CAP6135: Malware and Software Vulnerability Analysis Buffer
41 Slides334.00 KB
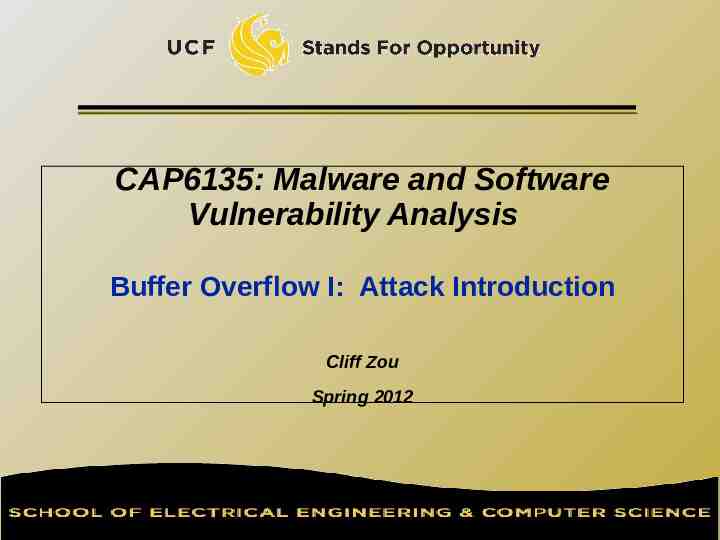
CAP6135: Malware and Software Vulnerability Analysis Buffer Overflow I: Attack Introduction Cliff Zou Spring 2012
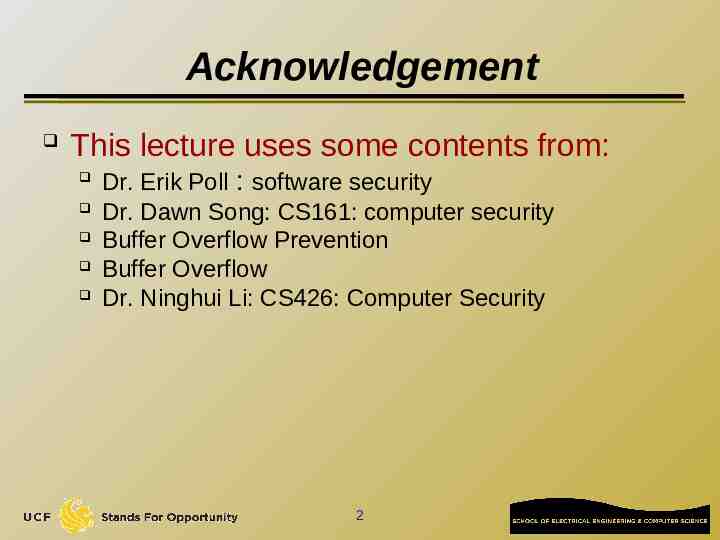
Acknowledgement This lecture uses some contents from: Dr. Erik Poll : software security Dr. Dawn Song: CS161: computer security Buffer Overflow Prevention Buffer Overflow Dr. Ninghui Li: CS426: Computer Security 2
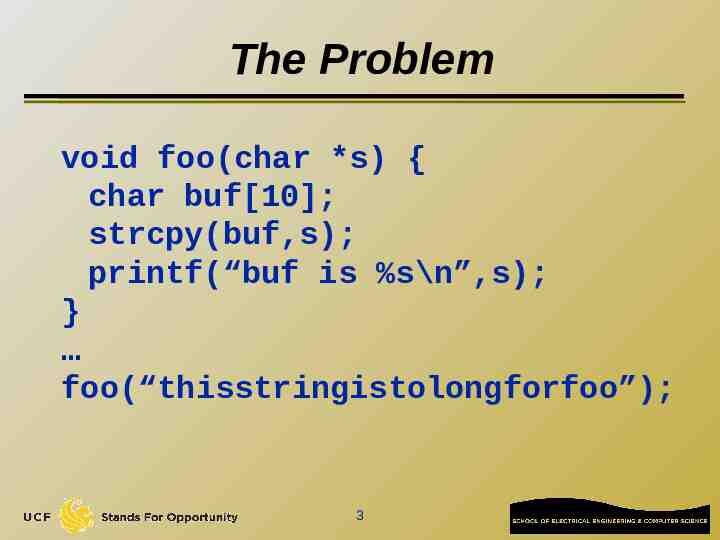
The Problem void foo(char *s) { char buf[10]; strcpy(buf,s); printf(“buf is %s\n”,s); } foo(“thisstringistolongforfoo”); 3
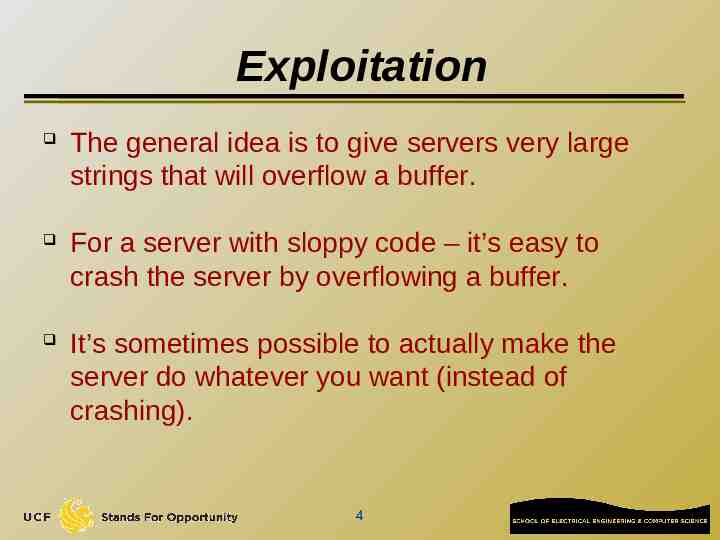
Exploitation The general idea is to give servers very large strings that will overflow a buffer. For a server with sloppy code – it’s easy to crash the server by overflowing a buffer. It’s sometimes possible to actually make the server do whatever you want (instead of crashing). 4
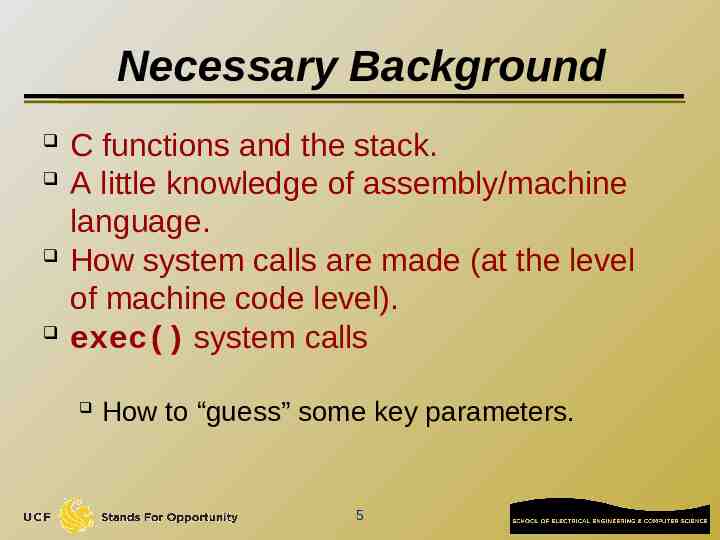
Necessary Background C functions and the stack. A little knowledge of assembly/machine language. How system calls are made (at the level of machine code level). exec() system calls How to “guess” some key parameters. 5
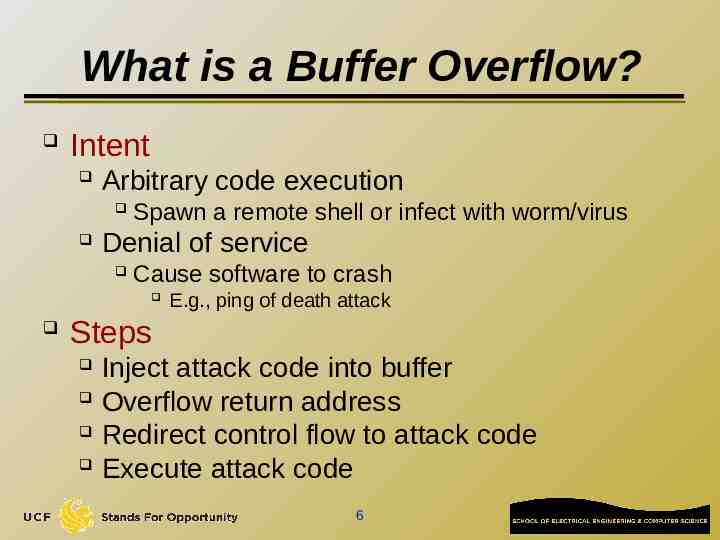
What is a Buffer Overflow? Intent Arbitrary code execution Spawn a remote shell or infect with worm/virus Denial of service Cause software to crash E.g., ping of death attack Steps Inject attack code into buffer Overflow return address Redirect control flow to attack code Execute attack code 6
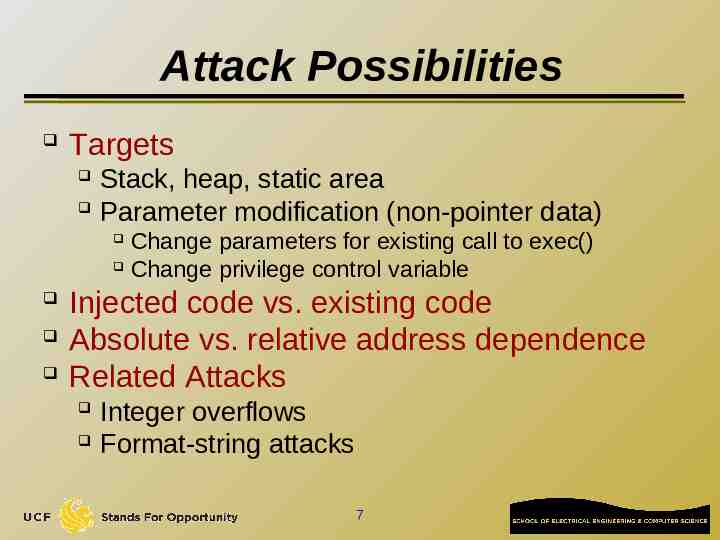
Attack Possibilities Targets Stack, heap, static area Parameter modification (non-pointer data) Change parameters for existing call to exec() Change privilege control variable Injected code vs. existing code Absolute vs. relative address dependence Related Attacks Integer overflows Format-string attacks 7
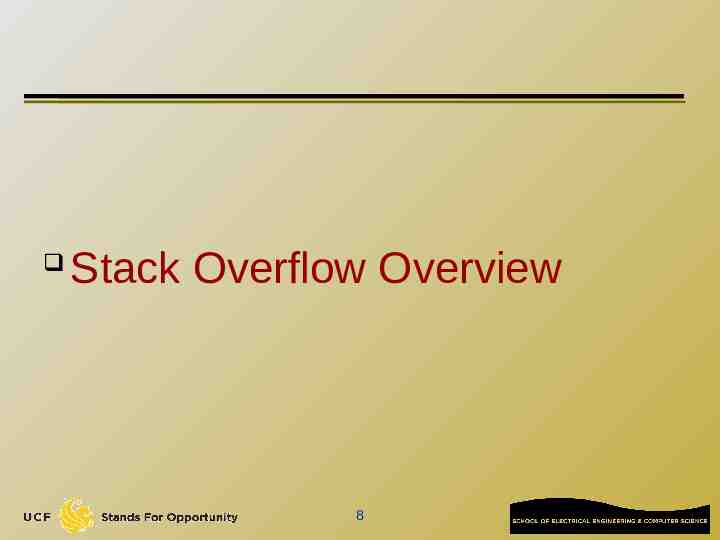
Stack Overflow Overview 8
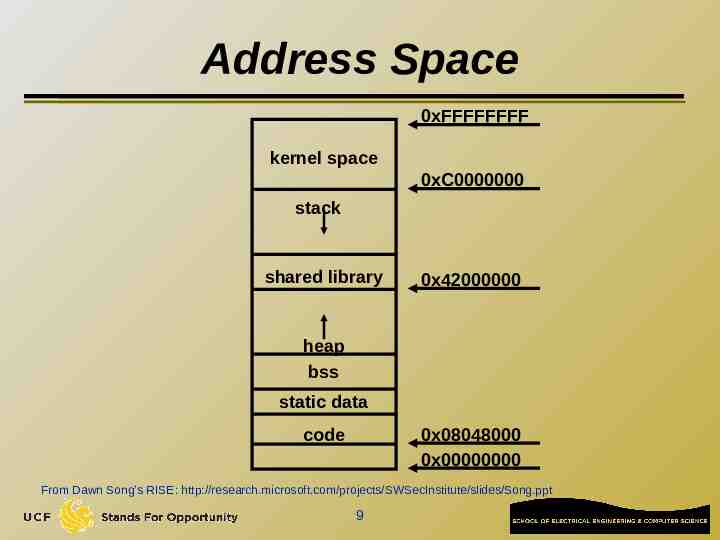
Address Space 0xFFFFFFFF kernel space 0xC0000000 stack shared library 0x42000000 heap bss static data code 0x08048000 0x00000000 From Dawn Song’s RISE: http://research.microsoft.com/projects/SWSecInstitute/slides/Song.ppt 9
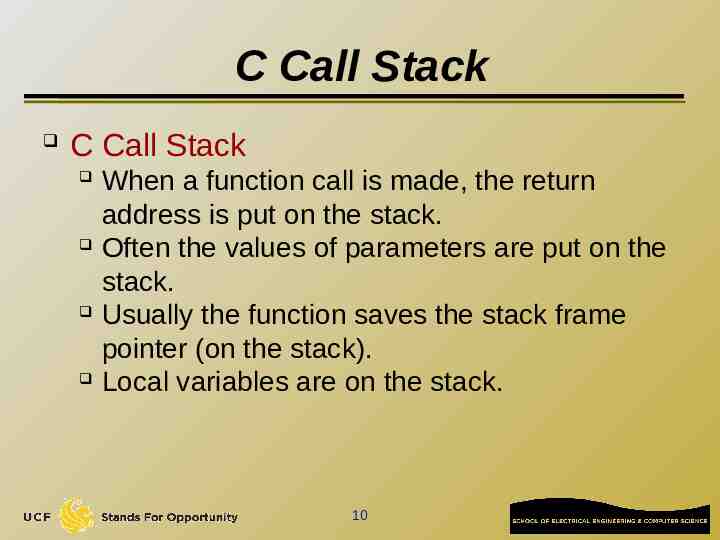
C Call Stack C Call Stack When a function call is made, the return address is put on the stack. Often the values of parameters are put on the stack. Usually the function saves the stack frame pointer (on the stack). Local variables are on the stack. 10
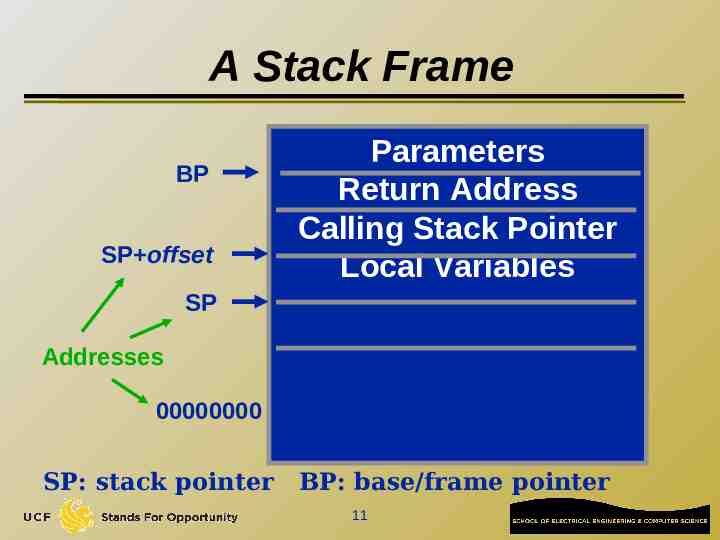
A Stack Frame BP SP offset Parameters Return Address Calling Stack Pointer Local Variables SP Addresses 00000000 SP: stack pointer BP: base/frame pointer 11
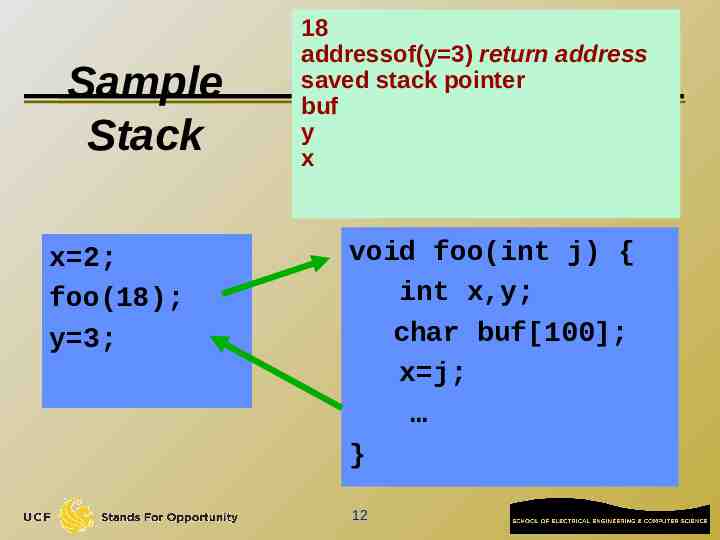
Sample Stack x 2; foo(18); y 3; 18 addressof(y 3) return address saved stack pointer buf y x void foo(int j) { int x,y; char buf[100]; x j; } 12
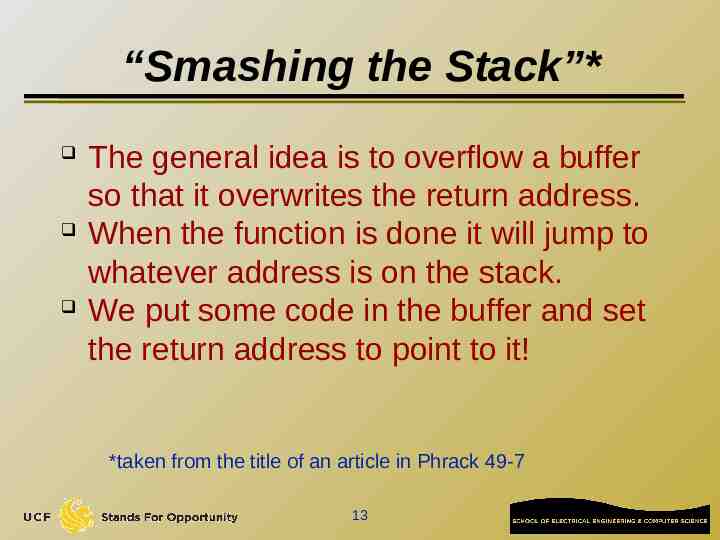
“Smashing the Stack”* The general idea is to overflow a buffer so that it overwrites the return address. When the function is done it will jump to whatever address is on the stack. We put some code in the buffer and set the return address to point to it! *taken from the title of an article in Phrack 49-7 13
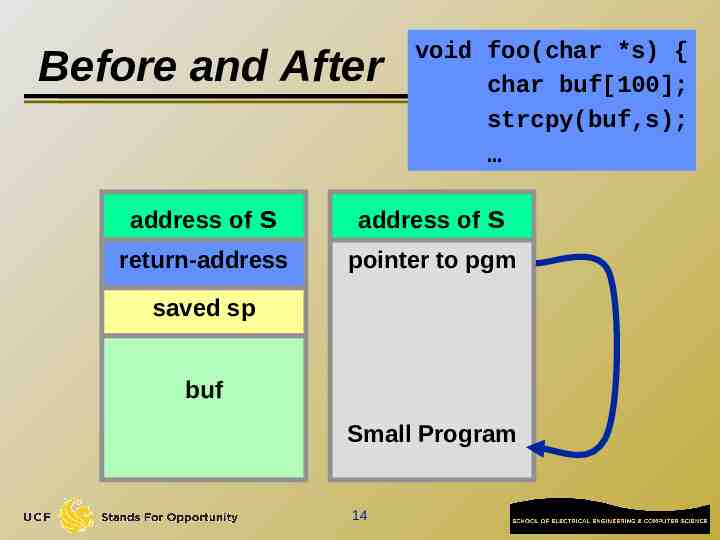
Before and After void foo(char *s) { char buf[100]; strcpy(buf,s); address of s address of s return-address pointer to pgm saved sp buf Small Program 14
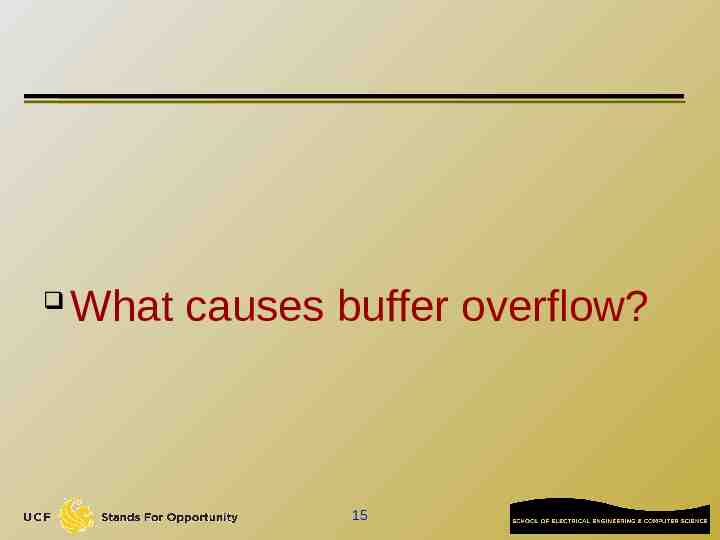
What causes buffer overflow? 15
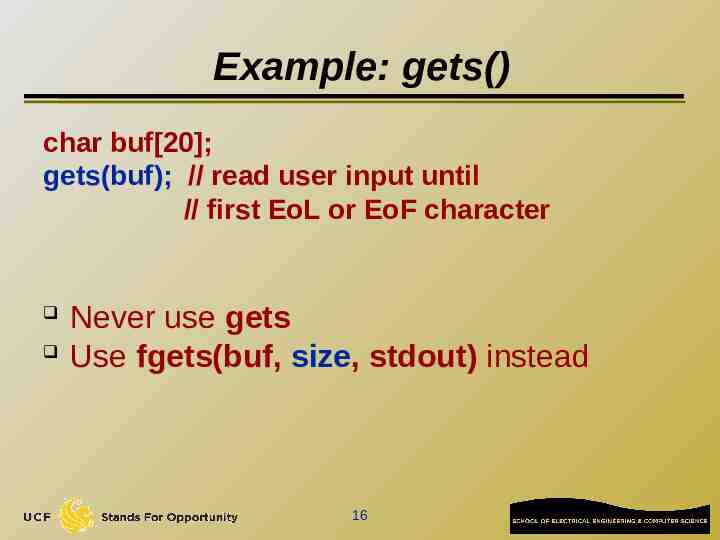
Example: gets() char buf[20]; gets(buf); // read user input until // first EoL or EoF character Never use gets Use fgets(buf, size, stdout) instead 16
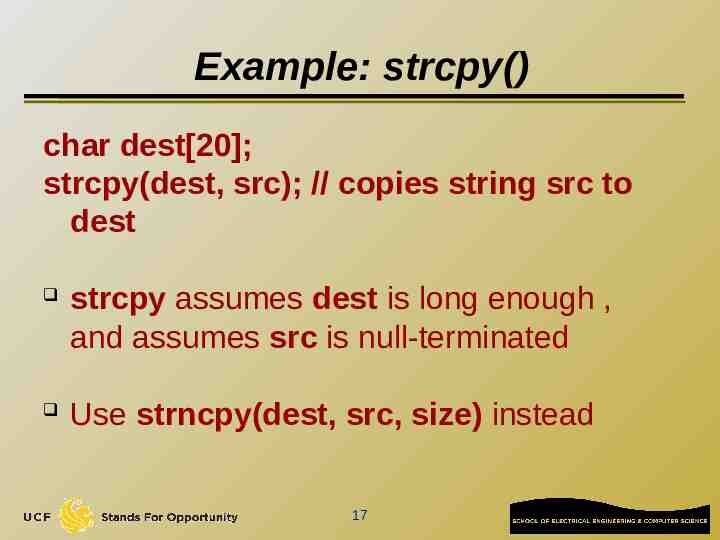
Example: strcpy() char dest[20]; strcpy(dest, src); // copies string src to dest strcpy assumes dest is long enough , and assumes src is null-terminated Use strncpy(dest, src, size) instead 17
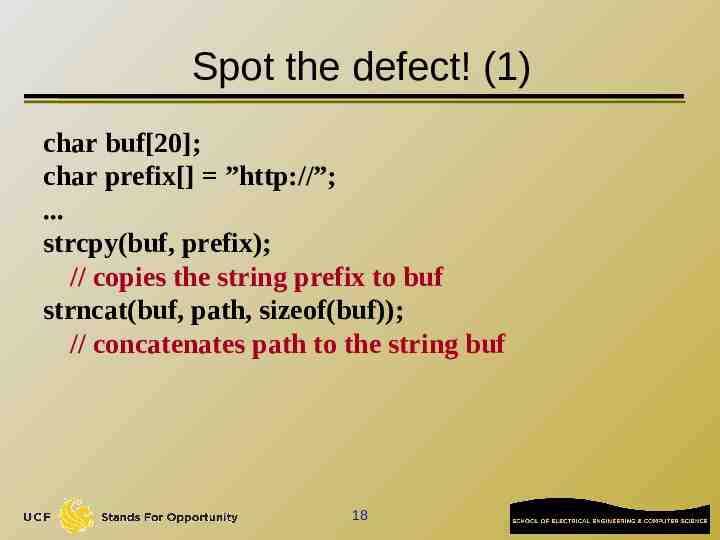
Spot the defect! (1) char buf[20]; char prefix[] ”http://”; . strcpy(buf, prefix); // copies the string prefix to buf strncat(buf, path, sizeof(buf)); // concatenates path to the string buf 18
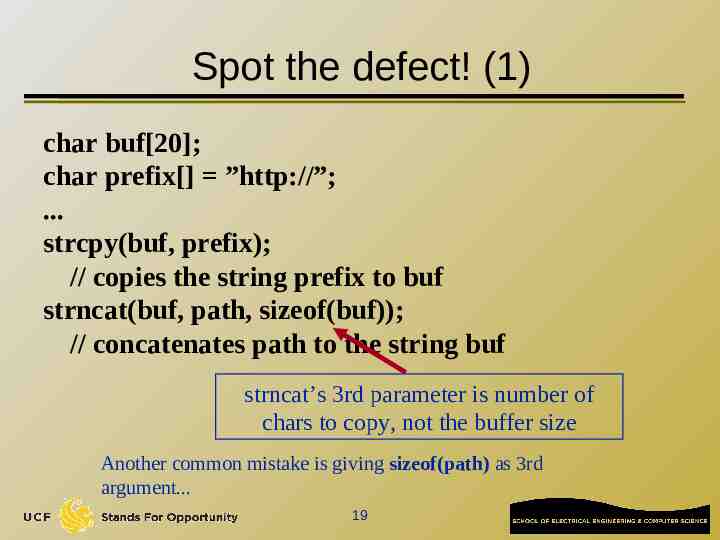
Spot the defect! (1) char buf[20]; char prefix[] ”http://”; . strcpy(buf, prefix); // copies the string prefix to buf strncat(buf, path, sizeof(buf)); // concatenates path to the string buf strncat’s 3rd parameter is number of chars to copy, not the buffer size Another common mistake is giving sizeof(path) as 3rd argument. 19
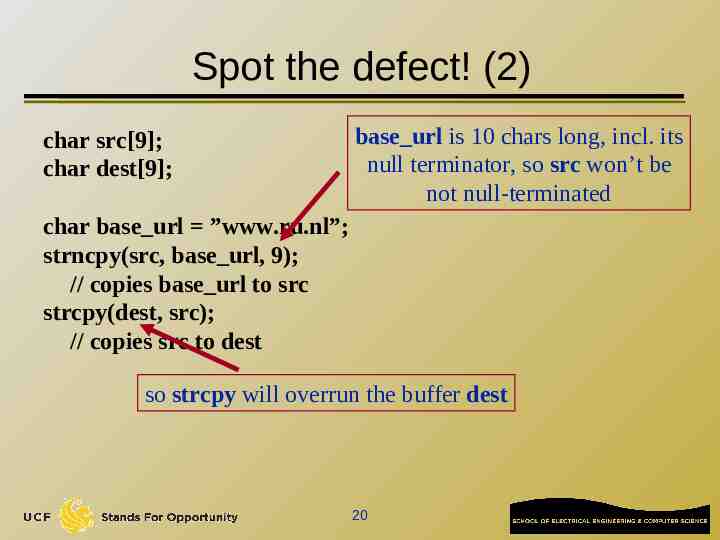
Spot the defect! (2) char src[9]; char dest[9]; base url is 10 chars long, incl. its null terminator, so src won’t be not null-terminated char base url ”www.ru.nl”; strncpy(src, base url, 9); // copies base url to src strcpy(dest, src); // copies src to dest so strcpy will overrun the buffer dest 20
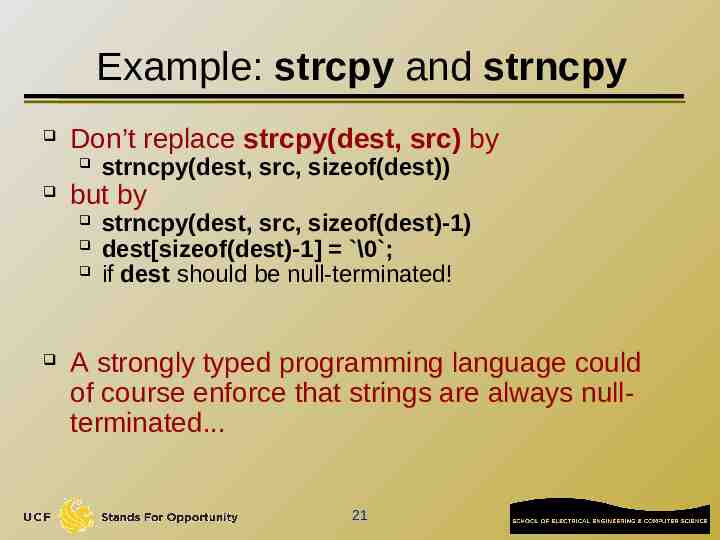
Example: strcpy and strncpy Don’t replace strcpy(dest, src) by but by strncpy(dest, src, sizeof(dest)) strncpy(dest, src, sizeof(dest)-1) dest[sizeof(dest)-1] \0 ; if dest should be null-terminated! A strongly typed programming language could of course enforce that strings are always nullterminated. 21
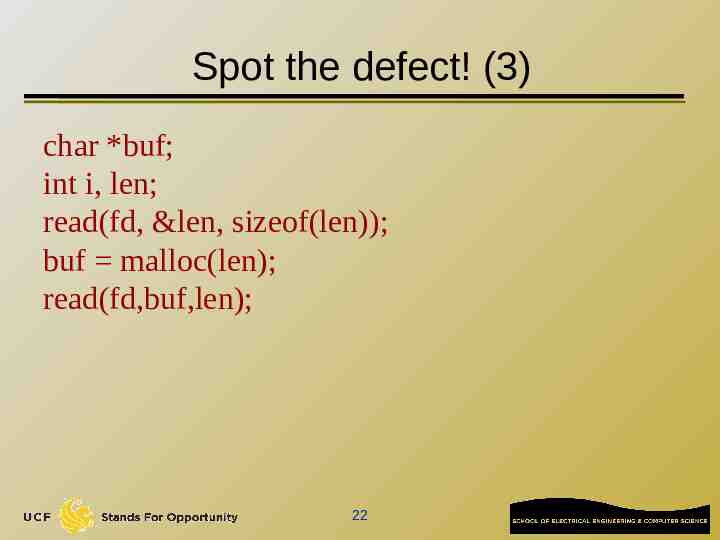
Spot the defect! (3) char *buf; int i, len; read(fd, &len, sizeof(len)); buf malloc(len); read(fd,buf,len); 22
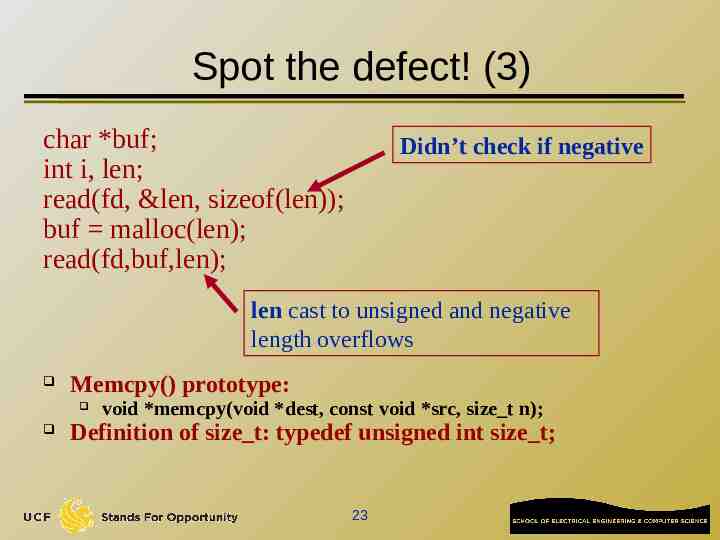
Spot the defect! (3) char *buf; int i, len; read(fd, &len, sizeof(len)); buf malloc(len); read(fd,buf,len); Didn’t check if negative len cast to unsigned and negative length overflows Memcpy() prototype: void *memcpy(void *dest, const void *src, size t n); Definition of size t: typedef unsigned int size t; 23
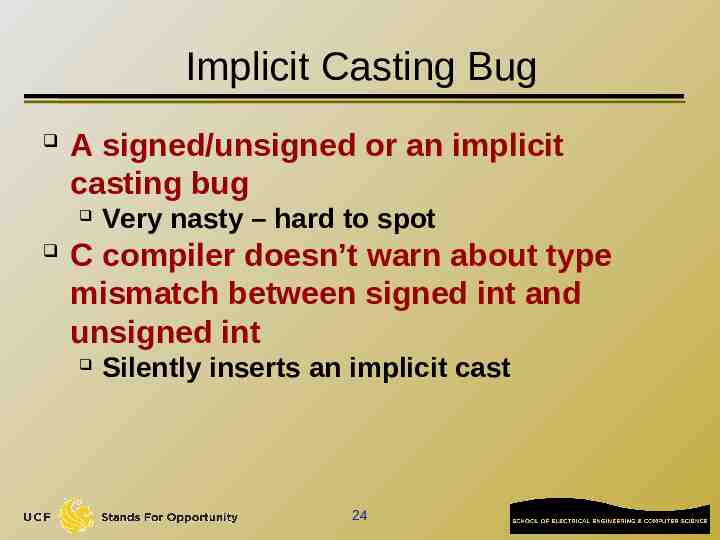
Implicit Casting Bug A signed/unsigned or an implicit casting bug Very nasty – hard to spot C compiler doesn’t warn about type mismatch between signed int and unsigned int Silently inserts an implicit cast 24
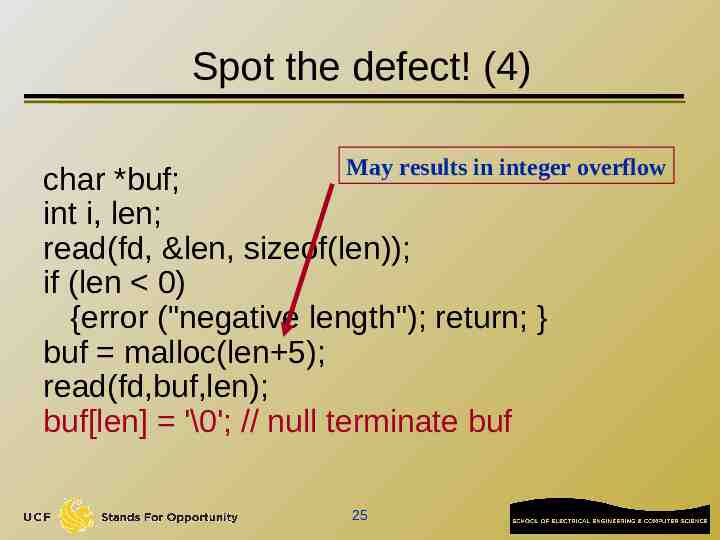
Spot the defect! (4) May results in integer overflow char *buf; int i, len; read(fd, &len, sizeof(len)); if (len 0) {error ("negative length"); return; } buf malloc(len 5); read(fd,buf,len); buf[len] '\0'; // null terminate buf 25
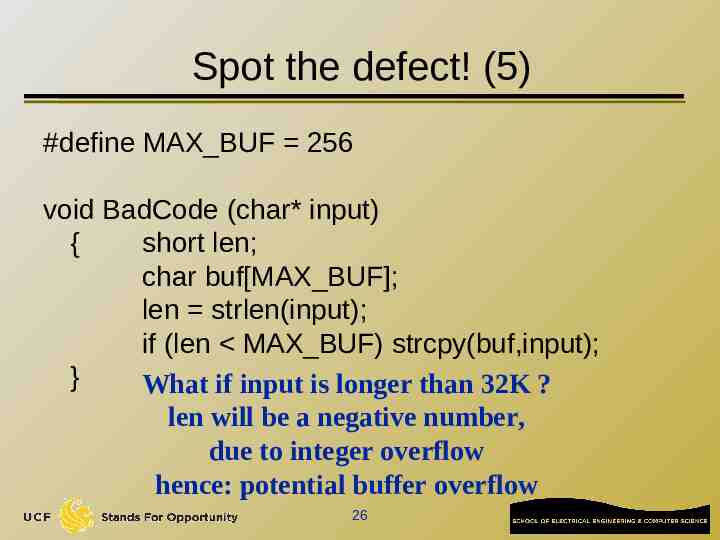
Spot the defect! (5) #define MAX BUF 256 void BadCode (char* input) { short len; char buf[MAX BUF]; len strlen(input); if (len MAX BUF) strcpy(buf,input); } What if input is longer than 32K ? len will be a negative number, due to integer overflow hence: potential buffer overflow 26
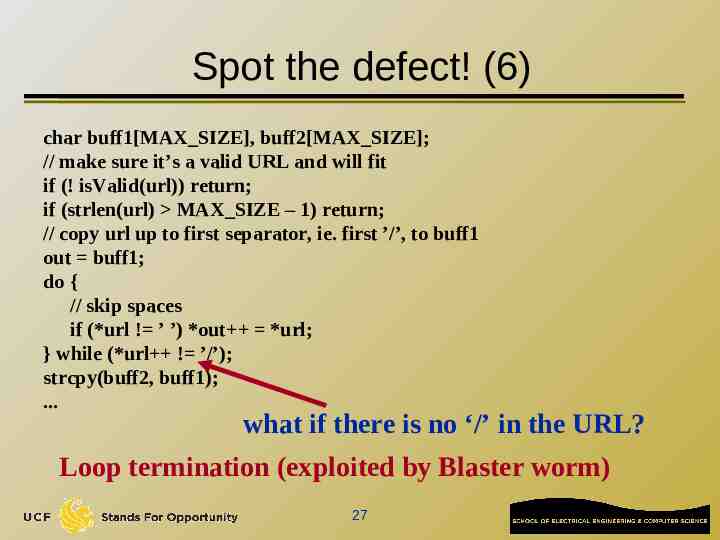
Spot the defect! (6) char buff1[MAX SIZE], buff2[MAX SIZE]; // make sure it’s a valid URL and will fit if (! isValid(url)) return; if (strlen(url) MAX SIZE – 1) return; // copy url up to first separator, ie. first ’/’, to buff1 out buff1; do { // skip spaces if (*url ! ’ ’) *out *url; } while (*url ! ’/’); strcpy(buff2, buff1); . what if there is no ‘/’ in the URL? Loop termination (exploited by Blaster worm) 27
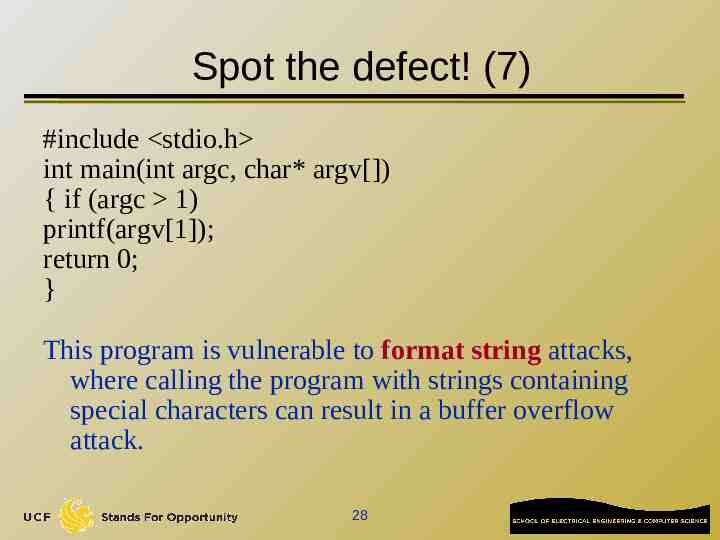
Spot the defect! (7) #include stdio.h int main(int argc, char* argv[]) { if (argc 1) printf(argv[1]); return 0; } This program is vulnerable to format string attacks, where calling the program with strings containing special characters can result in a buffer overflow attack. 28
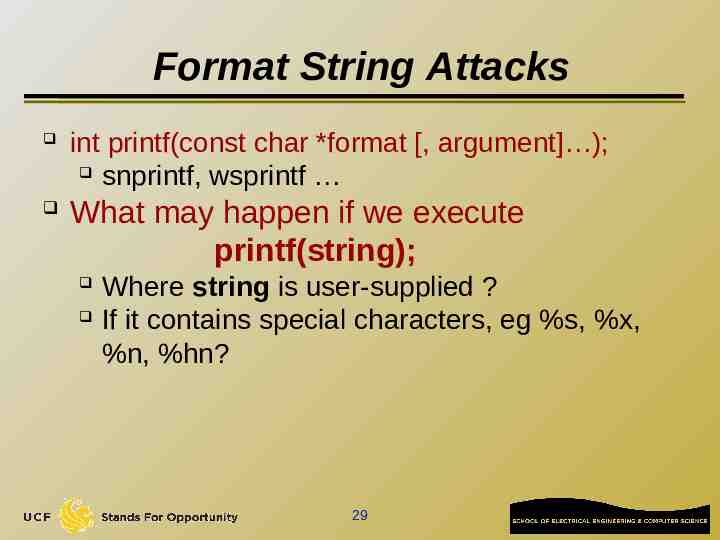
Format String Attacks int printf(const char *format [, argument] ); snprintf, wsprintf What may happen if we execute printf(string); Where string is user-supplied ? If it contains special characters, eg %s, %x, %n, %hn? 29
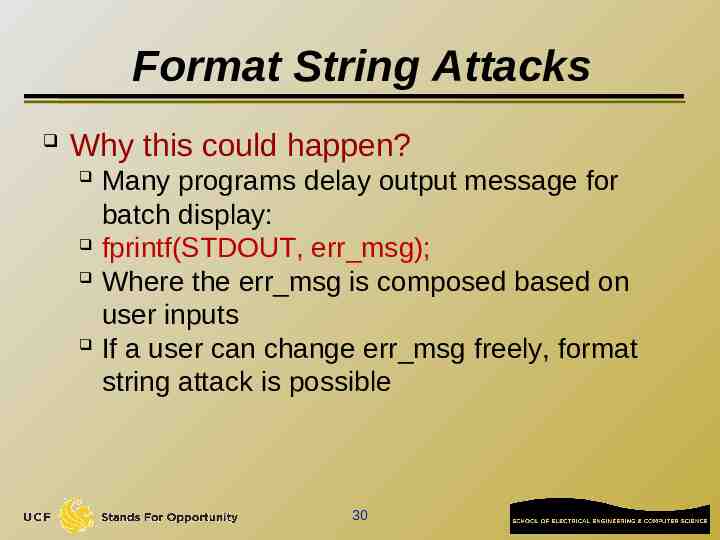
Format String Attacks Why this could happen? Many programs delay output message for batch display: fprintf(STDOUT, err msg); Where the err msg is composed based on user inputs If a user can change err msg freely, format string attack is possible 30
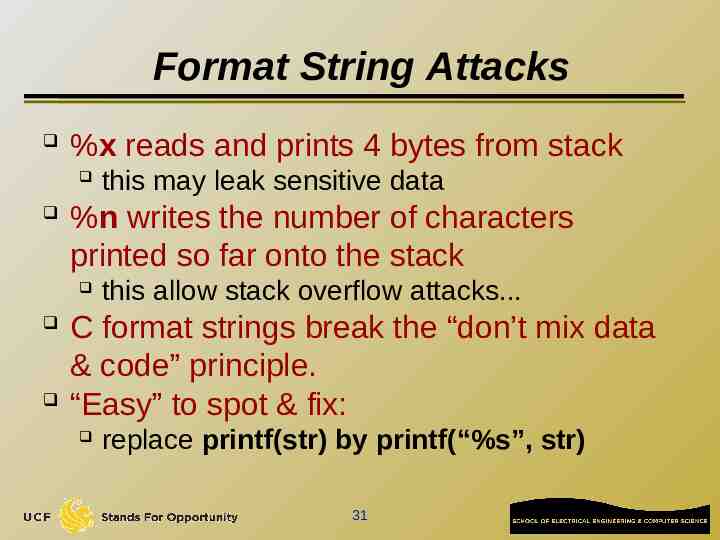
Format String Attacks %x reads and prints 4 bytes from stack %n writes the number of characters printed so far onto the stack this may leak sensitive data this allow stack overflow attacks. C format strings break the “don’t mix data & code” principle. “Easy” to spot & fix: replace printf(str) by printf(“%s”, str) 31
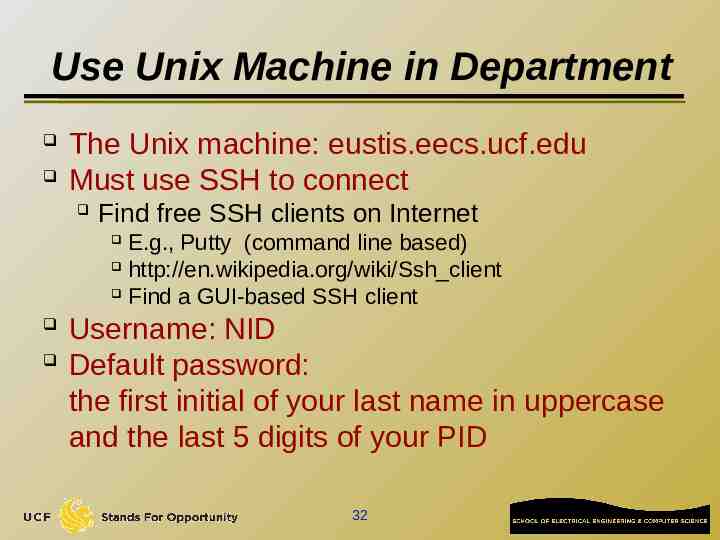
Use Unix Machine in Department The Unix machine: eustis.eecs.ucf.edu Must use SSH to connect Find free SSH clients on Internet E.g., Putty (command line based) http://en.wikipedia.org/wiki/Ssh client Find a GUI-based SSH client Username: NID Default password: the first initial of your last name in uppercase and the last 5 digits of your PID 32
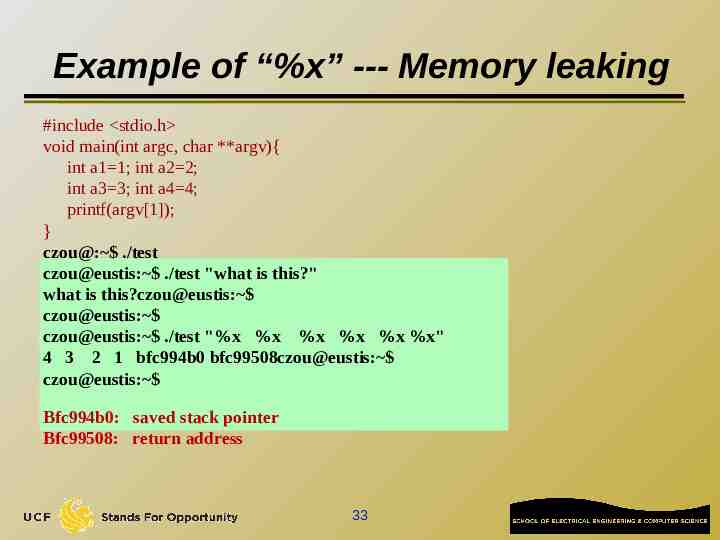
Example of “%x” --- Memory leaking #include stdio.h void main(int argc, char **argv){ int a1 1; int a2 2; int a3 3; int a4 4; printf(argv[1]); } czou@: ./test czou@eustis: ./test "what is this?" what is this?czou@eustis: czou@eustis: czou@eustis: ./test "%x %x %x %x %x %x" 4 3 2 1 bfc994b0 bfc99508czou@eustis: czou@eustis: Bfc994b0: saved stack pointer Bfc99508: return address 33
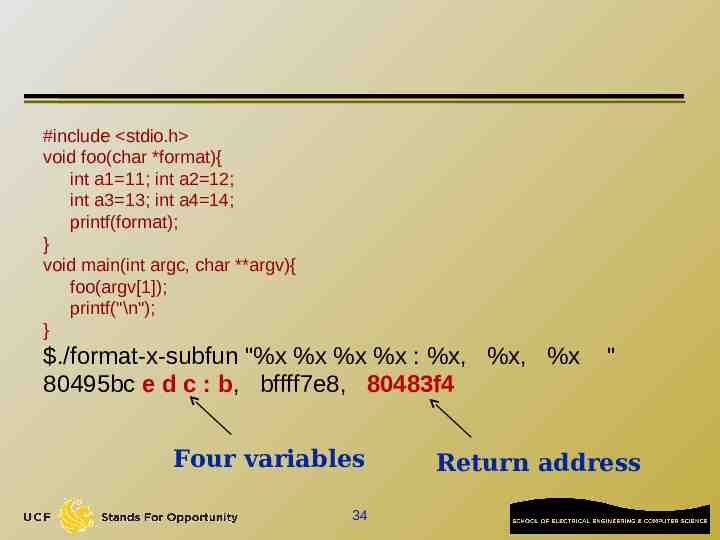
#include stdio.h void foo(char *format){ int a1 11; int a2 12; int a3 13; int a4 14; printf(format); } void main(int argc, char **argv){ foo(argv[1]); printf("\n"); } ./format-x-subfun "%x %x %x %x : %x, %x, %x 80495bc e d c : b, bffff7e8, 80483f4 Four variables 34 " Return address
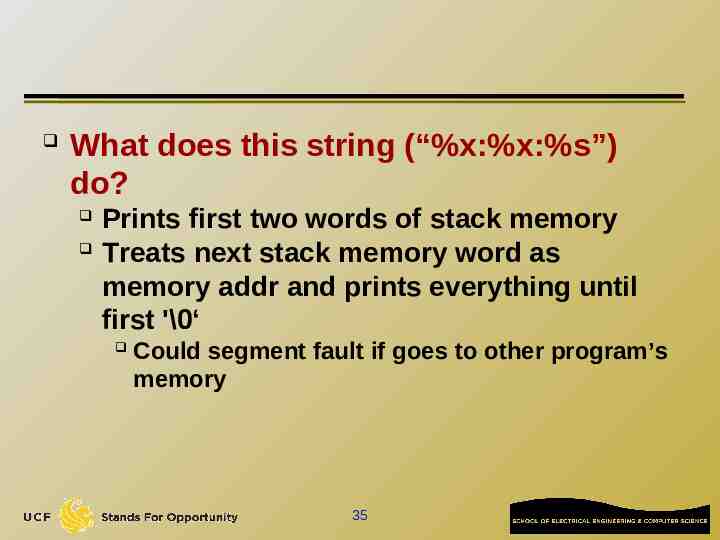
What does this string (“%x:%x:%s”) do? Prints first two words of stack memory Treats next stack memory word as memory addr and prints everything until first '\0‘ Could segment fault if goes to other program’s memory 35
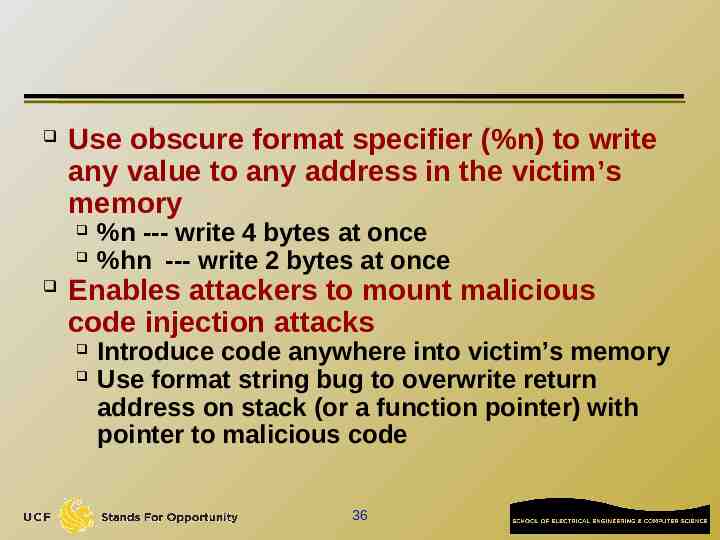
Use obscure format specifier (%n) to write any value to any address in the victim’s memory %n --- write 4 bytes at once %hn --- write 2 bytes at once Enables attackers to mount malicious code injection attacks Introduce code anywhere into victim’s memory Use format string bug to overwrite return address on stack (or a function pointer) with pointer to malicious code 36
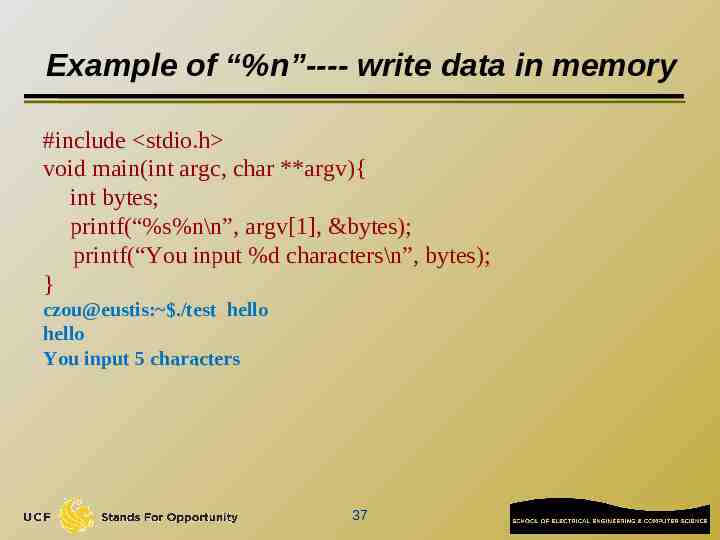
Example of “%n”---- write data in memory #include stdio.h void main(int argc, char **argv){ int bytes; printf(“%s%n\n”, argv[1], &bytes); printf(“You input %d characters\n”, bytes); } czou@eustis: ./test hello hello You input 5 characters 37
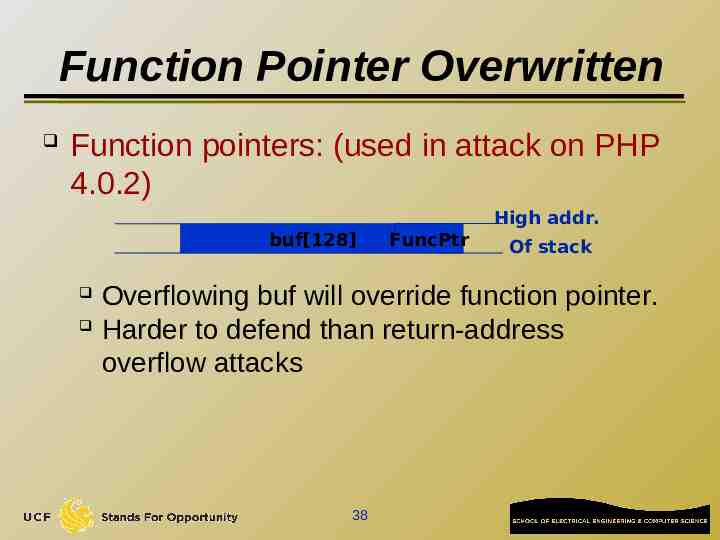
Function Pointer Overwritten Function pointers: (used in attack on PHP 4.0.2) High addr. buf[128] FuncPtr Of stack Overflowing buf will override function pointer. Harder to defend than return-address overflow attacks 38
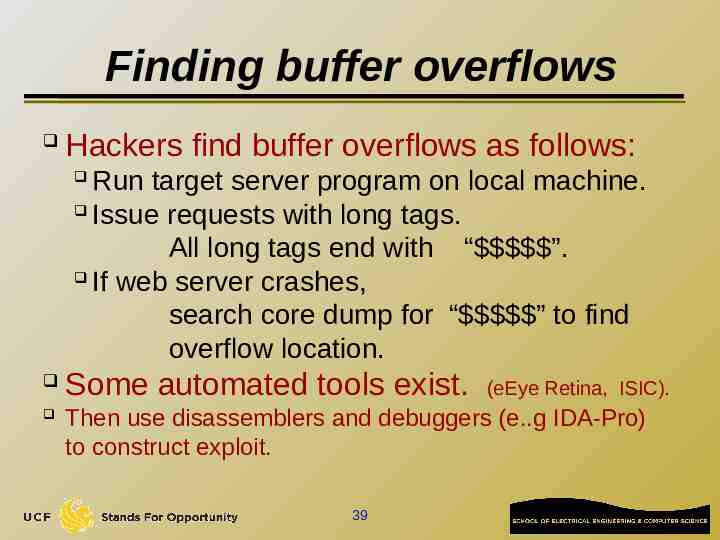
Finding buffer overflows Hackers find buffer overflows as follows: Run target server program on local machine. Issue requests with long tags. All long tags end with “ ”. If web server crashes, search core dump for “ ” to find overflow location. Some automated tools exist. (eEye Retina, ISIC). Then use disassemblers and debuggers (e.g IDA-Pro) to construct exploit. 39
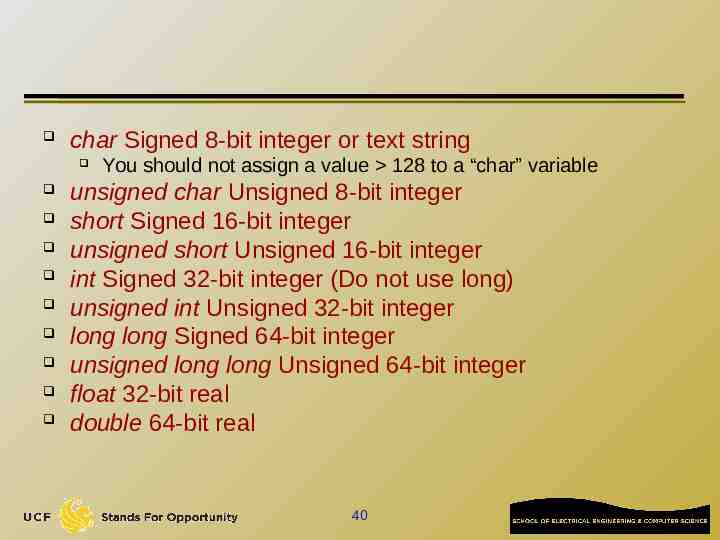
char Signed 8-bit integer or text string You should not assign a value 128 to a “char” variable unsigned char Unsigned 8-bit integer short Signed 16-bit integer unsigned short Unsigned 16-bit integer int Signed 32-bit integer (Do not use long) unsigned int Unsigned 32-bit integer long long Signed 64-bit integer unsigned long long Unsigned 64-bit integer float 32-bit real double 64-bit real 40
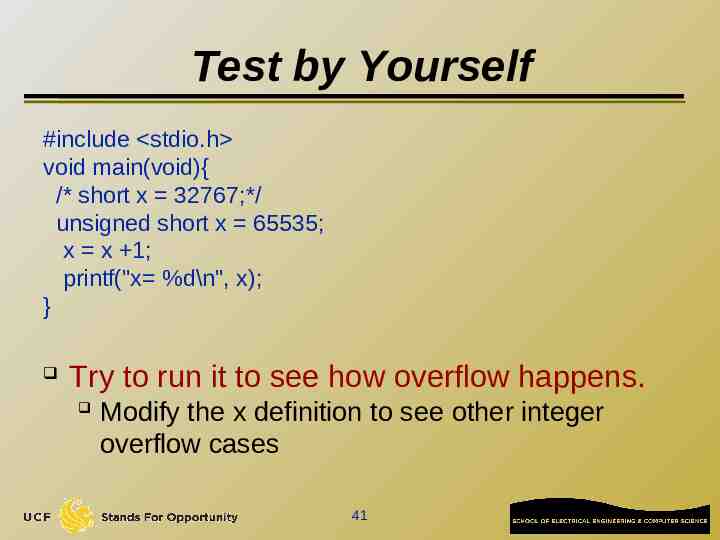
Test by Yourself #include stdio.h void main(void){ /* short x 32767;*/ unsigned short x 65535; x x 1; printf("x %d\n", x); } Try to run it to see how overflow happens. Modify the x definition to see other integer overflow cases 41