virtual void Scratch(); }; Public inheritance only

Automatic Lifetime (Stack, Member) Defining public ref class DatabaseConnection { public: DatabaseConnection(); }; Using { DatabaseConnection db(); db.SetName( “Employees”); // // lots of queries, updates, etc. // } // DatabaseConnection() www.buildwindows.com

Property Defining Trivial properties (with private backing store) public: property String Name; User defined properties public: property Person Sibling { Person get() { InitSiblings(); return sibling; } void set(Person value) { sibling value; NotifySibling(); } } private: Person sibling; Using Person p ref new Person(“John”); p- Sibling ref new Person(p- Name); www.buildwindows.com

Delegate Declaring: like a function public delegate void PropertyChanged( String propName, String propValue ); Instantiating: like a class From lambda: auto p ref new PropertyChanged( [](String pn, String pv) { cout pn ” “ pv; } ); From free-function auto p ref new PropertyChanged( UIPropertyChanged ); From class-member auto p ref new PropertyChanged( this, MainPage::OnPropertyChanged ); Invoking: like a function p( “Visible”, false ); www.buildwindows.com

Event Defining Trivial event (with private backing store) public: event PropertyChanged OnPropertyChanged; User defined event public: event PropertyChanged OnNetworkChanged { EventRegistrationToken add(PropertyChanged ); void remove(EventRegistrationToken t); void raise(String , String ); } Using Subscribing person- OnPropertyChanged propertyChangedDelegate; auto token person- OnPropertyChanged::add(propertyChangedDelegate); Unsubscribing person- OnPropertyChanged - token; person- OnPropertyChanged::remove(token); www.buildwindows.com

Exception E OUTOFMEMORY E INVALIDARG OutOfMemoryException InvalidArgumentExceptio n InvalidCastException NullReferenceException NotImplementedExceptio n AccessDeniedException FailureException OutOfBoundsException ChangedStateException ClassNotRegisteredExcep tion DisconnectedException OperationCanceledExcep tion Signaling an error case: throw exception throw ref new InvalidArgumentException(); throw ref new COMException(E *); E ACCESSDENIED E FAIL E BOUNDS E CHANGED STATE REGDB E CLASSNOT REG E DISCONNECTED E ABORT Handling an error case: catch exception Exception E NOINTERFACE E POINTER E NOTIMPL HRESULT try { } catch (OutOfMemoryException ex) { } Access HRESULT value via ex- HResult Notes on exceptions: catch (Platform::Exception ) catches all WinRT exceptions Exceptions don’t carry any state and don’t travel across modules Deriving from an exception class is ill-formed www.buildwindows.com

Generics Defining generic typename T, typename U public interface class IPair { property T First; property U Second; }; Using IPair String , Uri uri GetUri(); auto first uri- First; // type is String auto second uri- Second; // type is Uri www.buildwindows.com Implementing ref class PairStringUri: IPair String , Uri { public: property String First; property Uri Second; };

Template Runtime Class Defining template typename T, typename U ref class Pair: IPair T, U { public: property T First; property U Second; Pair(T first, U second) { First first; Second second; } }; Using IPair String , Uri pair ref new Pair String , Uri ( “//BUILD/”, ref new Uri(“http://www.buildwindows.com”)); www.buildwindows.com

.winmd metadata .winmd files Contain the metadata representation of WinRT types To consume a winmd file: Right click on project in Solution Explorer References Add New Reference #using Company.Component.winmd Or Make sure the winmd and implementation dll is packaged together with your application To produce a .winmd file: Start from the “C WinRT Component Dll” template Define public types (ref classes, interfaces, delegates, etc.) Tip: C WinRT components can be consumed from C , JS or C# www.buildwindows.com

Partial Runtime Class Partial class definition private partial ref class MainPage: UserControl, IComponentConnector { public: void InitializeComponent(); void Connect() { btn1- Click ref new EventHandler(this, &MainPage::Button Click); } }; Class definition ref class MainPage { public: MainPage() { InitializeComponent(); } void Button Click(Object sender, RoutedEventArgs e); }; www.buildwindows.com
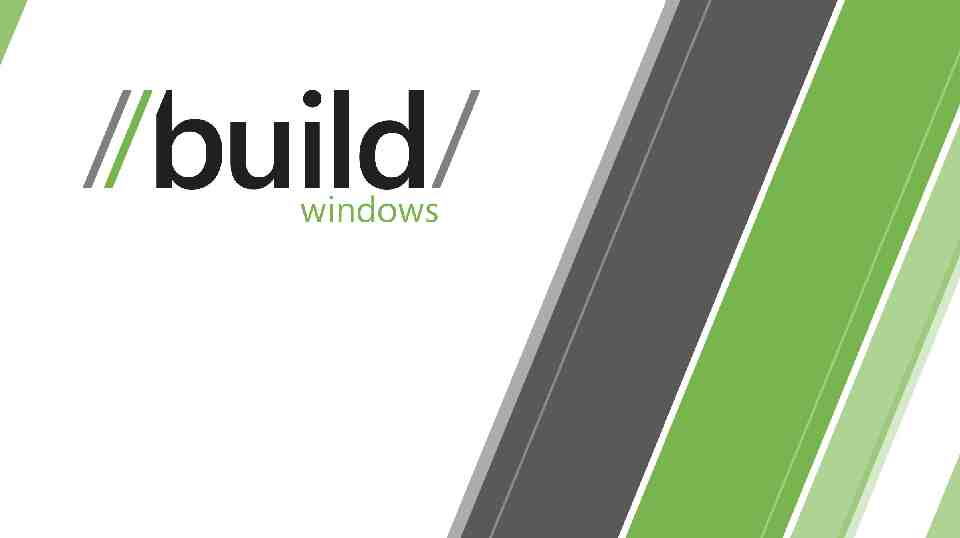
Libraries

Vector and ObservableVector Instantiating using namespace Platform; Vector String items ref new Vector String (); Adding elements items- Append(“Hello”); Returning a read-only view of the vector IVectorView String GetItems () { return items- GetView(); } Getting notification for changes items- VectorChanged ref new VectorChangedEventHandler String (this, &MyClass::VectorChanged); www.buildwindows.com

Map and ObservableMap Defining using namespace Platform; Map String , Uri favorites ref new Map String , Uri (); Adding elements favorites- Insert(“MSDN”, ref new Uri(“http://msdn.com”)); Checking and removing elements if (favorites- HasKey(“MSDN”)) favorites- Remove(“MSDN”); www.buildwindows.com

Library Integration: STL STL Algorithms String has Begin()/End() member methods. For WinRT collections, collection.h defines begin() and end() functions. IVector int v GetItems(); int sum 0; std::for each( begin(v), end(v), [&sum](int element) { sum element; } ); www.buildwindows.com Conversions String std::wstring (via wchar t*) Vector std::vector std::vector int v; v.push back(10); auto items ref new Vector int (v); Vector std::vector Vector int items ; std::vector int v to vector(items); Map std::map

For more information RELATED SESSIONS 100: Improving Software Quality Using Visual Studio 11 C Code Analysis Rong Lu 479: A Lap Around Visual Studio 11 Express for Metro style Apps Using C Vikas Bhatia, Joanna Mason 761: A Lap Around DirectX Game Development Tools Boris Jabes 802: Taming GPU Compute with C AMP Daniel Moth 835: Writing Modern C Code: How C has evolved over the years Herb Sutter 845: Tips and Tricks for Developing Metro style Apps Using C Tarek Madkour www.buildwindows.com ONLINE TALKS ON CHANNEL 9 Bringing Existing C Code into Metro style Apps Ale Contenti Under the Covers with C for Metro style Apps Deon Brewis Building Metro style apps with HTML5 and Compiled Code Raman Sharma

Q&A

2011 Microsoft Corporation. All rights reserved. Microsoft, Windows, Windows Vista and other product names are or may be registered trademarks and/or trademarks in the U.S. and/or other countries. The information herein is for informational purposes only and represents the current view of Microsoft Corporation as of the date of this presentation. Because Microsoft must respond to changing market conditions, it should not be interpreted to be a commitment on the part of Microsoft, and Microsoft cannot guarantee the accuracy of any information provided after the date of this presentation. MICROSOFT MAKES NO WARRANTIES, EXPRESS, IMPLIED OR STATUTORY, AS TO THE INFORMATION IN THIS PRESENTATION.
