Classes and Objects
50 Slides379.50 KB

Classes and Objects
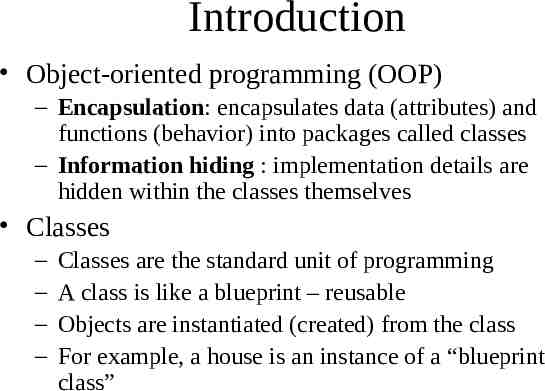
Introduction Object-oriented programming (OOP) – Encapsulation: encapsulates data (attributes) and functions (behavior) into packages called classes – Information hiding : implementation details are hidden within the classes themselves Classes – – – – Classes are the standard unit of programming A class is like a blueprint – reusable Objects are instantiated (created) from the class For example, a house is an instance of a “blueprint class”
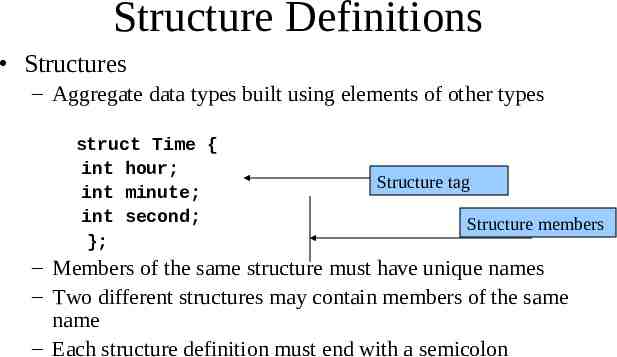
Structure Definitions Structures – Aggregate data types built using elements of other types struct Time { int hour; int minute; int second; }; Structure tag Structure members – Members of the same structure must have unique names – Two different structures may contain members of the same name – Each structure definition must end with a semicolon
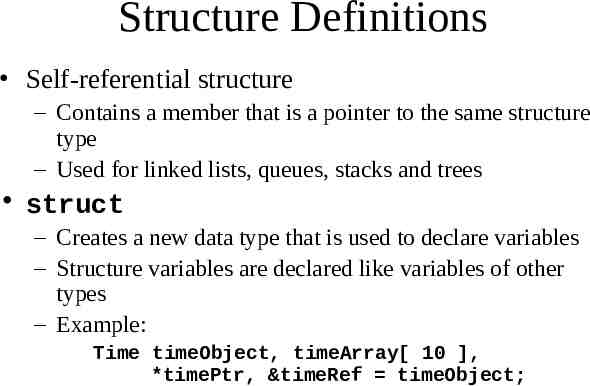
Structure Definitions Self-referential structure – Contains a member that is a pointer to the same structure type – Used for linked lists, queues, stacks and trees struct – Creates a new data type that is used to declare variables – Structure variables are declared like variables of other types – Example: Time timeObject, timeArray[ 10 ], *timePtr, &timeRef timeObject;
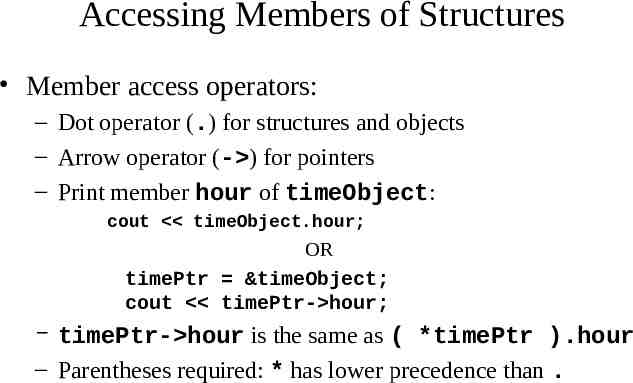
Accessing Members of Structures Member access operators: – Dot operator (.) for structures and objects – Arrow operator (- ) for pointers – Print member hour of timeObject: cout timeObject.hour; OR timePtr &timeObject; cout timePtr- hour; – timePtr- hour is the same as ( *timePtr ).hour – Parentheses required: * has lower precedence than .
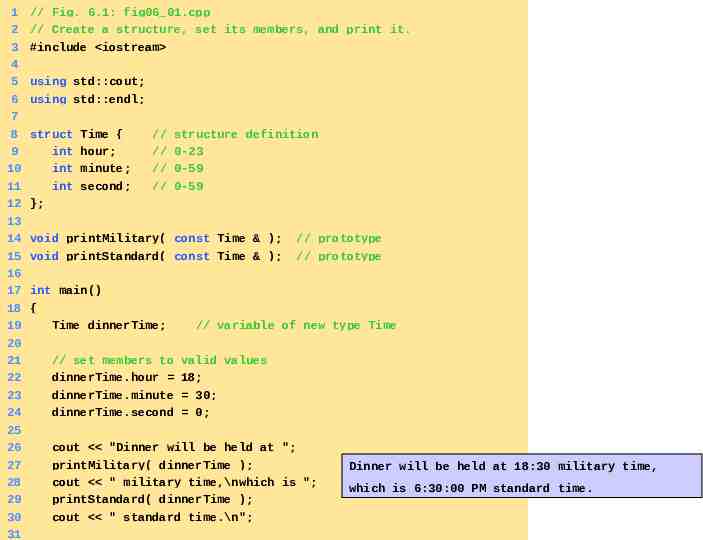
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 // Fig. 6.1: fig06 01.cpp // Create a structure, set its members, and print it. #include iostream using std::cout; using std::endl; struct int int int }; Time { hour; minute; second; // // // // structure definition 0-23 0-59 0-59 void printMilitary( const Time & ); void printStandard( const Time & ); int main() { Time dinnerTime; // set members to dinnerTime.hour dinnerTime.minute dinnerTime.second // prototype // prototype // variable of new type Time valid values 18; 30; 0; cout "Dinner will be held at "; printMilitary( dinnerTime ); cout " military time,\nwhich is "; printStandard( dinnerTime ); cout " standard time.\n"; Dinner will be held at 18:30 military time, which is 6:30:00 PM standard time.
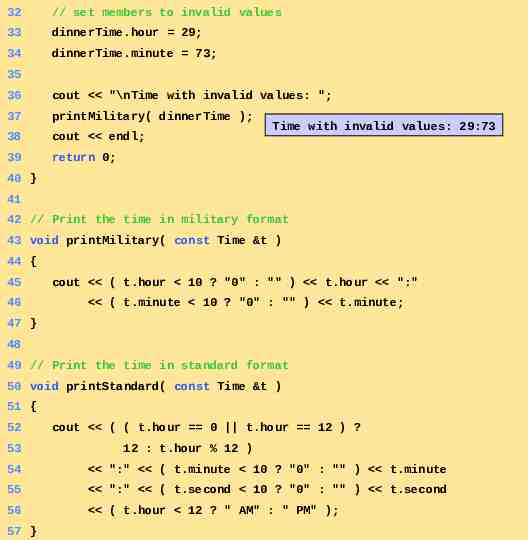
32 // set members to invalid values 33 dinnerTime.hour 29; 34 dinnerTime.minute 73; 35 36 cout "\nTime with invalid values: "; 37 printMilitary( dinnerTime ); 38 cout endl; 39 return 0; Time with invalid values: 29:73 40 } 41 42 // Print the time in military format 43 void printMilitary( const Time &t ) 44 { 45 46 cout ( t.hour 10 ? "0" : "" ) t.hour ":" ( t.minute 10 ? "0" : "" ) t.minute; 47 } 48 49 // Print the time in standard format 50 void printStandard( const Time &t ) 51 { 52 53 cout ( ( t.hour 0 t.hour 12 ) ? 12 : t.hour % 12 ) 54 ":" ( t.minute 10 ? "0" : "" ) t.minute 55 ":" ( t.second 10 ? "0" : "" ) t.second 56 ( t.hour 12 ? " AM" : " PM" ); 57 }
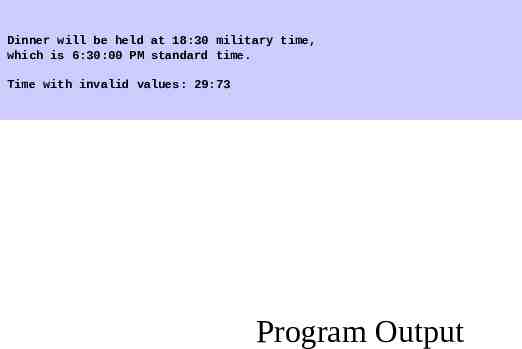
Dinner will be held at 18:30 military time, which is 6:30:00 PM standard time. Time with invalid values: 29:73 Program Output
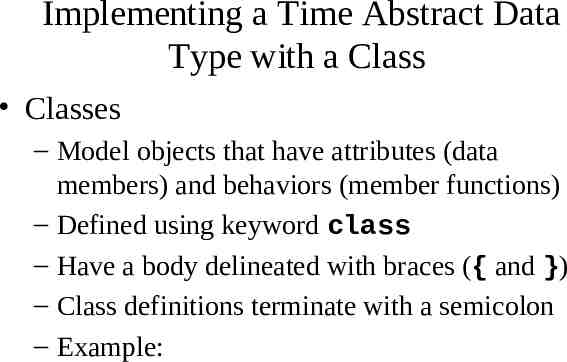
Implementing a Time Abstract Data Type with a Class Classes – Model objects that have attributes (data members) and behaviors (member functions) – Defined using keyword class – Have a body delineated with braces ({ and }) – Class definitions terminate with a semicolon – Example:
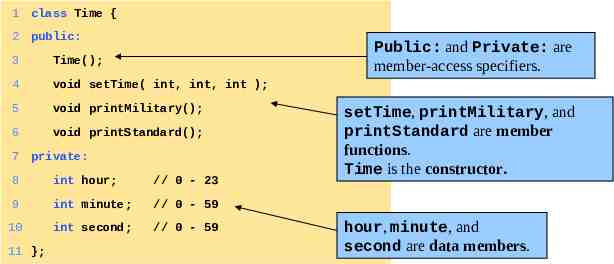
1 class Time { 2 public: Public: and Private: are member-access specifiers. 3 Time(); 4 void setTime( int, int, int ); 5 void printMilitary(); 6 void printStandard(); 7 private: 8 int hour; // 0 - 23 9 int minute; // 0 - 59 10 int second; // 0 - 59 11 }; setTime, printMilitary, and printStandard are member functions. Time is the constructor. hour, minute, and second are data members.
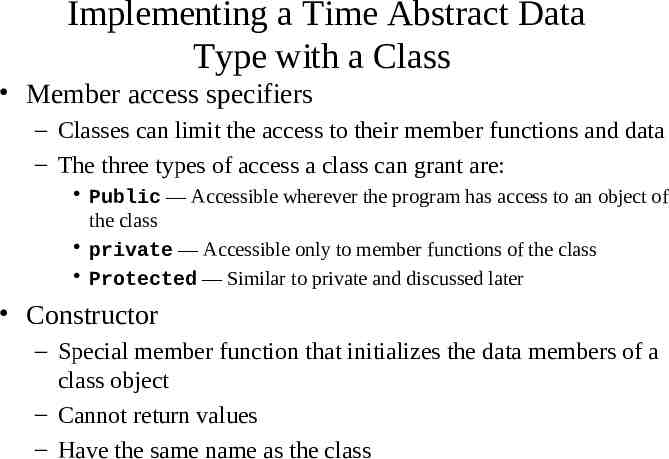
Implementing a Time Abstract Data Type with a Class Member access specifiers – Classes can limit the access to their member functions and data – The three types of access a class can grant are: Public — Accessible wherever the program has access to an object of the class private — Accessible only to member functions of the class Protected — Similar to private and discussed later Constructor – Special member function that initializes the data members of a class object – Cannot return values – Have the same name as the class
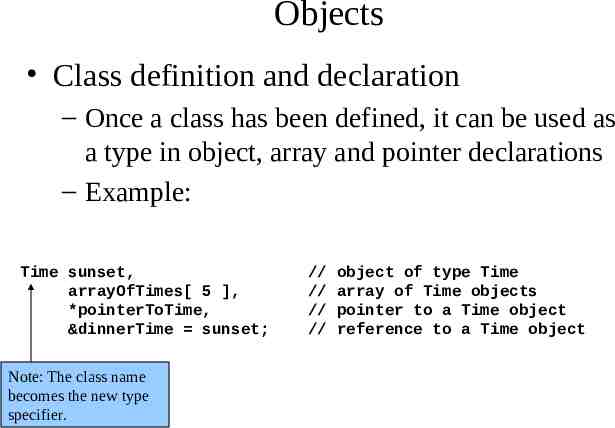
Objects Class definition and declaration – Once a class has been defined, it can be used as a type in object, array and pointer declarations – Example: Time sunset, arrayOfTimes[ 5 ], *pointerToTime, &dinnerTime sunset; Note: The class name becomes the new type specifier. // // // // object of type Time array of Time objects pointer to a Time object reference to a Time object
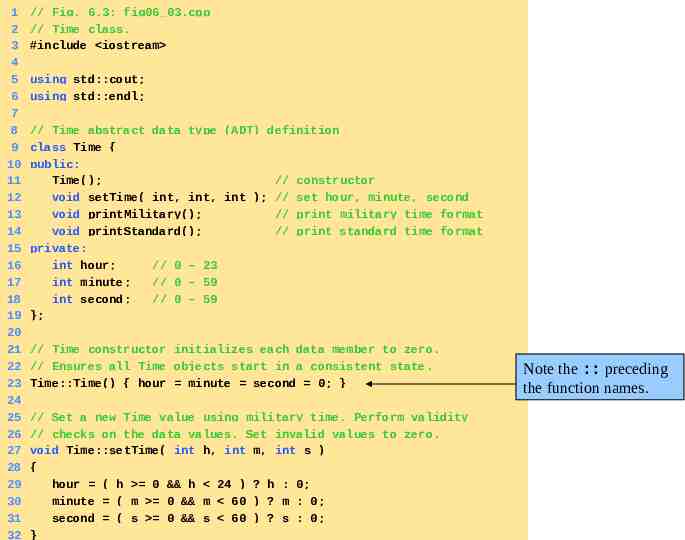
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 // Fig. 6.3: fig06 03.cpp // Time class. #include iostream using std::cout; using std::endl; // Time abstract data type (ADT) definition class Time { public: Time(); // constructor void setTime( int, int, int ); // set hour, minute, second void printMilitary(); // print military time format void printStandard(); // print standard time format private: int hour; // 0 – 23 int minute; // 0 – 59 int second; // 0 – 59 }; // Time constructor initializes each data member to zero. // Ensures all Time objects start in a consistent state. Time::Time() { hour minute second 0; } // Set a new Time value using military time. Perform validity // checks on the data values. Set invalid values to zero. void Time::setTime( int h, int m, int s ) { hour ( h 0 && h 24 ) ? h : 0; minute ( m 0 && m 60 ) ? m : 0; second ( s 0 && s 60 ) ? s : 0; } Note the :: preceding the function names.
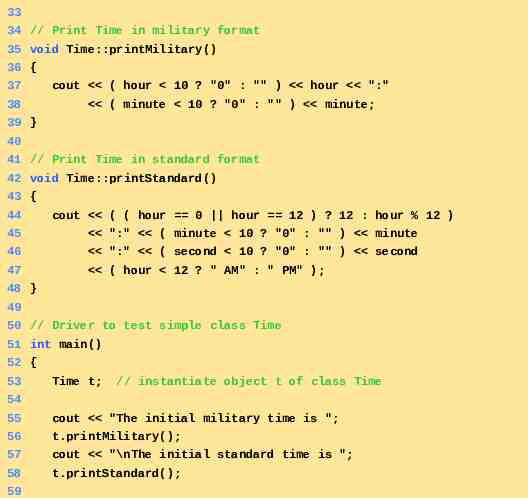
33 34 // Print Time in military format 35 void Time::printMilitary() 36 { 37 38 cout ( hour 10 ? "0" : "" ) hour ":" ( minute 10 ? "0" : "" ) minute; 39 } 40 41 // Print Time in standard format 42 void Time::printStandard() 43 { 44 cout ( ( hour 0 hour 12 ) ? 12 : hour % 12 ) 45 ":" ( minute 10 ? "0" : "" ) minute 46 ":" ( second 10 ? "0" : "" ) second 47 ( hour 12 ? " AM" : " PM" ); 48 } 49 50 // Driver to test simple class Time 51 int main() 52 { 53 Time t; // instantiate object t of class Time 54 55 cout "The initial military time is "; 56 t.printMilitary(); 57 cout "\nThe initial standard time is "; 58 t.printStandard(); 59
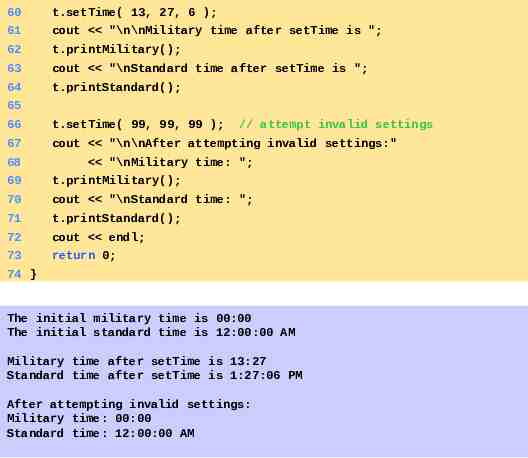
60 t.setTime( 13, 27, 6 ); 61 cout "\n\nMilitary time after setTime is "; 62 t.printMilitary(); 63 cout "\nStandard time after setTime is "; 64 t.printStandard(); 65 66 t.setTime( 99, 99, 99 ); 67 cout "\n\nAfter attempting invalid settings:" 68 // attempt invalid settings "\nMilitary time: "; 69 t.printMilitary(); 70 cout "\nStandard time: "; 71 t.printStandard(); 72 cout endl; 73 return 0; 74 } The initial military time is 00:00 The initial standard time is 12:00:00 AM Military time after setTime is 13:27 Standard time after setTime is 1:27:06 PM After attempting invalid settings: Military time: 00:00 Standard time: 12:00:00 AM
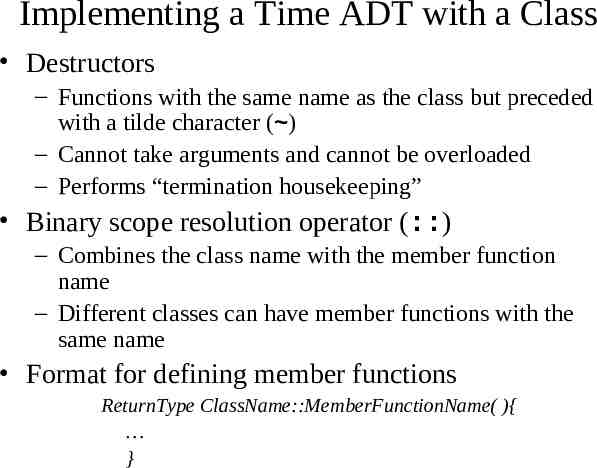
Implementing a Time ADT with a Class Destructors – Functions with the same name as the class but preceded with a tilde character ( ) – Cannot take arguments and cannot be overloaded – Performs “termination housekeeping” Binary scope resolution operator (::) – Combines the class name with the member function name – Different classes can have member functions with the same name Format for defining member functions ReturnType ClassName::MemberFunctionName( ){ }
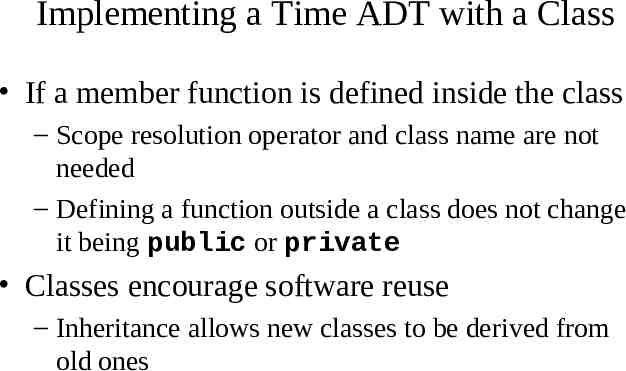
Implementing a Time ADT with a Class If a member function is defined inside the class – Scope resolution operator and class name are not needed – Defining a function outside a class does not change it being public or private Classes encourage software reuse – Inheritance allows new classes to be derived from old ones
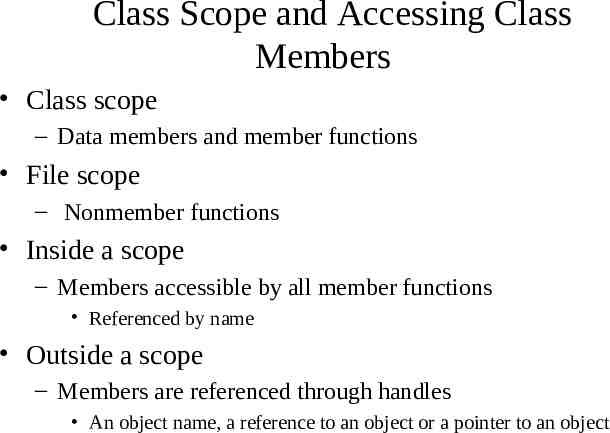
Class Scope and Accessing Class Members Class scope – Data members and member functions File scope – Nonmember functions Inside a scope – Members accessible by all member functions Referenced by name Outside a scope – Members are referenced through handles An object name, a reference to an object or a pointer to an object
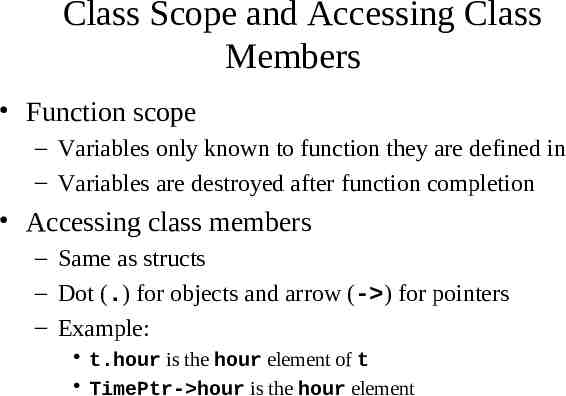
Class Scope and Accessing Class Members Function scope – Variables only known to function they are defined in – Variables are destroyed after function completion Accessing class members – Same as structs – Dot (.) for objects and arrow (- ) for pointers – Example: t.hour is the hour element of t TimePtr- hour is the hour element
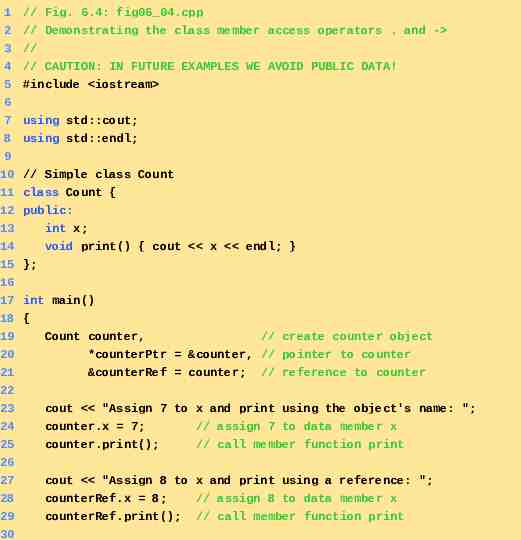
1 // Fig. 6.4: fig06 04.cpp 2 // Demonstrating the class member access operators . and - 3 // 4 // CAUTION: IN FUTURE EXAMPLES WE AVOID PUBLIC DATA! 5 #include iostream 6 7 using std::cout; 8 using std::endl; 9 10 // Simple class Count 11 class Count { 12 public: 13 int x; 14 void print() { cout x endl; } 15 }; 16 17 int main() 18 { 19 20 21 Count counter, // create counter object *counterPtr &counter, // pointer to counter &counterRef counter; // reference to counter 22 23 24 cout "Assign 7 to x and print using the object's name: "; counter.x 7; // assign 7 to data member x 25 counter.print(); // call member function print 26 27 28 cout "Assign 8 to x and print using a reference: "; counterRef.x 8; // assign 8 to data member x 29 counterRef.print(); 30 // call member function print
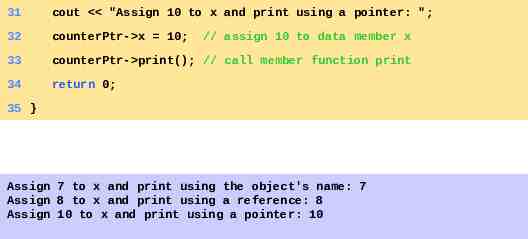
31 cout "Assign 10 to x and print using a pointer: "; 32 counterPtr- x 10; 33 counterPtr- print(); // call member function print 34 return 0; // assign 10 to data member x 35 } Assign 7 to x and print using the object's name: 7 Assign 8 to x and print using a reference: 8 Assign 10 to x and print using a pointer: 10
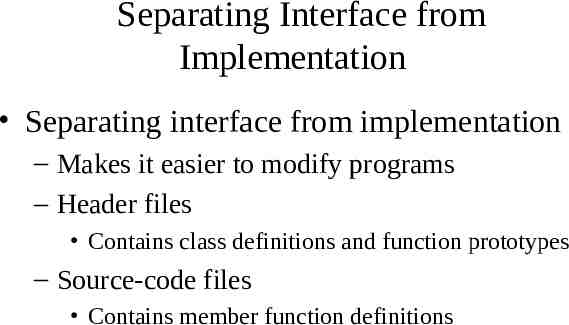
Separating Interface from Implementation Separating interface from implementation – Makes it easier to modify programs – Header files Contains class definitions and function prototypes – Source-code files Contains member function definitions
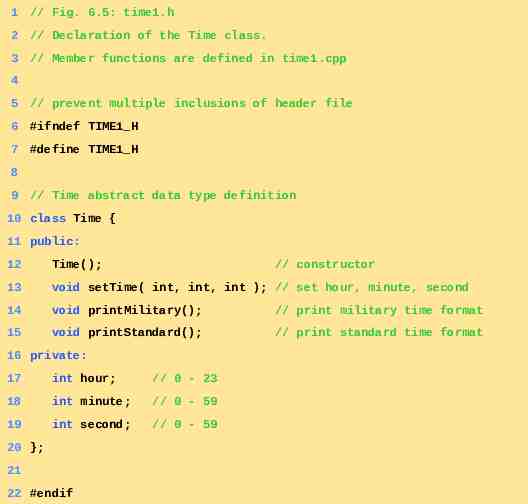
1 // Fig. 6.5: time1.h 2 // Declaration of the Time class. 3 // Member functions are defined in time1.cpp 4 5 // prevent multiple inclusions of header file 6 #ifndef TIME1 H 7 #define TIME1 H 8 9 // Time abstract data type definition 10 class Time { 11 public: 12 Time(); // constructor 13 void setTime( int, int, int ); // set hour, minute, second 14 void printMilitary(); // print military time format 15 void printStandard(); // print standard time format 16 private: 17 int hour; // 0 - 23 18 int minute; // 0 - 59 19 int second; // 0 - 59 20 }; 21 22 #endif
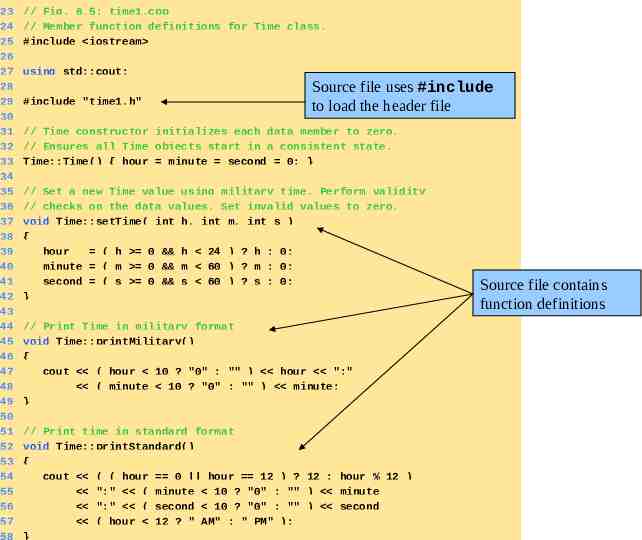
23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 // Fig. 6.5: time1.cpp // Member function definitions for Time class. #include iostream using std::cout; #include "time1.h" Source file uses #include to load the header file // Time constructor initializes each data member to zero. // Ensures all Time objects start in a consistent state. Time::Time() { hour minute second 0; } // Set a new Time value using military time. Perform validity // checks on the data values. Set invalid values to zero. void Time::setTime( int h, int m, int s ) { hour ( h 0 && h 24 ) ? h : 0; minute ( m 0 && m 60 ) ? m : 0; second ( s 0 && s 60 ) ? s : 0; } // Print Time in military format void Time::printMilitary() { cout ( hour 10 ? "0" : "" ) hour ":" ( minute 10 ? "0" : "" ) minute; } // Print time in standard format void Time::printStandard() { cout ( ( hour 0 hour 12 ) ? 12 : hour % 12 ) ":" ( minute 10 ? "0" : "" ) minute ":" ( second 10 ? "0" : "" ) second ( hour 12 ? " AM" : " PM" ); } Source file contains function definitions
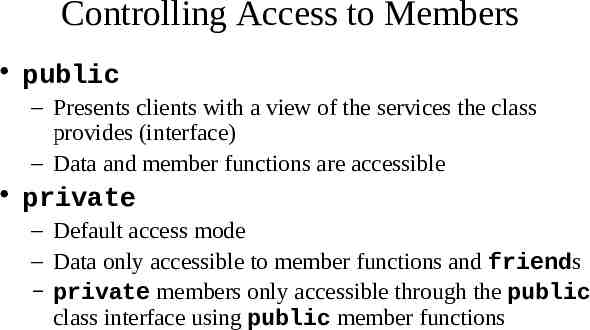
Controlling Access to Members public – Presents clients with a view of the services the class provides (interface) – Data and member functions are accessible private – Default access mode – Data only accessible to member functions and friends – private members only accessible through the public class interface using public member functions
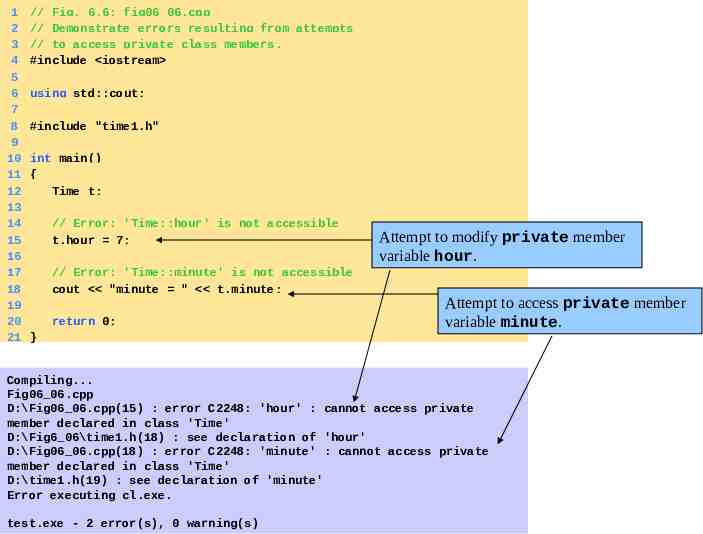
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 // Fig. 6.6: fig06 06.cpp // Demonstrate errors resulting from attempts // to access private class members. #include iostream using std::cout; #include "time1.h" int main() { Time t; // Error: 'Time::hour' is not accessible t.hour 7; // Error: 'Time::minute' is not accessible cout "minute " t.minute; return 0; } Attempt to modify private member variable hour. Attempt to access private member variable minute. Compiling. Fig06 06.cpp D:\Fig06 06.cpp(15) : error C2248: 'hour' : cannot access private member declared in class 'Time' D:\Fig6 06\time1.h(18) : see declaration of 'hour' D:\Fig06 06.cpp(18) : error C2248: 'minute' : cannot access private member declared in class 'Time' D:\time1.h(19) : see declaration of 'minute' Error executing cl.exe. test.exe - 2 error(s), 0 warning(s)
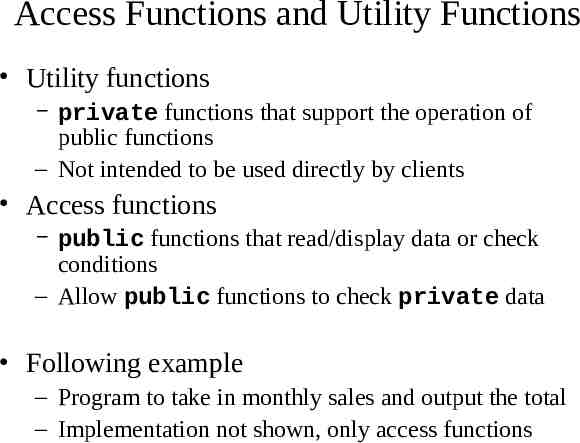
Access Functions and Utility Functions Utility functions – private functions that support the operation of public functions – Not intended to be used directly by clients Access functions – public functions that read/display data or check conditions – Allow public functions to check private data Following example – Program to take in monthly sales and output the total – Implementation not shown, only access functions
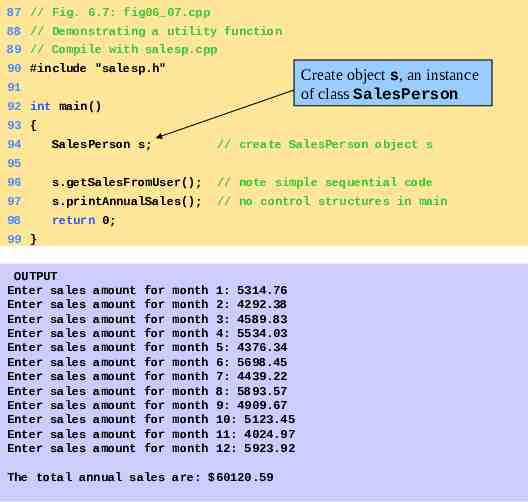
87 // Fig. 6.7: fig06 07.cpp 88 // Demonstrating a utility function 89 // Compile with salesp.cpp 90 #include "salesp.h" Create object s, an instance of class SalesPerson 91 92 int main() 93 { 94 SalesPerson s; // create SalesPerson object s 96 s.getSalesFromUser(); // note simple sequential code 97 s.printAnnualSales(); // no control structures in main 98 return 0; 95 99 } OUTPUT Enter sales Enter sales Enter sales Enter sales Enter sales Enter sales Enter sales Enter sales Enter sales Enter sales Enter sales Enter sales amount amount amount amount amount amount amount amount amount amount amount amount for for for for for for for for for for for for month month month month month month month month month month month month 1: 5314.76 2: 4292.38 3: 4589.83 4: 5534.03 5: 4376.34 6: 5698.45 7: 4439.22 8: 5893.57 9: 4909.67 10: 5123.45 11: 4024.97 12: 5923.92 The total annual sales are: 60120.59
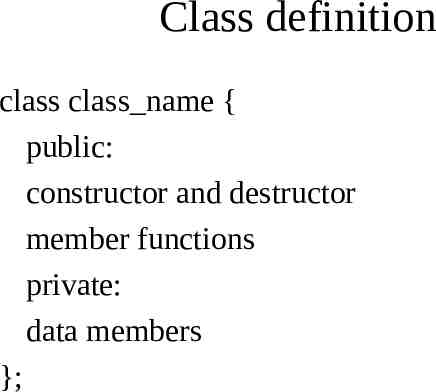
Class definition class class name { public: constructor and destructor member functions private: data members };
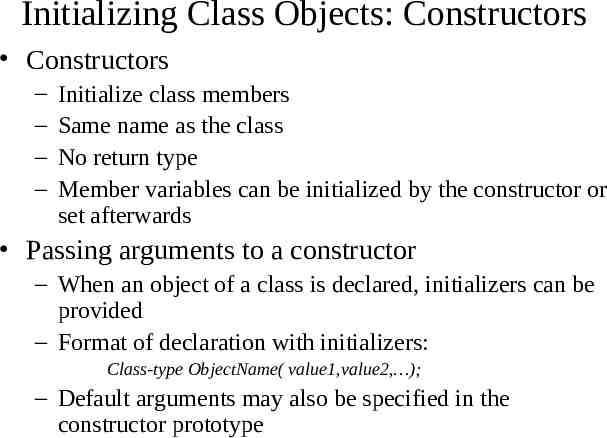
Initializing Class Objects: Constructors Constructors – – – – Initialize class members Same name as the class No return type Member variables can be initialized by the constructor or set afterwards Passing arguments to a constructor – When an object of a class is declared, initializers can be provided – Format of declaration with initializers: Class-type ObjectName( value1,value2, ); – Default arguments may also be specified in the constructor prototype
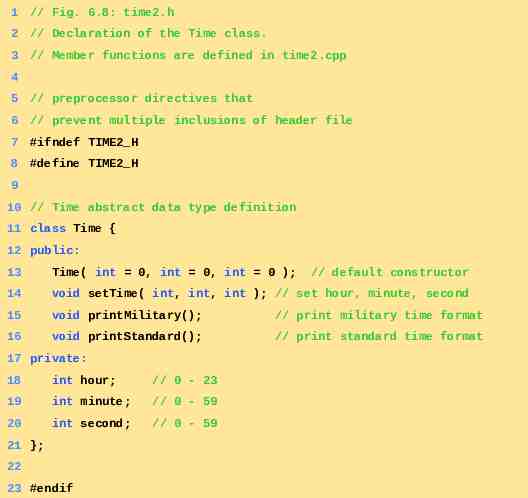
1 // Fig. 6.8: time2.h 2 // Declaration of the Time class. 3 // Member functions are defined in time2.cpp 4 5 // preprocessor directives that 6 // prevent multiple inclusions of header file 7 #ifndef TIME2 H 8 #define TIME2 H 9 10 // Time abstract data type definition 11 class Time { 12 public: 13 Time( int 0, int 0, int 0 ); 14 void setTime( int, int, int ); // set hour, minute, second 15 void printMilitary(); // print military time format 16 void printStandard(); // print standard time format 17 private: 18 int hour; // 0 - 23 19 int minute; // 0 - 59 20 int second; // 0 - 59 21 }; 22 23 #endif // default constructor
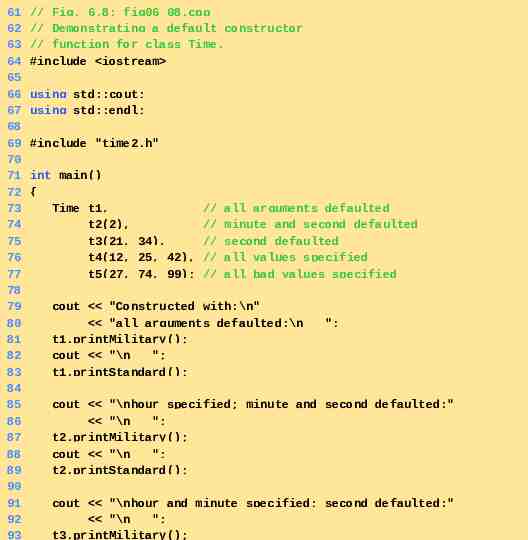
61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 // Fig. 6.8: fig06 08.cpp // Demonstrating a default constructor // function for class Time. #include iostream using std::cout; using std::endl; #include "time2.h" int main() { Time t1, // t2(2), // t3(21, 34), // t4(12, 25, 42), // t5(27, 74, 99); // all arguments defaulted minute and second defaulted second defaulted all values specified all bad values specified cout "Constructed with:\n" "all arguments defaulted:\n t1.printMilitary(); cout "\n "; t1.printStandard(); "; cout "\nhour specified; minute and second defaulted:" "\n "; t2.printMilitary(); cout "\n "; t2.printStandard(); cout "\nhour and minute specified; second defaulted:" "\n "; t3.printMilitary();
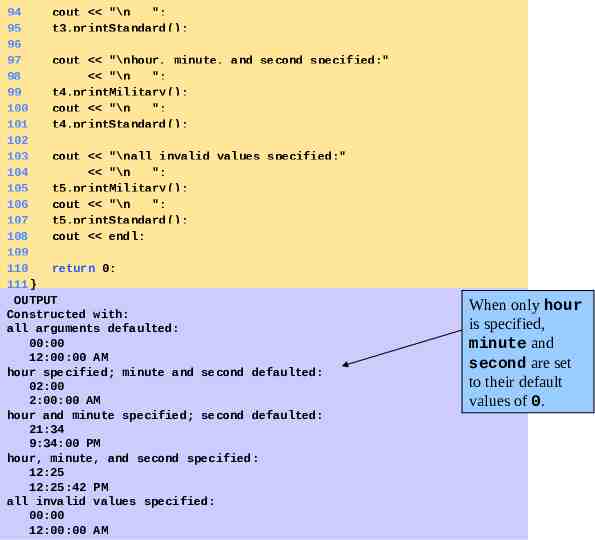
94 cout "\n "; 95 t3.printStandard(); 96 97 cout "\nhour, minute, and second specified:" 98 "\n "; 99 t4.printMilitary(); 100 cout "\n "; 101 t4.printStandard(); 102 103 cout "\nall invalid values specified:" 104 "\n "; 105 t5.printMilitary(); 106 cout "\n "; 107 t5.printStandard(); 108 cout endl; 109 110 return 0; 111 } OUTPUT Constructed with: all arguments defaulted: 00:00 12:00:00 AM hour specified; minute and second defaulted: 02:00 2:00:00 AM hour and minute specified; second defaulted: 21:34 9:34:00 PM hour, minute, and second specified: 12:25 12:25:42 PM all invalid values specified: 00:00 12:00:00 AM When only hour is specified, minute and second are set to their default values of 0.
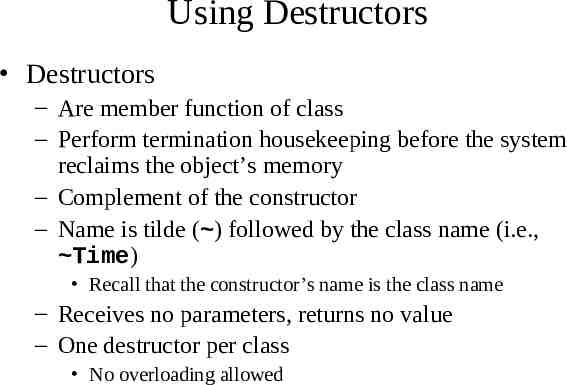
Using Destructors Destructors – Are member function of class – Perform termination housekeeping before the system reclaims the object’s memory – Complement of the constructor – Name is tilde ( ) followed by the class name (i.e., Time) Recall that the constructor’s name is the class name – Receives no parameters, returns no value – One destructor per class No overloading allowed
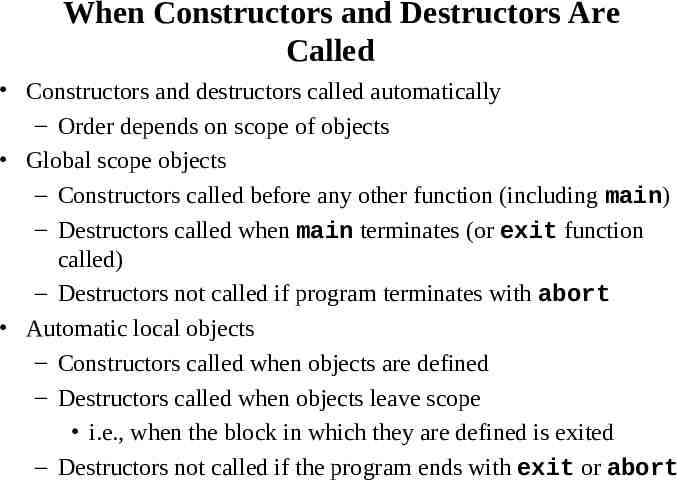
When Constructors and Destructors Are Called Constructors and destructors called automatically – Order depends on scope of objects Global scope objects – Constructors called before any other function (including main) – Destructors called when main terminates (or exit function called) – Destructors not called if program terminates with abort Automatic local objects – Constructors called when objects are defined – Destructors called when objects leave scope i.e., when the block in which they are defined is exited – Destructors not called if the program ends with exit or abort
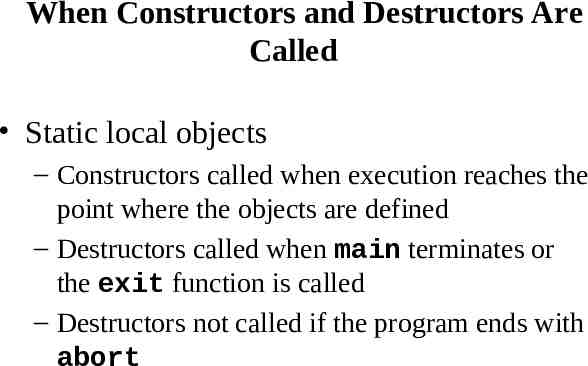
When Constructors and Destructors Are Called Static local objects – Constructors called when execution reaches the point where the objects are defined – Destructors called when main terminates or the exit function is called – Destructors not called if the program ends with abort
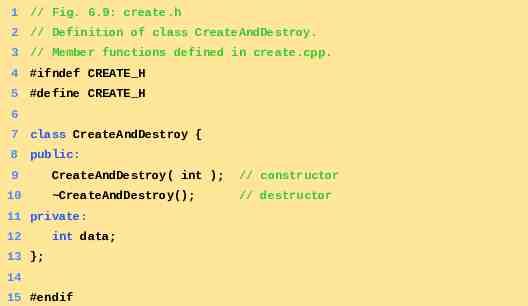
1 // Fig. 6.9: create.h 2 // Definition of class CreateAndDestroy. 3 // Member functions defined in create.cpp. 4 #ifndef CREATE H 5 #define CREATE H 6 7 class CreateAndDestroy { 8 public: 9 10 CreateAndDestroy( int ); // constructor CreateAndDestroy(); // destructor 11 private: 12 int data; 13 }; 14 15 #endif
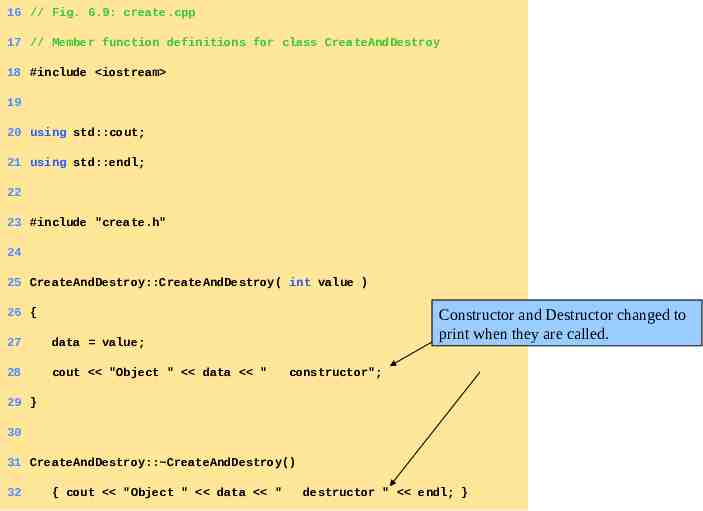
16 // Fig. 6.9: create.cpp 17 // Member function definitions for class CreateAndDestroy 18 #include iostream 19 20 using std::cout; 21 using std::endl; 22 23 #include "create.h" 24 25 CreateAndDestroy::CreateAndDestroy( int value ) Constructor and Destructor changed to print when they are called. 26 { 27 data value; 28 cout "Object " data " constructor"; 29 } 30 31 CreateAndDestroy:: CreateAndDestroy() 32 { cout "Object " data " destructor " endl; }
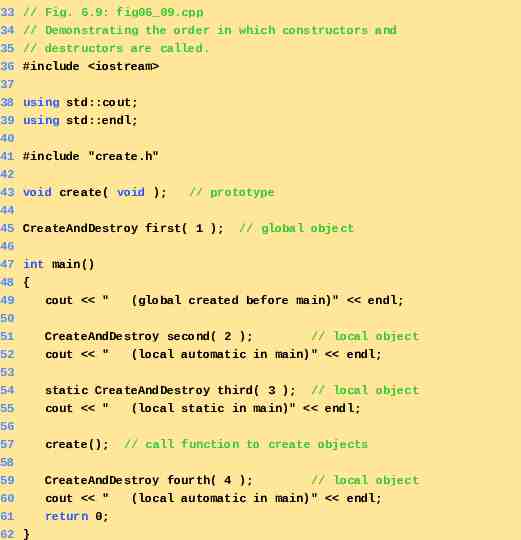
33 // Fig. 6.9: fig06 09.cpp 34 // Demonstrating the order in which constructors and 35 // destructors are called. 36 #include iostream 37 38 using std::cout; 39 using std::endl; 40 41 #include "create.h" 42 43 void create( void ); // prototype 44 45 CreateAndDestroy first( 1 ); // global object 46 47 int main() 48 { 49 cout " (global created before main)" endl; 50 51 52 CreateAndDestroy second( 2 ); // local object cout " (local automatic in main)" endl; 53 54 static CreateAndDestroy third( 3 ); // local object 55 56 cout " (local static in main)" endl; 57 create(); // call function to create objects 58 59 60 CreateAndDestroy fourth( 4 ); // local object cout " (local automatic in main)" endl; 61 return 0; 62 }
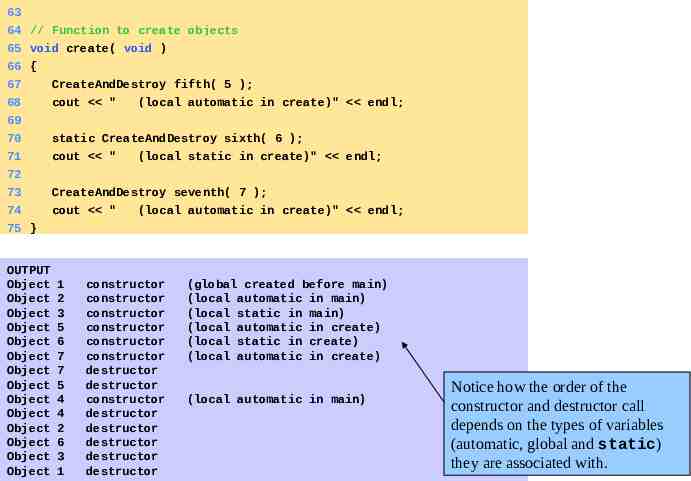
63 64 // Function to create objects 65 void create( void ) 66 { 67 CreateAndDestroy fifth( 5 ); 68 69 cout " 70 static CreateAndDestroy sixth( 6 ); 71 cout " 72 73 CreateAndDestroy seventh( 7 ); 74 cout " (local automatic in create)" endl; (local static in create)" endl; (local automatic in create)" endl; 75 } OUTPUT Object Object Object Object Object Object Object Object Object Object Object Object Object Object 1 2 3 5 6 7 7 5 4 4 2 6 3 1 constructor constructor constructor constructor constructor constructor destructor destructor constructor destructor destructor destructor destructor destructor (global created before main) (local automatic in main) (local static in main) (local automatic in create) (local static in create) (local automatic in create) (local automatic in main) Notice how the order of the constructor and destructor call depends on the types of variables (automatic, global and static) they are associated with.
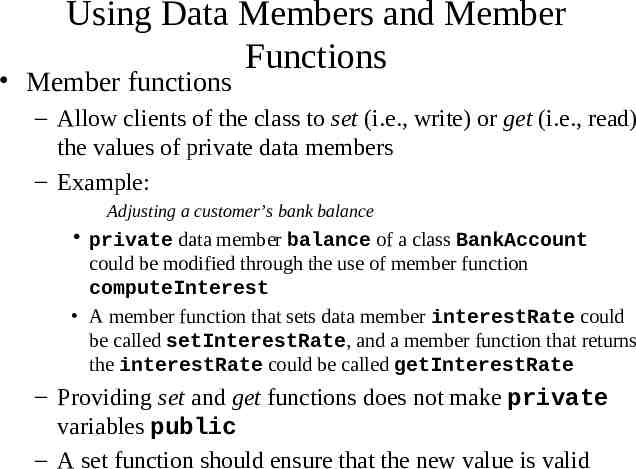
Using Data Members and Member Functions Member functions – Allow clients of the class to set (i.e., write) or get (i.e., read) the values of private data members – Example: Adjusting a customer’s bank balance private data member balance of a class BankAccount could be modified through the use of member function computeInterest A member function that sets data member interestRate could be called setInterestRate, and a member function that returns the interestRate could be called getInterestRate – Providing set and get functions does not make private variables public – A set function should ensure that the new value is valid
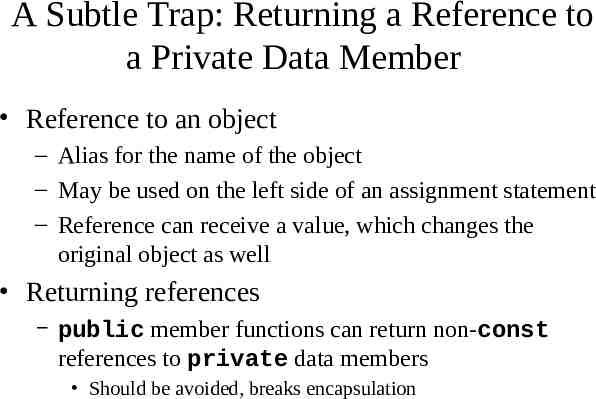
A Subtle Trap: Returning a Reference to a Private Data Member Reference to an object – Alias for the name of the object – May be used on the left side of an assignment statement – Reference can receive a value, which changes the original object as well Returning references – public member functions can return non-const references to private data members Should be avoided, breaks encapsulation
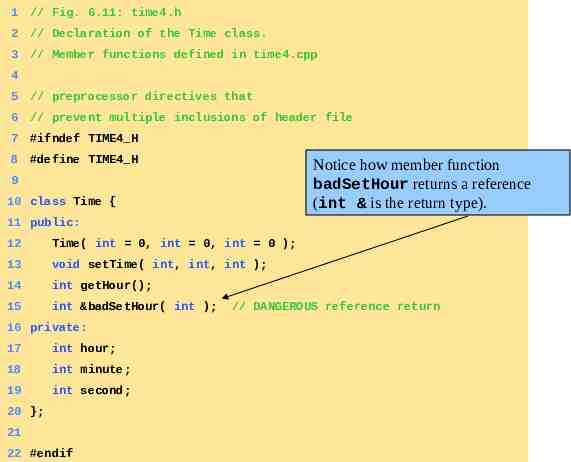
1 // Fig. 6.11: time4.h 2 // Declaration of the Time class. 3 // Member functions defined in time4.cpp 4 5 // preprocessor directives that 6 // prevent multiple inclusions of header file 7 #ifndef TIME4 H 8 #define TIME4 H Notice how member function badSetHour returns a reference (int & is the return type). 9 10 class Time { 11 public: 12 Time( int 0, int 0, int 0 ); 13 void setTime( int, int, int ); 14 int getHour(); 15 int &badSetHour( int ); 16 private: 17 int hour; 18 int minute; 19 int second; 20 }; 21 22 #endif // DANGEROUS reference return
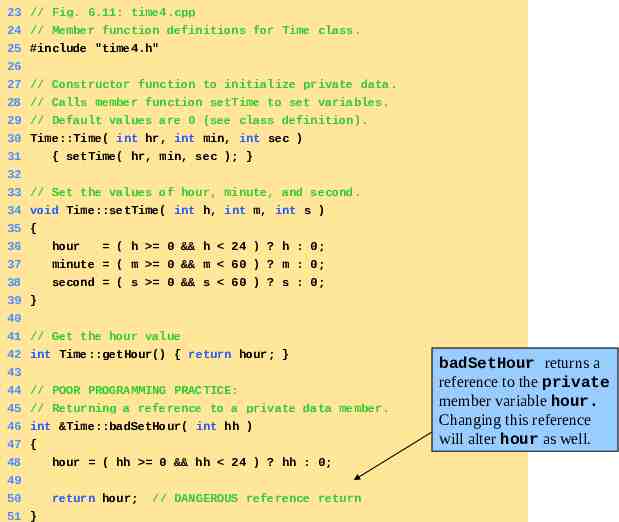
23 // Fig. 6.11: time4.cpp 24 // Member function definitions for Time class. 25 #include "time4.h" 26 27 // Constructor function to initialize private data. 28 // Calls member function setTime to set variables. 29 // Default values are 0 (see class definition). 30 Time::Time( int hr, int min, int sec ) 31 { setTime( hr, min, sec ); } 32 33 // Set the values of hour, minute, and second. 34 void Time::setTime( int h, int m, int s ) 35 { 36 37 hour ( h 0 && h 24 ) ? h : 0; minute ( m 0 && m 60 ) ? m : 0; 38 second ( s 0 && s 60 ) ? s : 0; 39 } 40 1. Load header 41 // Get the hour value 42 int Time::getHour() { return hour; } 43 44 // POOR PROGRAMMING PRACTICE: badSetHour returns a reference to the private member variable hour. Changing this reference will alter hour as well. 1.1 Function definitions 45 // Returning a reference to a private data member. 46 int &Time::badSetHour( int hh ) 47 { 48 hour ( hh 0 && hh 24 ) ? hh : 0; 49 50 return hour; 51 } // DANGEROUS reference return
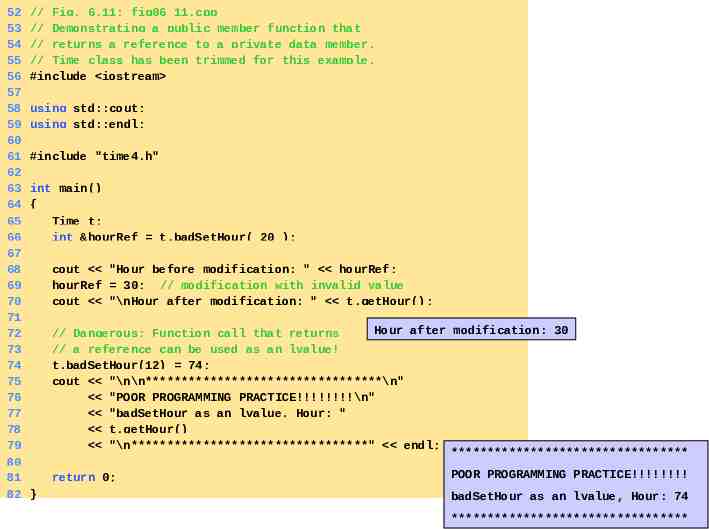
52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 // Fig. 6.11: fig06 11.cpp // Demonstrating a public member function that // returns a reference to a private data member. // Time class has been trimmed for this example. #include iostream using std::cout; using std::endl; #include "time4.h" int main() { Time t; int &hourRef t.badSetHour( 20 ); cout "Hour before modification: " hourRef; hourRef 30; // modification with invalid value cout "\nHour after modification: " t.getHour(); Hour after modification: 30 // Dangerous: Function call that returns // a reference can be used as an lvalue! t.badSetHour(12) 74; cout "\n\n*********************************\n" "POOR PROGRAMMING PRACTICE!!!!!!!!\n" "badSetHour as an lvalue, Hour: " t.getHour() "\n*********************************" endl; ********************************* return 0; } POOR PROGRAMMING PRACTICE!!!!!!!! badSetHour as an lvalue, Hour: 74 *********************************
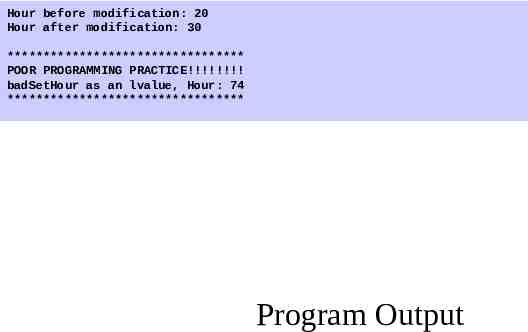
Hour before modification: 20 Hour after modification: 30 ********************************* POOR PROGRAMMING PRACTICE!!!!!!!! badSetHour as an lvalue, Hour: 74 ********************************* Program Output
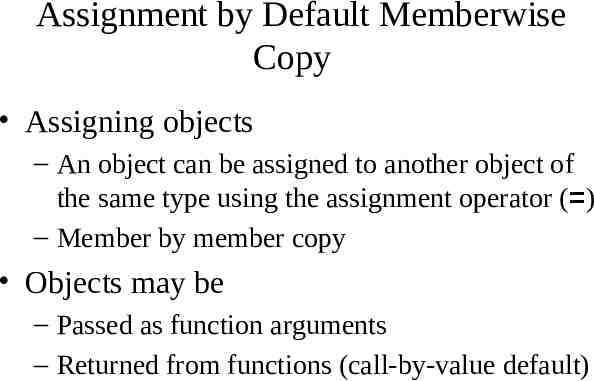
Assignment by Default Memberwise Copy Assigning objects – An object can be assigned to another object of the same type using the assignment operator ( ) – Member by member copy Objects may be – Passed as function arguments – Returned from functions (call-by-value default)
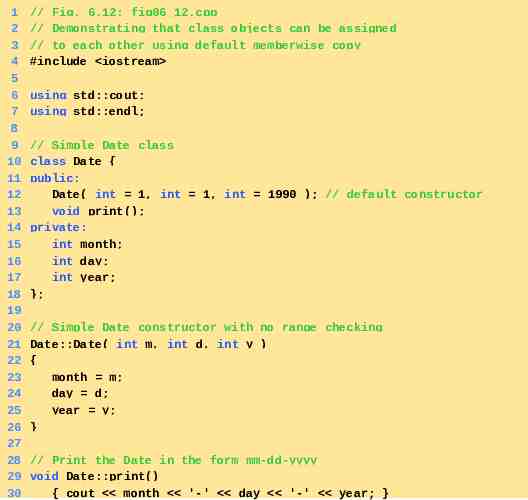
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 // Fig. 6.12: fig06 12.cpp // Demonstrating that class objects can be assigned // to each other using default memberwise copy #include iostream using std::cout; using std::endl; // Simple Date class class Date { public: Date( int 1, int 1, int 1990 ); // default constructor void print(); private: int month; int day; int year; }; // Simple Date constructor with no range checking Date::Date( int m, int d, int y ) { month m; day d; year y; } // Print the Date in the form mm-dd-yyyy void Date::print() { cout month '-' day '-' year; }
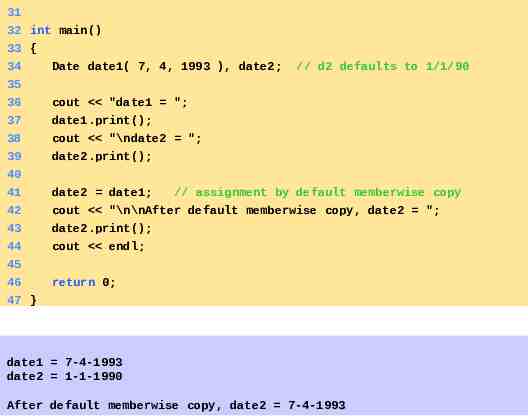
31 32 int main() 33 { 34 Date date1( 7, 4, 1993 ), date2; // d2 defaults to 1/1/90 35 36 37 cout "date1 "; date1.print(); 38 cout "\ndate2 "; 39 date2.print(); 40 41 date2 date1; 42 cout "\n\nAfter default memberwise copy, date2 "; 43 date2.print(); 44 45 cout endl; 46 return 0; // assignment by default memberwise copy 47 } date1 7-4-1993 date2 1-1-1990 After default memberwise copy, date2 7-4-1993
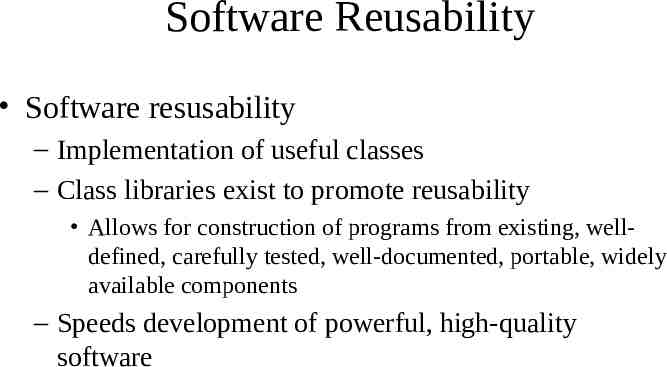
Software Reusability Software resusability – Implementation of useful classes – Class libraries exist to promote reusability Allows for construction of programs from existing, welldefined, carefully tested, well-documented, portable, widely available components – Speeds development of powerful, high-quality software