EE 319K Introduction to Embedded Systems Lecture 5:Subroutines and
23 Slides647.00 KB
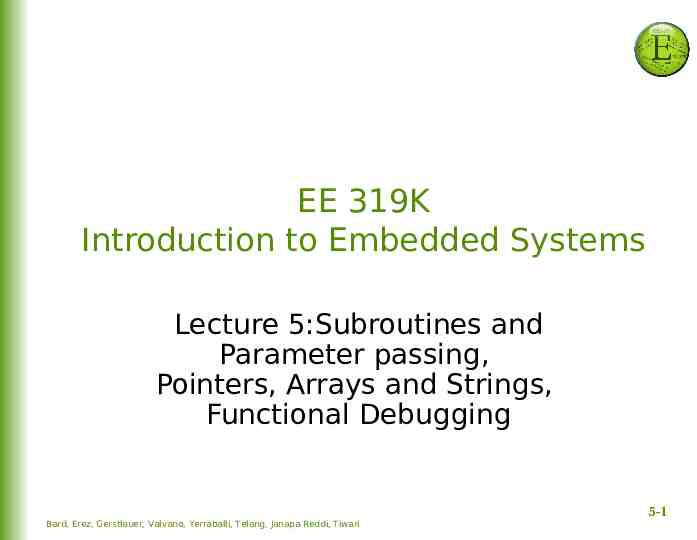
EE 319K Introduction to Embedded Systems Lecture 5:Subroutines and Parameter passing, Pointers, Arrays and Strings, Functional Debugging 5-1 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Agenda Recap Branches and Arithmetic overflow o Signed (V) vs. Unsigned (C) Control Structures o if, if-else, while, do-while, for Signed: B{LT,GE,GT,LE}; LDRSB, LDRSH Unsigned: B{LO,HS,HI,LS}; LDRB, LDRH Outline Subroutines and Parameter Passing Indexed Addressing and Pointers Data Structures: Arrays, Strings Functional Debugging 5-2 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Registers to pass parameters High level program 1) Sets Registers to contain inputs 2) Calls subroutine 6) Registers contain outputs Subroutine 3) Sees the inputs in registers 4) Performs the action of the subroutine 5) Places the outputs in registers 5-3 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Stack to pass parameters High level program 1) Pushes inputs on the Stack 2) Calls subroutine 6) Stack contain outputs (pop) 7) Balance stack Subroutine 3) Sees the inputs on stack (pops) 4) Performs the action of the subroutine 5) Pushes outputs on the stack 5-4 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Assembly Parameter Passing Parameters passed using registers/stack Parameter passing need not be done using only registers or only stack Some inputs could come in registers and some on stack and outputs could be returned in registers and some on the stack Calling Convention Application Binary Interface (ABI) ARM: use registers for first 4 parameters, use stack beyond, return using R0 Keep in mind that you may want your assembly subroutine to someday be callable from C or callable from someone else’s software 5-5 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

ARM Arch. Procedure Call Standard (AAPCS) ABI standard for all ARM architectures Use registers R0, R1, R2, and R3 to pass the first four input parameters (in order) into any function, C or assembly. We place the return parameter in Register R0. Functions can freely modify registers R0–R3 and R12. If a function needs to use R4 through R11, it is necessary to push their current register values onto the stack, use the register, and then pop the old value off the stack before returning. 5-6 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Parameter-Passing: Registers Caller ;--call a subroutine that ;uses registers for parameter passing MOV R0,#7 MOV R1,#3 BL Exp ;; R2 becomes 7 3 343 (0x157) Call by value Suggest changes to make it AAPCS Callee ;---------Exp----------; Input: R0 and R1 have inputs XX an YY ; Output: R2 has the result XX raised to YY ; Comments: R1 and R2 and non-negative ; Destroys input R1 XX RN 0 YY RN 1 Pow RN 2 Exp ADDS XX,#0 BEQ Zero ADDS YY,#0 ; check if YY is zero BEQ One MOV pow, #1 ; Initial product is 1 More MUL pow,XX ; multiply product with XX SUBS YY,#1 ; Decrement YY BNE More B Retn ; Done, so return Zero MOV pow,#0 ; XX is 0 so result is 0 B Retn One MOV pow,#1 ; YY is 0 so result is 1 Retn BX LR Return by value 5-7 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Parameter-Passing: Stack Caller ;-------- call a subroutine that ; uses stack for parameter passing MOV R0,#12 MOV R1,#5 MOV R2,#22 MOV R3,#7 MOV R4,#18 PUSH {R0-R4} ; Stack has 12,5,22,7 and 18 ; (with 12 on top) BL Max5 ; Call Max5 to find the maximum ;of the five numbers POP {R5} ;; R5 has the max element (22) Callee ;---------Max5----------; Input: 5 signed numbers pushed on the stack ; Output: put only the maximum number on the stack ; Comments: The input numbers are removed from stack numM RN 1 ; current number max RN 2 ; maximum so far count RN 0 ; how many elements Max5 POP {max} ; get top element (top of stack) ; store it in max MOV count,#4 ; 4 more to go Again POP {numM} ; get next element CMP numM,max BLT Next MOV max, numM ; new numM is the max Next SUBS count,#1 ; one more checked BNE Again PUSH {max} ; found max so push it on stack BX LR Call by value Return by value Flexible style, but not AAPCS 5-8 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Parameter-Passing: Stack & Regs Caller ;------call a subroutine that uses ;both stack and registers for ;parameter passing MOV R0,#6 ; R0 elem count MOV R1,#-14 MOV R2,#5 MOV R3,#32 MOV R4,#-7 MOV R5,#0 MOV R6,#-5 PUSH {R4-R6} ; rest on stack ; R0 has element count ; R1-R3 have first 3 elements; ; remaining on Stack BL MinMax ;; R0 has -14 and R1 has 32 ;; upon return Not AAPCS Callee ;---------MinMax----------; Input: N numbers reg stack; N passed in R0 ; Output: Return in R0 the min and R1 the max ; Comments: The input numbers are removed from stack numMM RN 3; hold the current number in numMM max RN 1 ; hold maximum so far in max min RN 2 N RN 0 ; how many elements MinMax PUSH {R1-R3} ; put all elements on stack CMP N,#0 ; if N is zero nothing to do BEQ DoneMM POP {min} ; pop top and set it MOV max,min ; as the current min and max loop SUBS N,#1 ; decrement and check BEQ DoneMM POP {numMM} CMP numMM,max BLT Chkmin MOV max,numMM ; new num is the max Chkmin CMP numMM,min BGT NextMM MOV min,numMM ; new num is the min NextMM B loop DoneMM MOV R0,min ; R0 has min 5-9 BX LR

Pointers Pointers are addresses that refer to objects Objects may be data, functions or other pointers If a register or memory location contains an address we say it points into memory Not pointing to anything Pt Object1 Pointing to Object1 Pt Object2 Pointing to Object2 Object1 Pt Object2 Object1 Object2 Use of indexed addressing mode First, setup pointer Memory Second, dereference pointer LDR R1,[R0] R0 R1 5-10

Data Structures Pointers are the basis for data structures Array or string Stack Pt Linked list FIFO queue Pt GetPt SP Data structure PutPt { Functions to facilitate access Organization of data 5-11 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Arrays Random access Sequential access An array Array or string Pt } Length o equal precision and o allows random access. Precision The precision is the size of each element. (n) The length is the number of elements (fixed or variable). The origin is the index of the first element. zero-origin indexing. Data in consecutive memory int32 t Buffer[100] {Access: Buffer[i] 5-12 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Array Declaration In assembly A Prime AREA Data SPACE 400 AREA .text DCW 1,2,3,5,7,11,13 In C uint32 t A[100]; const uint16 t Prime[] {1,2,3,5,7,11,13}; Split declaration on multiple lines if needed Length of the array? One simple mechanism saves the length of the array as the first element In C: const int8 t Data[5] {4,0x05,0x06,0x0A,0x09}; const int16 t Powers[6] {5,1,10,100,1000,10000}; const int32 t Filter[5] {4,0xAABBCCDD,0x00,0xFFFFFFFF,-0x01} In assembly: Data DCB 4,0x05,0x06,0x0A,0x09 Powers DCW 5,1,10,100,1000,10000 Filter DCD 4,0xAABBCCDD, 0x00 DCD 0xFFFFFFFF,-0x01 5-13 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Arrays Length of the Array: Buffer4 R0 -1 4 -3 1000 -4 String2 -400 'Y' -1 'e' -32768 Alternative mechanism stores a special termination code as the last element. R0 ASCII NUL ETX EOT FF CR ETB C \0 \x03 \x04 \f \r \x17 code 0x00 0x03 0x04 0x0C 0x0D 0x17 name null end of text end of transmission form feed carriage return end of transmission block 'r' 'r' 'a' 'b' 'a' 'l' 'l' 'i' ' ' 'i' 's' '?' 0 7 elements 16-bit R0 Lab9 STX '1' '.' '2' '3' '4' CR ETX 8-bit 8-bit 5-14 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Array Access In general, let n be the precision of a zero-origin indexed array in elements. n 1 (8-bit elements) n 2 (16-bit elements) n 4 (32-bit elements) If I is the index and Base is the base address of the array, then the address of the element at I is In C d Prime[4]; In assembly LDR R0, Prime LDRH R1,[R0,#8] Base n*I 5-15 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Array Access Indexed access In C In C uint32 t i; sum 0; for(i 0; i 7; i ) { sum Prime[i]; } In assembly Pointer access uint16 t uint16 t *p; sum 0; for(p Prime; p ! &Prime[7]; p ){ sum *p; } In assembly LDR R0, Prime LDR R0, Prime MOV R1,#0 ; ofs 0 ADD R1,R0,#14 MOV R3,#0 ; sum 0 MOV R3,#0 lp LDRH R2,[R0,R1] ;Prime[i] lp LDRH R2,[R0] ADD R3,R3,R2 ; sum ADD R3,R3,R2 ADD R1,R1,#2 ; ofs 2 ADD R0,R0,#2 CMP R1,#14 ; ofs 14? CMP R0,R1 BLO lp BNE lp Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari ; p Prime ; &Prime[7] ; sum 0 ; *p ; sum . ; p 5-16

Example 6.2 Statement: Design an exponential function, y 10x, with a 32-bit output. Solution: Since the output is less than 4,294,967,295, the input must be between 0 and 9. One simple solution is to employ a constant word array, as shown below. Each element is 32 bits. In assembly, we define a word constant using DCD, making sure in exists in ROM. 0x00000134 0x00000138 0x0000013C 0x00000140 0x00000144 0x00000148 0x0000014C 0x00000150 0x00000154 0x00000158 1 10 100 1,000 10,000 100,000 1,000,000 10,000,000 100,000,000 1,000,000,000 const uint32 t Powers[10] {1,10,100,1000,10000,100000,1000000,10000000,100000000,1000000000}; 5-17 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Solution C Assembly const uint32 t Powers[10] {1,10,100,1000,10000, 100000,1000000,10000000, 100000000,1000000000}; AREA .text ,CODE,READONLY,ALIGN 2 Powers DCD 1, 10, 100, 1000, 10000 DCD 100000, 1000000, 10000000 DCD 100000000, 1000000000 ;------power---; Input: R0 x ; Output: R0 10 x power LSL R0, R0, #2 ; x x*4 LDR R1, Powers ; R1 &Powers LDR R0, [R1, R0] ; y Powers[x] BX LR uint32 t power(uint32 t x){ return Powers[x]; } Powers[0] Powers[1] Powers[2] Powers[3] Powers[4] Powers[5] Powers[6] Powers[7] Powers[8] Powers[9] 1 10 100 1,000 10,000 100,000 1,000,000 10,000,000 100,000,000 1,000,000,000 Base Offset 32-bit 5-18 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Strings Problem: Write software to output an ASCII string to an output device. Solution: Because the length of the string may be too long to place all the ASCII characters into the registers at the same time, call by reference parameter passing will be used. With call by reference, a pointer to the string will be passed. The function OutString, will output the string data to the output device. The function OutChar will be developed later. For now all we need to know is that it outputs a single ASCII character to the serial port. 5-19 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Solution Hello DCB "Hello world\n\r",0 const uint8 t Hello[] "Hello world\n\r"; ;Input: R0 points to string UART OutString PUSH {R4, LR} MOV R4, R0 void UART OutString(uint8 t *pt){ while(*pt){ UART OutChar(*pt); pt ; } } void main(void){ UART Init(); UART OutString("Hello World"); while(1){}; } loop LDRB R0, [R4] CMP R0, #0 ;done? BEQ BL ADD B done POP done ;0 sentinel UART OutChar ;print R4, #1 ;next loop {R4, PC} Start LDR R0, Hello BL UART OutString Call by reference Think about how this is insecure. What might happen if a malicious program called this? 5-20 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Functional Debugging Instrumentation: dump into array without filtering Assume PortA and PortB have strategic 8-bit information. SIZE ABuf BBuf Cnt EQU SPACE SPACE SPACE 20 SIZE SIZE 4 #define SIZE 20 uint8 t ABuf[SIZE]; uint8 t BBuf[SIZE]; uint32 t Cnt; Cnt will be used to index into the buffers Cnt must be set to index the first element, before the debugging begins. LDR R0, Cnt MOV R1,#0 STR R1,[R0] Cnt 0; 5-21 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Functional Debugging The debugging instrument saves the strategic Port information into respective Buffers Save PUSH {R0-R4,LR} LDR R0, Cnt ;R0 &Cnt LDR R1,[R0] ;R1 Cnt CMP R1,#SIZE BHS done ;full? LDR R3, GPIO PORTA DATA R LDRB R3,[R3] ;R3 is PortA LDR R2, ABuf STRB R3,[R2,R1] ;save PortA LDR R3, GPIO PORTB DATA R LDRB R3,[R3] ;R3 is PortB LDR R2, BBuf STRB R3,[R2,R1] ;save PortB ADD R1,#1 STR R1,[R0] ;save Cnt done POP {R0-R4,PC} void Save(void){ if(Cnt SIZE){ ABuf[Cnt] GPIO PORTA DATA R; BBuf[Cnt] GPIO PORTB DATA R; Cnt ; } } Debugging instruments save/restore all registers Next, you add BL Save statements at strategic places within the system. Use the debugger to display the results after program is done 5-22 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari

Lab 4 preparation 0) Watch Video 9.1 in ebook chapter 9 http://users.ece.utexas.edu/ valvano/Volume1/E-Bo ok/C9 ArrayFunctionalDebugging.htm 1) Read and watch section 9.5 in the ebook http://users.ece.utexas.edu/ valvano/Volume1/E-Bo ok/C9 ArrayFunctionalDebugging.htm 2) Watch Videos 10.1 and 10.2. in ebook chapter 10 http://users.ece.utexas.edu/ valvano/Volume1/E-Bo ok/C10 FiniteStateMachines.htm 3) Read textbook sections 4.3 and 4.4, you will use assembly code from book Program 4.7 5-23 Bard, Erez, Gerstlauer, Valvano, Yerraballi, Telang, Janapa Reddi, Tiwari